
First release
Dependencies: EthernetInterface HTTPServer TextLCD mbed-rpc mbed-rtos mbed
ov7670.h
00001 // This code was written by Mr.Sadaei Osakabe. 00002 // Original code is located at 00003 // https://mbed.org/users/diasea/code/OV7670_with_AL422B_Color_Size_test/ 00004 00005 #include "mbed.h" 00006 #include "ov7670reg.h" 00007 00008 #define OV7670_WRITE (0x42) 00009 #define OV7670_READ (0x43) 00010 #define OV7670_WRITEWAIT (20) 00011 #define OV7670_NOACK (0) 00012 #define OV7670_REGMAX (201) 00013 #define OV7670_I2CFREQ (50000) 00014 00015 // 00016 // OV7670 + FIFO AL422B camera board test 00017 // 00018 class OV7670 00019 { 00020 public: 00021 I2C camera; 00022 InterruptIn vsync,href; 00023 DigitalOut wen; 00024 BusIn data; 00025 DigitalOut rrst,oe,rclk; 00026 volatile int LineCounter; 00027 volatile int LastLines; 00028 volatile bool CaptureReq; 00029 volatile bool Busy; 00030 volatile bool Done; 00031 00032 OV7670( 00033 PinName sda,// Camera I2C port 00034 PinName scl,// Camera I2C port 00035 PinName vs, // VSYNC 00036 PinName hr, // HREF 00037 PinName we, // WEN 00038 PinName d7, // D7 00039 PinName d6, // D6 00040 PinName d5, // D5 00041 PinName d4, // D4 00042 PinName d3, // D3 00043 PinName d2, // D2 00044 PinName d1, // D1 00045 PinName d0, // D0 00046 PinName rt, // /RRST 00047 PinName o, // /OE 00048 PinName rc // RCLK 00049 ) : camera(sda,scl),vsync(vs),href(hr),wen(we),data(d0,d1,d2,d3,d4,d5,d6,d7),rrst(rt),oe(o),rclk(rc) 00050 { 00051 camera.stop(); 00052 camera.frequency(OV7670_I2CFREQ); 00053 vsync.fall(this,&OV7670::VsyncHandler); 00054 href.rise(this,&OV7670::HrefHandler); 00055 CaptureReq = false; 00056 Busy = false; 00057 Done = false; 00058 LineCounter = 0; 00059 rrst = 1; 00060 oe = 1; 00061 rclk = 1; 00062 wen = 0; 00063 } 00064 00065 // capture request 00066 void CaptureNext(void) 00067 { 00068 CaptureReq = true; 00069 Busy = true; 00070 } 00071 00072 // capture done? (with clear) 00073 bool CaptureDone(void) 00074 { 00075 bool result; 00076 if (Busy) { 00077 result = false; 00078 } else { 00079 result = Done; 00080 Done = false; 00081 } 00082 return result; 00083 } 00084 00085 // write to camera 00086 void WriteReg(int addr,int data) 00087 { 00088 // WRITE 0x42,ADDR,DATA 00089 camera.start(); 00090 camera.write(OV7670_WRITE); 00091 wait_us(OV7670_WRITEWAIT); 00092 camera.write(addr); 00093 wait_us(OV7670_WRITEWAIT); 00094 camera.write(data); 00095 camera.stop(); 00096 } 00097 00098 // read from camera 00099 int ReadReg(int addr) 00100 { 00101 int data; 00102 00103 // WRITE 0x42,ADDR 00104 camera.start(); 00105 camera.write(OV7670_WRITE); 00106 wait_us(OV7670_WRITEWAIT); 00107 camera.write(addr); 00108 camera.stop(); 00109 wait_us(OV7670_WRITEWAIT); 00110 00111 // WRITE 0x43,READ 00112 camera.start(); 00113 camera.write(OV7670_READ); 00114 wait_us(OV7670_WRITEWAIT); 00115 data = camera.read(OV7670_NOACK); 00116 camera.stop(); 00117 00118 return data; 00119 } 00120 00121 // print register 00122 void PrintRegister(void) { 00123 printf("AD : +0 +1 +2 +3 +4 +5 +6 +7 +8 +9 +A +B +C +D +E +F"); 00124 for (int i=0;i<OV7670_REGMAX;i++) { 00125 int data; 00126 data = ReadReg(i); // READ REG 00127 if ((i & 0x0F) == 0) { 00128 printf("\r\n%02X : ",i); 00129 } 00130 printf("%02X ",data); 00131 } 00132 printf("\r\n"); 00133 } 00134 00135 void Reset(void) { 00136 WriteReg(REG_COM7,COM7_RESET); // RESET CAMERA 00137 wait_ms(200); 00138 } 00139 00140 void InitForFIFOWriteReset(void) { 00141 WriteReg(REG_COM10, COM10_VS_NEG); 00142 } 00143 00144 void InitSetColorbar(void) { 00145 int reg_com7 = ReadReg(REG_COM7); 00146 // color bar 00147 WriteReg(REG_COM17, reg_com7|COM17_CBAR); 00148 } 00149 00150 void InitDefaultReg(void) { 00151 // Gamma curve values 00152 WriteReg(0x7a, 0x20); 00153 WriteReg(0x7b, 0x10); 00154 WriteReg(0x7c, 0x1e); 00155 WriteReg(0x7d, 0x35); 00156 WriteReg(0x7e, 0x5a); 00157 WriteReg(0x7f, 0x69); 00158 WriteReg(0x80, 0x76); 00159 WriteReg(0x81, 0x80); 00160 WriteReg(0x82, 0x88); 00161 WriteReg(0x83, 0x8f); 00162 WriteReg(0x84, 0x96); 00163 WriteReg(0x85, 0xa3); 00164 WriteReg(0x86, 0xaf); 00165 WriteReg(0x87, 0xc4); 00166 WriteReg(0x88, 0xd7); 00167 WriteReg(0x89, 0xe8); 00168 00169 // AGC and AEC parameters. Note we start by disabling those features, 00170 //then turn them only after tweaking the values. 00171 WriteReg(REG_COM8, COM8_FASTAEC | COM8_AECSTEP | COM8_BFILT); 00172 WriteReg(REG_GAIN, 0); 00173 WriteReg(REG_AECH, 0); 00174 WriteReg(REG_COM4, 0x40); 00175 // magic reserved bit 00176 WriteReg(REG_COM9, 0x18); 00177 // 4x gain + magic rsvd bit 00178 WriteReg(REG_BD50MAX, 0x05); 00179 WriteReg(REG_BD60MAX, 0x07); 00180 WriteReg(REG_AEW, 0x95); 00181 WriteReg(REG_AEB, 0x33); 00182 WriteReg(REG_VPT, 0xe3); 00183 WriteReg(REG_HAECC1, 0x78); 00184 WriteReg(REG_HAECC2, 0x68); 00185 WriteReg(0xa1, 0x03); 00186 // magic 00187 WriteReg(REG_HAECC3, 0xd8); 00188 WriteReg(REG_HAECC4, 0xd8); 00189 WriteReg(REG_HAECC5, 0xf0); 00190 WriteReg(REG_HAECC6, 0x90); 00191 WriteReg(REG_HAECC7, 0x94); 00192 WriteReg(REG_COM8, COM8_FASTAEC|COM8_AECSTEP|COM8_BFILT|COM8_AGC|COM8_AEC); 00193 00194 // Almost all of these are magic "reserved" values. 00195 WriteReg(REG_COM5, 0x61); 00196 WriteReg(REG_COM6, 0x4b); 00197 WriteReg(0x16, 0x02); 00198 WriteReg(REG_MVFP, 0x07); 00199 WriteReg(0x21, 0x02); 00200 WriteReg(0x22, 0x91); 00201 WriteReg(0x29, 0x07); 00202 WriteReg(0x33, 0x0b); 00203 WriteReg(0x35, 0x0b); 00204 WriteReg(0x37, 0x1d); 00205 WriteReg(0x38, 0x71); 00206 WriteReg(0x39, 0x2a); 00207 WriteReg(REG_COM12, 0x78); 00208 WriteReg(0x4d, 0x40); 00209 WriteReg(0x4e, 0x20); 00210 WriteReg(REG_GFIX, 0); 00211 WriteReg(0x6b, 0x0a); 00212 WriteReg(0x74, 0x10); 00213 WriteReg(0x8d, 0x4f); 00214 WriteReg(0x8e, 0); 00215 WriteReg(0x8f, 0); 00216 WriteReg(0x90, 0); 00217 WriteReg(0x91, 0); 00218 WriteReg(0x96, 0); 00219 WriteReg(0x9a, 0); 00220 WriteReg(0xb0, 0x84); 00221 WriteReg(0xb1, 0x0c); 00222 WriteReg(0xb2, 0x0e); 00223 WriteReg(0xb3, 0x82); 00224 WriteReg(0xb8, 0x0a); 00225 00226 // More reserved magic, some of which tweaks white balance 00227 WriteReg(0x43, 0x0a); 00228 WriteReg(0x44, 0xf0); 00229 WriteReg(0x45, 0x34); 00230 WriteReg(0x46, 0x58); 00231 WriteReg(0x47, 0x28); 00232 WriteReg(0x48, 0x3a); 00233 WriteReg(0x59, 0x88); 00234 WriteReg(0x5a, 0x88); 00235 WriteReg(0x5b, 0x44); 00236 WriteReg(0x5c, 0x67); 00237 WriteReg(0x5d, 0x49); 00238 WriteReg(0x5e, 0x0e); 00239 WriteReg(0x6c, 0x0a); 00240 WriteReg(0x6d, 0x55); 00241 WriteReg(0x6e, 0x11); 00242 WriteReg(0x6f, 0x9f); 00243 // "9e for advance AWB" 00244 WriteReg(0x6a, 0x40); 00245 WriteReg(REG_BLUE, 0x40); 00246 WriteReg(REG_RED, 0x60); 00247 WriteReg(REG_COM8, COM8_FASTAEC|COM8_AECSTEP|COM8_BFILT|COM8_AGC|COM8_AEC|COM8_AWB); 00248 00249 // Matrix coefficients 00250 WriteReg(0x4f, 0x80); 00251 WriteReg(0x50, 0x80); 00252 WriteReg(0x51, 0); 00253 WriteReg(0x52, 0x22); 00254 WriteReg(0x53, 0x5e); 00255 WriteReg(0x54, 0x80); 00256 WriteReg(0x58, 0x9e); 00257 00258 WriteReg(REG_COM16, COM16_AWBGAIN); 00259 WriteReg(REG_EDGE, 0); 00260 WriteReg(0x75, 0x05); 00261 WriteReg(0x76, 0xe1); 00262 WriteReg(0x4c, 0); 00263 WriteReg(0x77, 0x01); 00264 WriteReg(0x4b, 0x09); 00265 WriteReg(0xc9, 0x60); 00266 WriteReg(REG_COM16, 0x38); 00267 WriteReg(0x56, 0x40); 00268 00269 WriteReg(0x34, 0x11); 00270 WriteReg(REG_COM11, COM11_EXP|COM11_HZAUTO_ON); 00271 WriteReg(0xa4, 0x88); 00272 WriteReg(0x96, 0); 00273 WriteReg(0x97, 0x30); 00274 WriteReg(0x98, 0x20); 00275 WriteReg(0x99, 0x30); 00276 WriteReg(0x9a, 0x84); 00277 WriteReg(0x9b, 0x29); 00278 WriteReg(0x9c, 0x03); 00279 WriteReg(0x9d, 0x4c); 00280 WriteReg(0x9e, 0x3f); 00281 WriteReg(0x78, 0x04); 00282 00283 // Extra-weird stuff. Some sort of multiplexor register 00284 WriteReg(0x79, 0x01); 00285 WriteReg(0xc8, 0xf0); 00286 WriteReg(0x79, 0x0f); 00287 WriteReg(0xc8, 0x00); 00288 WriteReg(0x79, 0x10); 00289 WriteReg(0xc8, 0x7e); 00290 WriteReg(0x79, 0x0a); 00291 WriteReg(0xc8, 0x80); 00292 WriteReg(0x79, 0x0b); 00293 WriteReg(0xc8, 0x01); 00294 WriteReg(0x79, 0x0c); 00295 WriteReg(0xc8, 0x0f); 00296 WriteReg(0x79, 0x0d); 00297 WriteReg(0xc8, 0x20); 00298 WriteReg(0x79, 0x09); 00299 WriteReg(0xc8, 0x80); 00300 WriteReg(0x79, 0x02); 00301 WriteReg(0xc8, 0xc0); 00302 WriteReg(0x79, 0x03); 00303 WriteReg(0xc8, 0x40); 00304 WriteReg(0x79, 0x05); 00305 WriteReg(0xc8, 0x30); 00306 WriteReg(0x79, 0x26); 00307 } 00308 00309 void InitRGB444(void){ 00310 int reg_com7 = ReadReg(REG_COM7); 00311 00312 WriteReg(REG_COM7, reg_com7|COM7_RGB); 00313 WriteReg(REG_RGB444, RGB444_ENABLE|RGB444_XBGR); 00314 WriteReg(REG_COM15, COM15_R01FE|COM15_RGB444); 00315 00316 WriteReg(REG_COM1, 0x40); // Magic reserved bit 00317 WriteReg(REG_COM9, 0x38); // 16x gain ceiling; 0x8 is reserved bit 00318 WriteReg(0x4f, 0xb3); // "matrix coefficient 1" 00319 WriteReg(0x50, 0xb3); // "matrix coefficient 2" 00320 WriteReg(0x51, 0x00); // vb 00321 WriteReg(0x52, 0x3d); // "matrix coefficient 4" 00322 WriteReg(0x53, 0xa7); // "matrix coefficient 5" 00323 WriteReg(0x54, 0xe4); // "matrix coefficient 6" 00324 WriteReg(REG_COM13, COM13_GAMMA|COM13_UVSAT|0x2); // Magic rsvd bit 00325 00326 WriteReg(REG_TSLB, 0x04); 00327 } 00328 00329 void InitRGB555(void){ 00330 int reg_com7 = ReadReg(REG_COM7); 00331 00332 WriteReg(REG_COM7, reg_com7|COM7_RGB); 00333 WriteReg(REG_RGB444, RGB444_DISABLE); 00334 WriteReg(REG_COM15, COM15_RGB555|COM15_R00FF); 00335 00336 WriteReg(REG_TSLB, 0x04); 00337 00338 WriteReg(REG_COM1, 0x00); 00339 WriteReg(REG_COM9, 0x38); // 16x gain ceiling; 0x8 is reserved bit 00340 WriteReg(0x4f, 0xb3); // "matrix coefficient 1" 00341 WriteReg(0x50, 0xb3); // "matrix coefficient 2" 00342 WriteReg(0x51, 0x00); // vb 00343 WriteReg(0x52, 0x3d); // "matrix coefficient 4" 00344 WriteReg(0x53, 0xa7); // "matrix coefficient 5" 00345 WriteReg(0x54, 0xe4); // "matrix coefficient 6" 00346 WriteReg(REG_COM13, COM13_GAMMA|COM13_UVSAT); 00347 } 00348 00349 void InitRGB565(void){ 00350 int reg_com7 = ReadReg(REG_COM7); 00351 00352 WriteReg(REG_COM7, reg_com7|COM7_RGB); 00353 WriteReg(REG_RGB444, RGB444_DISABLE); 00354 WriteReg(REG_COM15, COM15_R00FF|COM15_RGB565); 00355 00356 WriteReg(REG_TSLB, 0x04); 00357 00358 WriteReg(REG_COM1, 0x00); 00359 WriteReg(REG_COM9, 0x38); // 16x gain ceiling; 0x8 is reserved bit 00360 WriteReg(0x4f, 0xb3); // "matrix coefficient 1" 00361 WriteReg(0x50, 0xb3); // "matrix coefficient 2" 00362 WriteReg(0x51, 0x00); // vb 00363 WriteReg(0x52, 0x3d); // "matrix coefficient 4" 00364 WriteReg(0x53, 0xa7); // "matrix coefficient 5" 00365 WriteReg(0x54, 0xe4); // "matrix coefficient 6" 00366 WriteReg(REG_COM13, COM13_GAMMA|COM13_UVSAT); 00367 } 00368 00369 void InitYUV(void){ 00370 int reg_com7 = ReadReg(REG_COM7); 00371 00372 WriteReg(REG_COM7, reg_com7|COM7_YUV); 00373 WriteReg(REG_RGB444, RGB444_DISABLE); 00374 WriteReg(REG_COM15, COM15_R00FF); 00375 00376 WriteReg(REG_TSLB, 0x04); 00377 // WriteReg(REG_TSLB, 0x14); 00378 // WriteReg(REG_MANU, 0x00); 00379 // WriteReg(REG_MANV, 0x00); 00380 00381 WriteReg(REG_COM1, 0x00); 00382 WriteReg(REG_COM9, 0x18); // 4x gain ceiling; 0x8 is reserved bit 00383 WriteReg(0x4f, 0x80); // "matrix coefficient 1" 00384 WriteReg(0x50, 0x80); // "matrix coefficient 2" 00385 WriteReg(0x51, 0x00); // vb 00386 WriteReg(0x52, 0x22); // "matrix coefficient 4" 00387 WriteReg(0x53, 0x5e); // "matrix coefficient 5" 00388 WriteReg(0x54, 0x80); // "matrix coefficient 6" 00389 WriteReg(REG_COM13, COM13_GAMMA|COM13_UVSAT|COM13_UVSWAP); 00390 } 00391 00392 void InitBayerRGB(void){ 00393 int reg_com7 = ReadReg(REG_COM7); 00394 00395 // odd line BGBG... even line GRGR... 00396 WriteReg(REG_COM7, reg_com7|COM7_BAYER); 00397 // odd line GBGB... even line RGRG... 00398 //WriteReg(REG_COM7, reg_com7|COM7_PBAYER); 00399 00400 WriteReg(REG_RGB444, RGB444_DISABLE); 00401 WriteReg(REG_COM15, COM15_R00FF); 00402 00403 WriteReg(REG_COM13, 0x08); /* No gamma, magic rsvd bit */ 00404 WriteReg(REG_COM16, 0x3d); /* Edge enhancement, denoise */ 00405 WriteReg(REG_REG76, 0xe1); /* Pix correction, magic rsvd */ 00406 00407 WriteReg(REG_TSLB, 0x04); 00408 } 00409 00410 void InitVGA(void) { 00411 // VGA 00412 int reg_com7 = ReadReg(REG_COM7); 00413 00414 WriteReg(REG_COM7,reg_com7|COM7_VGA); 00415 00416 WriteReg(REG_HSTART,HSTART_VGA); 00417 WriteReg(REG_HSTOP,HSTOP_VGA); 00418 WriteReg(REG_HREF,HREF_VGA); 00419 WriteReg(REG_VSTART,VSTART_VGA); 00420 WriteReg(REG_VSTOP,VSTOP_VGA); 00421 WriteReg(REG_VREF,VREF_VGA); 00422 WriteReg(REG_COM3, COM3_VGA); 00423 WriteReg(REG_COM14, COM14_VGA); 00424 WriteReg(REG_SCALING_XSC, SCALING_XSC_VGA); 00425 WriteReg(REG_SCALING_YSC, SCALING_YSC_VGA); 00426 WriteReg(REG_SCALING_DCWCTR, SCALING_DCWCTR_VGA); 00427 WriteReg(REG_SCALING_PCLK_DIV, SCALING_PCLK_DIV_VGA); 00428 WriteReg(REG_SCALING_PCLK_DELAY, SCALING_PCLK_DELAY_VGA); 00429 } 00430 00431 void InitFIFO_2bytes_color_nealy_limit_size(void) { 00432 // nealy FIFO limit 544x360 00433 int reg_com7 = ReadReg(REG_COM7); 00434 00435 WriteReg(REG_COM7,reg_com7|COM7_VGA); 00436 00437 WriteReg(REG_HSTART,HSTART_VGA); 00438 WriteReg(REG_HSTOP,HSTOP_VGA); 00439 WriteReg(REG_HREF,HREF_VGA); 00440 WriteReg(REG_VSTART,VSTART_VGA); 00441 WriteReg(REG_VSTOP,VSTOP_VGA); 00442 WriteReg(REG_VREF,VREF_VGA); 00443 WriteReg(REG_COM3, COM3_VGA); 00444 WriteReg(REG_COM14, COM14_VGA); 00445 WriteReg(REG_SCALING_XSC, SCALING_XSC_VGA); 00446 WriteReg(REG_SCALING_YSC, SCALING_YSC_VGA); 00447 WriteReg(REG_SCALING_DCWCTR, SCALING_DCWCTR_VGA); 00448 WriteReg(REG_SCALING_PCLK_DIV, SCALING_PCLK_DIV_VGA); 00449 WriteReg(REG_SCALING_PCLK_DELAY, SCALING_PCLK_DELAY_VGA); 00450 00451 WriteReg(REG_HSTART, 0x17); 00452 WriteReg(REG_HSTOP, 0x5b); 00453 WriteReg(REG_VSTART, 0x12); 00454 WriteReg(REG_VSTOP, 0x6c); 00455 } 00456 00457 void InitVGA_3_4(void) { 00458 // VGA 3/4 -> 480x360 00459 int reg_com7 = ReadReg(REG_COM7); 00460 00461 WriteReg(REG_COM7,reg_com7|COM7_VGA); 00462 00463 WriteReg(REG_HSTART,HSTART_VGA); 00464 WriteReg(REG_HSTOP,HSTOP_VGA); 00465 WriteReg(REG_HREF,HREF_VGA); 00466 WriteReg(REG_VSTART,VSTART_VGA); 00467 WriteReg(REG_VSTOP,VSTOP_VGA); 00468 WriteReg(REG_VREF,VREF_VGA); 00469 WriteReg(REG_COM3, COM3_VGA); 00470 WriteReg(REG_COM14, COM14_VGA); 00471 WriteReg(REG_SCALING_XSC, SCALING_XSC_VGA); 00472 WriteReg(REG_SCALING_YSC, SCALING_YSC_VGA); 00473 WriteReg(REG_SCALING_DCWCTR, SCALING_DCWCTR_VGA); 00474 WriteReg(REG_SCALING_PCLK_DIV, SCALING_PCLK_DIV_VGA); 00475 WriteReg(REG_SCALING_PCLK_DELAY, SCALING_PCLK_DELAY_VGA); 00476 00477 WriteReg(REG_HSTART, 0x1b); 00478 WriteReg(REG_HSTOP, 0x57); 00479 WriteReg(REG_VSTART, 0x12); 00480 WriteReg(REG_VSTOP, 0x6c); 00481 } 00482 00483 void InitQVGA(void) { 00484 // QQVGA 00485 int reg_com7 = ReadReg(REG_COM7); 00486 00487 WriteReg(REG_COM7,reg_com7|COM7_QVGA); 00488 00489 WriteReg(REG_HSTART,HSTART_QVGA); 00490 WriteReg(REG_HSTOP,HSTOP_QVGA); 00491 WriteReg(REG_HREF,HREF_QVGA); 00492 WriteReg(REG_VSTART,VSTART_QVGA); 00493 WriteReg(REG_VSTOP,VSTOP_QVGA); 00494 WriteReg(REG_VREF,VREF_QVGA); 00495 WriteReg(REG_COM3, COM3_QVGA); 00496 WriteReg(REG_COM14, COM14_QVGA); 00497 WriteReg(REG_SCALING_XSC, SCALING_XSC_QVGA); 00498 WriteReg(REG_SCALING_YSC, SCALING_YSC_QVGA); 00499 WriteReg(REG_SCALING_DCWCTR, SCALING_DCWCTR_QVGA); 00500 WriteReg(REG_SCALING_PCLK_DIV, SCALING_PCLK_DIV_QVGA); 00501 WriteReg(REG_SCALING_PCLK_DELAY, SCALING_PCLK_DELAY_QVGA); 00502 } 00503 00504 void InitQQVGA(void) { 00505 // QQVGA 00506 int reg_com7 = ReadReg(REG_COM7); 00507 00508 WriteReg(REG_COM7,reg_com7|COM7_QQVGA); 00509 00510 WriteReg(REG_HSTART,HSTART_QQVGA); 00511 WriteReg(REG_HSTOP,HSTOP_QQVGA); 00512 WriteReg(REG_HREF,HREF_QQVGA); 00513 WriteReg(REG_VSTART,VSTART_QQVGA); 00514 WriteReg(REG_VSTOP,VSTOP_QQVGA); 00515 WriteReg(REG_VREF,VREF_QQVGA); 00516 WriteReg(REG_COM3, COM3_QQVGA); 00517 WriteReg(REG_COM14, COM14_QQVGA); 00518 WriteReg(REG_SCALING_XSC, SCALING_XSC_QQVGA); 00519 WriteReg(REG_SCALING_YSC, SCALING_YSC_QQVGA); 00520 WriteReg(REG_SCALING_DCWCTR, SCALING_DCWCTR_QQVGA); 00521 WriteReg(REG_SCALING_PCLK_DIV, SCALING_PCLK_DIV_QQVGA); 00522 WriteReg(REG_SCALING_PCLK_DELAY, SCALING_PCLK_DELAY_QQVGA); 00523 } 00524 00525 // vsync handler 00526 void VsyncHandler(void) 00527 { 00528 // Capture Enable 00529 if (CaptureReq) { 00530 wen = 1; 00531 Done = false; 00532 CaptureReq = false; 00533 } else { 00534 wen = 0; 00535 if (Busy) { 00536 Busy = false; 00537 Done = true; 00538 } 00539 } 00540 00541 // Hline Counter 00542 LastLines = LineCounter; 00543 LineCounter = 0; 00544 } 00545 00546 // href handler 00547 void HrefHandler(void) 00548 { 00549 LineCounter++; 00550 } 00551 00552 // Data Read 00553 int ReadOneByte(void) 00554 { 00555 int result; 00556 rclk = 1; 00557 // wait_us(1); 00558 result = data; 00559 rclk = 0; 00560 return result; 00561 } 00562 00563 // Data Start 00564 void ReadStart(void) 00565 { 00566 rrst = 0; 00567 oe = 0; 00568 wait_us(1); 00569 rclk = 0; 00570 wait_us(1); 00571 rclk = 1; 00572 wait_us(1); 00573 rrst = 1; 00574 } 00575 00576 // Data Stop 00577 void ReadStop(void) 00578 { 00579 oe = 1; 00580 ReadOneByte(); 00581 rclk = 1; 00582 } 00583 }; 00584
Generated on Tue Jul 12 2022 21:22:57 by
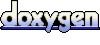