PIR grove sensor that sends the sensed data to Thingspeak.com through the wifi module ESP 8266
Embed:
(wiki syntax)
Show/hide line numbers
ESP8266.cpp
00001 #include "ESP8266.h" 00002 00003 // Constructor 00004 ESP8266::ESP8266(PinName tx, PinName rx, int br) : comm(tx, rx) { 00005 comm.baud(br); 00006 } 00007 00008 // Destructor 00009 ESP8266::~ESP8266() { } 00010 00011 // Add <CR> + <LF> at the end of the string 00012 void ESP8266::AddEOL(char * s) { 00013 char k; 00014 k = strlen(s); // Finds position of NULL character 00015 s[k] = 0x0D; // switch NULL for <CR> 00016 s[k + 1] = 0x0A; // Add <LF> 00017 s[k + 2] = 0; // Add NULL at the end 00018 } 00019 00020 // Add one ASCII character at the end of the string 00021 void ESP8266::AddChar(char * s, char c) { 00022 char k; 00023 k = strlen(s); 00024 s[k] = c; 00025 s[k + 1] = 0; 00026 } 00027 00028 // Converts integer number to null-terminated string 00029 void ESP8266::itoa(int n, char * s) { 00030 char k = 0; 00031 char r[11]; 00032 00033 if(n == 0) { 00034 s[0] = '0'; 00035 s[1] = 0; 00036 } else { 00037 while(n != 0) { 00038 r[k]= (n % 10) + '0'; 00039 n = n / 10; 00040 k++; 00041 } 00042 while(k > 0) { 00043 s[n] = r[k - 1] + '0'; 00044 n++; 00045 k--; 00046 } 00047 s[n] = 0; 00048 } 00049 } 00050 00051 // Sends command to ESP8266. Receives the command string 00052 void ESP8266::SendCMD(char * s) { 00053 AddEOL(s); 00054 comm.printf("%s", s); 00055 } 00056 00057 // Resets the ESP8266 00058 void ESP8266::Reset(void) { 00059 char rs[10]; 00060 strcpy(rs, "AT+RST"); 00061 SendCMD(rs); 00062 } 00063 00064 // Receive reply until no character is received after a given timeout in miliseconds 00065 void ESP8266::RcvReply(char * r, int to) { 00066 Timer t; 00067 bool ended = 0; 00068 char c; 00069 00070 strcpy(r, ""); 00071 t.start(); 00072 while(!ended) { 00073 if(comm.readable()) { 00074 c = comm.getc(); 00075 AddChar(r, c); 00076 t.start(); 00077 } 00078 if(t.read_ms() > to) { 00079 ended = 1; 00080 } 00081 } 00082 AddChar(r, 0x00); 00083 } 00084 00085 // Gets the AP list. Parameter: the string to receive the list 00086 void ESP8266::GetList(char * l) { 00087 char rs[15]; 00088 strcpy(rs, "AT+CWLAP"); 00089 SendCMD(rs); 00090 RcvReply(l, 5000); // Needs big timeout because it takes long to start replying 00091 } 00092 00093 // Joins a Wifi AP. Parameters: SSID and Password (strings) 00094 void ESP8266::Join(char * id, char * pwd) { 00095 char cmd[255]; 00096 strcpy(cmd, "AT+CWJAP="); 00097 AddChar(cmd, 0x22); 00098 strcat(cmd, id); 00099 AddChar(cmd, 0x22); 00100 AddChar(cmd, 0x2C); 00101 AddChar(cmd, 0x22); 00102 strcat(cmd, pwd); 00103 AddChar(cmd, 0x22); 00104 SendCMD(cmd); 00105 } 00106 00107 // Gets ESP IP. Parameter: string to contain IP 00108 void ESP8266::GetIP(char * ip) { 00109 char cmd[15]; 00110 strcpy(cmd, "AT+CIFSR"); 00111 SendCMD(cmd); 00112 RcvReply(ip, 2000); 00113 } 00114 00115 //Defines wifi mode; Parameter: mode; 1= STA, 2= AP, 3=both 00116 void ESP8266::SetMode(char mode) { 00117 char cmd[15]; 00118 strcpy(cmd, "AT+CWMODE="); 00119 mode = mode + 0x30; // Converts number into corresponding ASCII character 00120 AddChar(cmd, mode); // Completes command string 00121 SendCMD(cmd); 00122 } 00123 00124 // Quits the AP 00125 void ESP8266::Quit(void) { 00126 char cmd[15]; 00127 strcpy(cmd, "AT+CWQAP"); 00128 SendCMD(cmd); 00129 } 00130 00131 // Sets single connection 00132 void ESP8266::SetSingle(void) { 00133 char cmd[15]; 00134 strcpy(cmd, "AT+CIPMUX=0"); 00135 SendCMD(cmd); 00136 } 00137 00138 // Sets multiple connection 00139 void ESP8266::SetMultiple(void) { 00140 char rs[15]; 00141 strcpy(rs, "AT+CIPMUX=1"); 00142 SendCMD(rs); 00143 } 00144 00145 // Gets connection status. Parameter: string to contain status 00146 void ESP8266::GetConnStatus(char * st) { 00147 char cmd[15]; 00148 strcpy(cmd, "AT+CIPSTATUS"); 00149 SendCMD(cmd); 00150 RcvReply(st, 2000); 00151 } 00152 00153 // Starts server mode. Parameter: port to be used 00154 void ESP8266::StartServerMode(int port) { 00155 char rs[25]; 00156 char t[4]; 00157 strcpy(rs, "AT+CIPSERVER=1,"); 00158 itoa(port, t); 00159 strcat(rs, t); 00160 SendCMD(rs); 00161 } 00162 00163 // Close server mode. 00164 void ESP8266::CloseServerMode(void) { 00165 char rs[20]; 00166 strcpy(rs, "AT+CIPSERVER=0"); 00167 SendCMD(rs); 00168 }
Generated on Tue Jul 12 2022 14:11:10 by
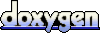