
Interfaced 9 MF51E103F3950 NTC 10k Thermistors to a Nucleo board for a science project.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Using MF51E103F3950 NTC 10k Thermistor, with a B value of 3950 00002 #include "mbed.h" 00003 00004 // which analog pin to connect 00005 #define THERMISTORPIN A0 00006 // resistance at 25 degrees C 00007 #define THERMISTORNOMINAL 10000 00008 // temp. for nominal resistance (almost always 25 C) 00009 #define TEMPERATURENOMINAL 25 00010 // how many samples to take and average, more takes longer 00011 // but is more 'smooth' 00012 #define NUMSAMPLES 5 00013 // The beta coefficient of the thermistor (usually 3000-4000) 00014 #define BCOEFFICIENT 3950 00015 // the value of the 'other' resistor 00016 #define SERIESRESISTOR 10000.0f 00017 00018 #define OFFSET_NUM_AVERAGES 20 00019 00020 Serial pc(USBTX, USBRX); // tx, rx 00021 AnalogIn ain0(A0); 00022 AnalogIn ain1(A1); 00023 AnalogIn ain2(A2); 00024 AnalogIn ain3(A3); 00025 AnalogIn ain4(A4); 00026 AnalogIn ain5(A5); 00027 AnalogIn ain6(PA_5); // Note: on the Nucleo F401, this is connected to the LED. 00028 AnalogIn ain7(PA_6); 00029 AnalogIn ain8(PA_7); 00030 00031 Timer timer; 00032 00033 float offsets[9] = {0}; 00034 float resistors[9] = {9984.270169f, 9999.947582f, 9999.962164f, 9999.960999f, 9999.958757f, 9999.918074f, 9999.969933f, 9999.966487f, 9999.977766f}; 00035 00036 int main() 00037 { 00038 pc.baud(115200); 00039 00040 pc.printf("\nThermistor test\n"); 00041 float rawReading[9] = {0}; 00042 float reading[9] = {0}; 00043 00044 // Find offset values (take average 20 readings for offset): 00045 float sum = 0; 00046 // for(uint8_t i = 0; i < OFFSET_NUM_AVERAGES; i++) { 00047 // sum += ain0.read(); 00048 // } 00049 // offsets[0] = (sum / OFFSET_NUM_AVERAGES); 00050 // 00051 // sum = 0; 00052 // for(uint8_t i = 0; i < OFFSET_NUM_AVERAGES; i++) { 00053 // sum += ain1.read(); 00054 // } 00055 // offsets[1] = (sum / OFFSET_NUM_AVERAGES); 00056 // 00057 // sum = 0; 00058 // for(uint8_t i = 0; i < OFFSET_NUM_AVERAGES; i++) { 00059 // sum += ain2.read(); 00060 // } 00061 // offsets[2] = (sum / OFFSET_NUM_AVERAGES); 00062 // 00063 // sum = 0; 00064 // for(uint8_t i = 0; i < OFFSET_NUM_AVERAGES; i++) { 00065 // sum += ain3.read(); 00066 // } 00067 // offsets[3] = (sum / OFFSET_NUM_AVERAGES); 00068 // 00069 // sum = 0; 00070 // for(uint8_t i = 0; i < OFFSET_NUM_AVERAGES; i++) { 00071 // sum += ain4.read(); 00072 // } 00073 // offsets[4] = (sum / OFFSET_NUM_AVERAGES); 00074 // 00075 // sum = 0; 00076 // for(uint8_t i = 0; i < OFFSET_NUM_AVERAGES; i++) { 00077 // sum += ain5.read(); 00078 // } 00079 // offsets[5] = (sum / OFFSET_NUM_AVERAGES); 00080 // 00081 // sum = 0; 00082 // for(uint8_t i = 0; i < OFFSET_NUM_AVERAGES; i++) { 00083 // sum += ain5.read(); 00084 // } 00085 // offsets[5] = (sum / OFFSET_NUM_AVERAGES); 00086 // 00087 // sum = 0; 00088 // for(uint8_t i = 0; i < OFFSET_NUM_AVERAGES; i++) { 00089 // sum += ain6.read(); 00090 // } 00091 // offsets[6] = (sum / OFFSET_NUM_AVERAGES); 00092 // 00093 // sum = 0; 00094 // for(uint8_t i = 0; i < OFFSET_NUM_AVERAGES; i++) { 00095 // sum += ain7.read(); 00096 // } 00097 // offsets[7] = (sum / OFFSET_NUM_AVERAGES); 00098 // 00099 // sum = 0; 00100 // for(uint8_t i = 0; i < OFFSET_NUM_AVERAGES; i++) { 00101 // sum += ain8.read(); 00102 // } 00103 // offsets[8] = (sum / OFFSET_NUM_AVERAGES); 00104 00105 // Single reading for offset: 00106 // offsets[0] = ain0.read(); 00107 // offsets[1] = ain1.read(); 00108 // offsets[2] = ain2.read(); 00109 // offsets[3] = ain3.read(); 00110 // offsets[4] = ain4.read(); 00111 // offsets[5] = ain5.read(); 00112 // offsets[6] = ain6.read(); 00113 // offsets[7] = ain7.read(); 00114 // offsets[8] = ain8.read(); 00115 00116 // Find average of offsets and store in offsets array: 00117 sum = 0; 00118 float average = 0; 00119 00120 for(uint8_t i = 0; i < 9; i++) { 00121 sum += offsets[i]; 00122 } 00123 00124 average = sum / 9; 00125 00126 for(uint8_t i = 0; i < 9; i++) { 00127 offsets[i] = offsets[i] - average; 00128 } 00129 00130 timer.start(); 00131 00132 while(1) { 00133 // Take reading and apply offset, then use voltage divider equation: 00134 // rawReading[0] = 1 / (ain0.read() - offsets[0]) - 1; 00135 // rawReading[1] = 1 / (ain1.read() - offsets[1]) - 1; 00136 // rawReading[2] = 1 / (ain2.read() - offsets[2]) - 1; 00137 // rawReading[3] = 1 / (ain3.read() - offsets[3]) - 1; 00138 // rawReading[4] = 1 / (ain4.read() - offsets[4]) - 1; 00139 // rawReading[5] = 1 / (ain5.read() - offsets[5]) - 1; 00140 // rawReading[6] = 1 / (ain6.read() - offsets[6]) - 1; 00141 // rawReading[7] = 1 / (ain7.read() - offsets[7]) - 1; 00142 // rawReading[8] = 1 / (ain8.read() - offsets[8]) - 1; 00143 00144 rawReading[0] = 1 / ain0.read() - 1; 00145 rawReading[1] = 1 / ain1.read() - 1; 00146 rawReading[2] = 1 / ain2.read() - 1; 00147 rawReading[3] = 1 / ain3.read() - 1; 00148 rawReading[4] = 1 / ain4.read() - 1; 00149 rawReading[5] = 1 / ain5.read() - 1; 00150 rawReading[6] = 1 / ain6.read() - 1; 00151 rawReading[7] = 1 / ain7.read() - 1; 00152 rawReading[8] = 1 / ain8.read() - 1; 00153 00154 for(uint8_t i = 0; i < 9; i++) { 00155 reading[i] = resistors[i] / rawReading[i]; 00156 } 00157 00158 // pc.printf("Thermistor resistance "); 00159 // pc.printf("%f\t", reading0); 00160 00161 // Apply Steinhart equation: 00162 00163 for(uint8_t i = 0; i < 9; i++) { 00164 float steinhart; 00165 steinhart = reading[i] / THERMISTORNOMINAL; // (R/Ro) 00166 steinhart = log(steinhart); // ln(R/Ro) 00167 steinhart /= BCOEFFICIENT; // 1/B * ln(R/Ro) 00168 steinhart += 1.0 / (TEMPERATURENOMINAL + 273.15); // + (1/To) 00169 steinhart = 1.0f / steinhart; // Invert 00170 steinhart -= 273.15f; // convert to C 00171 // pc.printf("Temperature %d: %f *C\t", i, steinhart); 00172 00173 // Print commas for plotting (except at end): 00174 00175 if(i == 6) 00176 continue; // bad analog input (on LED) 00177 else { 00178 pc.printf("%.4f,", steinhart); 00179 } 00180 } 00181 pc.printf("%.2f\n", timer.read()/60.0f); // print time from boot in minutes 00182 00183 wait(1); 00184 } 00185 }
Generated on Mon Jul 18 2022 13:36:24 by
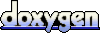