USB Joystick library, modified to work as a 6 DOF joystick.
Fork of USBJoystick by
USBJoystick.cpp
00001 /* mbed USBJoystick Library 00002 * Copyright (c) 2012, v01: Initial version, WH, 00003 * Modified USBMouse code ARM Limited. 00004 * (c) 2010-2011 mbed.org, MIT License 00005 * 2016, v02: Updated USBDevice Lib, Added waitForConnect, Updated 32 bits button 00006 * 00007 * Permission is hereby granted, free of charge, to any person obtaining a copy 00008 * of this software and associated documentation files (the "Software"), to deal 00009 * in the Software without restriction, inclumosig without limitation the rights 00010 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00011 * copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in 00015 * all copies or substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 * IMPLIED, INCLUmosiG BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00020 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00022 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00023 * THE SOFTWARE. 00024 */ 00025 00026 00027 #include "stdint.h" 00028 #include "USBJoystick.h" 00029 00030 bool USBJoystick::update(int16_t x, int16_t y, int16_t z, int16_t rx, int16_t ry, int16_t rz, uint32_t buttons, uint8_t hat) { 00031 00032 _x = x; 00033 _y = y; 00034 _z = z; 00035 _rx = rx; 00036 _ry = ry; 00037 _rz = rz; 00038 _buttons = buttons; 00039 _hat = hat; 00040 00041 return update(); 00042 } 00043 00044 bool USBJoystick::update() { 00045 HID_REPORT report; 00046 00047 // Fill the report according to the Joystick Descriptor 00048 report.data[0] = _x & 0xff; 00049 report.data[1] = (_x >> 8) & 0xff; 00050 report.data[2] = _y & 0xff; 00051 report.data[3] = (_y >> 8) & 0xff; 00052 report.data[4] = _z & 0xff; 00053 report.data[5] = (_z >> 8) & 0xff; 00054 report.data[6] = _rx & 0xff; 00055 report.data[7] = (_rx >> 8) & 0xff; 00056 report.data[8] = _ry & 0xff; 00057 report.data[9] = (_ry >> 8) & 0xff; 00058 report.data[10] = _rz & 0xff; 00059 report.data[11] = (_rz >> 8) & 0xff; 00060 00061 #if (BUTTONS4 == 1) 00062 //Hat and 4 Buttons 00063 // report.data[4] = ((_buttons & 0x0f) << 4) | (_hat & 0x0f) ; 00064 // report.length = 5; 00065 00066 00067 //Use 4 bit padding for hat4 or hat8 00068 report.data[12] = (_hat & 0x0f) ; 00069 00070 //Use 4 bit padding for buttons 00071 report.data[13] = (_buttons & 0x0f) ; 00072 report.length = 8; 00073 #endif 00074 00075 #if (BUTTONS8 == 1) 00076 //Hat and first 4 Buttons 00077 // report.data[4] = ((_buttons & 0x0f) << 4) | (_hat & 0x0f) ; 00078 // 00079 //Use bit padding for last 4 Buttons 00080 // report.data[5] = (_buttons & 0xf0) >> 4; 00081 // report.length = 6; 00082 00083 //Use 4 bit padding for hat4 or hat8 00084 report.data[12] = (_hat & 0x0f) ; 00085 00086 //Use 8 bits for buttons 00087 report.data[13] = (_buttons & 0xff) ; 00088 report.length = 8; 00089 #endif 00090 00091 #if (BUTTONS32 == 1) 00092 //Use 4 bit padding for hat4 or hat8 00093 report.data[12] = (_hat & 0x0f) ; 00094 00095 //No bit padding for 32 buttons 00096 report.data[13] = (_buttons >> 0) & 0xff; 00097 report.data[14] = (_buttons >> 8) & 0xff; 00098 report.data[15] = (_buttons >> 16) & 0xff; 00099 report.data[16] = (_buttons >> 24) & 0xff; 00100 report.length = 17; 00101 #endif 00102 00103 return send(&report); 00104 } 00105 00106 bool USBJoystick::move(int16_t x, int16_t y, int16_t z, int16_t rx, int16_t ry, int16_t rz) { 00107 _x = x; 00108 _y = y; 00109 _z = z; 00110 _rx = rx; 00111 _ry = ry; 00112 _rz = rz; 00113 return update(); 00114 } 00115 00116 bool USBJoystick::buttons(uint32_t buttons) { 00117 _buttons = buttons; 00118 return update(); 00119 } 00120 00121 bool USBJoystick::hat(uint8_t hat) { 00122 _hat = hat; 00123 return update(); 00124 } 00125 00126 void USBJoystick::_init() { 00127 _x = 0x0000; 00128 _y = 0x0000; 00129 _z = 0x0000; 00130 _rx = 0x0000; 00131 _ry = 0x0000; 00132 _rz = 0x0000; 00133 _buttons = 0x00000000; 00134 _hat = 0x00; 00135 } 00136 00137 00138 uint8_t * USBJoystick::reportDesc() { 00139 static uint8_t reportDescriptor[] = { 00140 // value in () is the number of bytes. These bytes follow the comma, least significant byte first 00141 // see USBHID_Types.h for more info 00142 USAGE_PAGE(1), 0x01, // Generic Desktop 00143 LOGICAL_MINIMUM(1), 0x00, // Logical_Minimum (0) 00144 USAGE(1), 0x04, // Usage (Joystick) 00145 COLLECTION(1), 0x01, // Application 00146 00147 // 6 Axes of Joystick 00148 USAGE_PAGE(1), 0x01, // Generic Desktop 00149 USAGE(1), 0x01, // Usage (Pointer) 00150 COLLECTION(1), 0x00, // Physical 00151 USAGE(1), 0x30, // X 00152 USAGE(1), 0x31, // Y 00153 USAGE(1), 0x32, // Z 00154 USAGE(1), 0x33, // RX 00155 USAGE(1), 0x34, // RY 00156 USAGE(1), 0x35, // RZ 00157 LOGICAL_MINIMUM(2), 0x00, 0x80, // -32768 (using 2's complement) 00158 LOGICAL_MAXIMUM(2), 0xff, 0x7f, // 32767 (0x7fff, least significant byte first) 00159 REPORT_SIZE(1), 0x10, // REPORT_SIZE describes the number of bits in this element (16, in this case) 00160 REPORT_COUNT(1), 0x06, 00161 INPUT(1), 0x02, // Data, Variable, Absolute 00162 END_COLLECTION(0), 00163 00164 #if (HAT4 == 1) 00165 // 4 Position Hat Switch 00166 USAGE(1), 0x39, // Usage (Hat switch) 00167 LOGICAL_MINIMUM(1), 0x00, // 0 00168 LOGICAL_MAXIMUM(1), 0x03, // 3 00169 PHYSICAL_MINIMUM(1), 0x00, // Physical_Minimum (0) 00170 PHYSICAL_MAXIMUM(2), 0x0E, 0x01, // Physical_Maximum (270) 00171 UNIT(1), 0x14, // Unit (Eng Rot:Angular Pos) 00172 REPORT_SIZE(1), 0x04, 00173 REPORT_COUNT(1), 0x01, 00174 INPUT(1), 0x02, // Data, Variable, Absolute 00175 #endif 00176 #if (HAT8 == 1) 00177 // 8 Position Hat Switch 00178 USAGE(1), 0x39, // Usage (Hat switch) 00179 LOGICAL_MINIMUM(1), 0x00, // 0 00180 LOGICAL_MAXIMUM(1), 0x07, // 7 00181 PHYSICAL_MINIMUM(1), 0x00, // Physical_Minimum (0) 00182 PHYSICAL_MAXIMUM(2), 0x3B, 0x01, // Physical_Maximum (45 deg x (max of)7 = 315) 00183 UNIT(1), 0x14, // Unit (Eng Rot:Angular Pos) 00184 REPORT_SIZE(1), 0x04, 00185 REPORT_COUNT(1), 0x01, 00186 INPUT(1), 0x02, // Data, Variable, Absolute 00187 #endif 00188 00189 // Padding 4 bits 00190 REPORT_SIZE(1), 0x01, 00191 REPORT_COUNT(1), 0x04, 00192 INPUT(1), 0x01, // Constant 00193 00194 00195 #if (BUTTONS4 == 1) 00196 // 4 Buttons 00197 USAGE_PAGE(1), 0x09, // Buttons 00198 USAGE_MINIMUM(1), 0x01, // 1 00199 USAGE_MAXIMUM(1), 0x04, // 4 00200 LOGICAL_MINIMUM(1), 0x00, // 0 00201 LOGICAL_MAXIMUM(1), 0x01, // 1 00202 REPORT_SIZE(1), 0x01, 00203 REPORT_COUNT(1), 0x04, 00204 UNIT_EXPONENT(1), 0x00, // Unit_Exponent (0) 00205 UNIT(1), 0x00, // Unit (None) 00206 INPUT(1), 0x02, // Data, Variable, Absolute 00207 00208 // Padding 4 bits 00209 REPORT_SIZE(1), 0x01, 00210 REPORT_COUNT(1), 0x04, 00211 INPUT(1), 0x01, // Constant 00212 00213 #endif 00214 #if (BUTTONS8 == 1) 00215 // 8 Buttons 00216 USAGE_PAGE(1), 0x09, // Buttons 00217 USAGE_MINIMUM(1), 0x01, // 1 00218 USAGE_MAXIMUM(1), 0x08, // 8 00219 LOGICAL_MINIMUM(1), 0x00, // 0 00220 LOGICAL_MAXIMUM(1), 0x01, // 1 00221 REPORT_SIZE(1), 0x01, 00222 REPORT_COUNT(1), 0x08, 00223 UNIT_EXPONENT(1), 0x00, // Unit_Exponent (0) 00224 UNIT(1), 0x00, // Unit (None) 00225 INPUT(1), 0x02, // Data, Variable, Absolute 00226 #endif 00227 00228 #if (BUTTONS32 == 1) 00229 // 32 Buttons 00230 USAGE_PAGE(1), 0x09, // Buttons 00231 USAGE_MINIMUM(1), 0x01, // 1 00232 USAGE_MAXIMUM(1), 0x20, // 32 00233 LOGICAL_MINIMUM(1), 0x00, // 0 00234 LOGICAL_MAXIMUM(1), 0x01, // 1 00235 REPORT_SIZE(1), 0x01, 00236 REPORT_COUNT(1), 0x20, 00237 UNIT_EXPONENT(1), 0x00, // Unit_Exponent (0) 00238 UNIT(1), 0x00, // Unit (None) 00239 INPUT(1), 0x02, // Data, Variable, Absolute 00240 #endif 00241 00242 END_COLLECTION(0) 00243 }; 00244 00245 reportLength = sizeof(reportDescriptor); 00246 return reportDescriptor; 00247 }
Generated on Mon Jul 18 2022 19:47:44 by
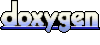