PIR grove sensor that sends the sensed data to Thingspeak.com through the wifi module ESP 8266
Embed:
(wiki syntax)
Show/hide line numbers
ESP8266.h
00001 #ifndef ESP8266_H 00002 #define ESP8266_H 00003 00004 #include <string> 00005 #include "mbed.h" 00006 00007 class ESP8266 00008 { 00009 public: 00010 /** 00011 * ESP8266 constructor 00012 * 00013 * @param tx TX pin 00014 * @param rx RX pin 00015 * @param br Baud Rate 00016 */ 00017 ESP8266(PinName tx, PinName rx, int br); 00018 00019 /** 00020 * ESP8266 destructor 00021 */ 00022 ~ESP8266(); 00023 00024 void SendCMD(char * s); 00025 void Reset(void); 00026 void RcvReply(char * r, int to); 00027 void GetList(char * l); 00028 void Join(char * id, char * pwd); 00029 void GetIP(char * ip); 00030 void SetMode(char mode); 00031 void Quit(void); 00032 void SetSingle(void); 00033 void SetMultiple(void); 00034 void GetConnStatus(char * st); 00035 void StartServerMode(int port); 00036 void CloseServerMode(void); 00037 00038 private: 00039 Serial comm; 00040 void AddEOL(char * s); 00041 void AddChar(char * s, char c); 00042 void itoa(int c, char s[]); 00043 00044 }; 00045 00046 #endif 00047 /* 00048 COMMAND TABLE 00049 Basic: 00050 AT: Just to generate "OK" reply 00051 Wifi: 00052 AT+RST: restart the module 00053 AT+CWMODE: define wifi mode; AT+CWMODE=<mode> 1= Sta, 2= AP, 3=both; Inquiry: AT+CWMODE? or AT+CWMODE=? 00054 AT+CWJAP: join the AP wifi; AT+ CWJAP =<ssid>,< pwd > - ssid = ssid, pwd = wifi password, both between quotes; Inquiry: AT+ CWJAP? 00055 AT+CWLAP: list the AP wifi 00056 AT+CWQAP: quit the AP wifi; Inquiry: AT+CWQAP=? 00057 * AT+CWSAP: set the parameters of AP; AT+CWSAP= <ssid>,<pwd>,<chl>,<ecn> - ssid, pwd, chl = channel, ecn = encryption; Inquiry: AT+CWJAP? 00058 TCP/IP: 00059 AT+CIPSTATUS: get the connection status 00060 * AT+CIPSTART: set up TCP or UDP connection 1)single connection (+CIPMUX=0) AT+CIPSTART= <type>,<addr>,<port>; 2) multiple connection (+CIPMUX=1) AT+CIPSTART= <id><type>,<addr>, <port> - id = 0-4, type = TCP/UDP, addr = IP address, port= port; Inquiry: AT+CIPSTART=? 00061 * AT+CIPSEND: send data; 1)single connection(+CIPMUX=0) AT+CIPSEND=<length>; 2) multiple connection (+CIPMUX=1) AT+CIPSEND= <id>,<length>; Inquiry: AT+CIPSEND=? 00062 * AT+CIPCLOSE: close TCP or UDP connection; AT+CIPCLOSE=<id> or AT+CIPCLOSE; Inquiry: AT+CIPCLOSE=? 00063 AT+CIFSR: Get IP address; Inquiry: AT+ CIFSR=? 00064 AT+CIPMUX: set mutiple connection; AT+ CIPMUX=<mode> - 0 for single connection 1 for mutiple connection; Inquiry: AT+CIPMUX? 00065 AT+CIPSERVER: set as server; AT+ CIPSERVER= <mode>[,<port> ] - mode 0 to close server mode, mode 1 to open; port = port; Inquiry: AT+CIFSR=? 00066 * +IPD: received data 00067 */
Generated on Tue Jul 12 2022 14:11:10 by
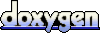