
Simple example to read humidity and temperature from DHT11 sensor using DISCO-L475VG-IOT01A.
Fork of Nucleo_DHT11_Example by
main.cpp
00001 #include "mbed.h" 00002 #include "DHT11.h" 00003 00004 //Tested with success on DISCO-L475VG-IOT01A 00005 //if you find target DISCO-L475VG-IOT01A not found, try to delete mbed library then import it again. 00006 00007 InterruptIn mybutton(USER_BUTTON); 00008 Serial pc(SERIAL_TX, SERIAL_RX); 00009 00010 DHT11 dht(A0); 00011 00012 int main() { 00013 pc.printf("\r\nDHT11 Reader example"); 00014 pc.printf("\r\n===================="); 00015 pc.printf("\r\nPress blue user button to read DHT1\r\n"); 00016 while(1) { 00017 if(mybutton == 0){ 00018 pc.printf("\r\nmybutton pressed!"); 00019 if(dht.readData() < 0){ 00020 pc.printf("\r\nError while reading"); 00021 } 00022 else{ 00023 pc.printf("\r\nReading DHT11 from A0"); 00024 pc.printf("\r\nTemperature: %d ",dht.getTemperature()); 00025 pc.printf("\r\nHumidity: %d \r\n",dht.getHumidity()); 00026 } 00027 00028 } 00029 } 00030 }
Generated on Thu Jul 28 2022 10:54:39 by
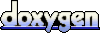