
SRK Version of mDot LoRa_Sensormode_SRK
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sensornode by
uBloxConfig.h
00001 /** 00002 * @file uBloxConfig.h 00003 * 00004 * @author Adrian 00005 * @date 30.05.2016 00006 * 00007 */ 00008 #include <string> 00009 #include <map> 00010 #include <vector> 00011 #include <stdint.h> 00012 #ifndef UBLOXCONFIG_H_ 00013 #define UBLOXCONFIG_H_ 00014 00015 00016 #define UBLOX_DISABLE_ALL_STRING "DISABLE ALL STRINGS" 00017 #define UBLOX_ENABLE_POSLLH_STRING "ENABLE POSLLH STRING" 00018 #define UBLOX_GNSS_ON "GNSS ON" 00019 #define UBLOX_GNSS_OFF "GNSS OFF" 00020 #define UBLOX_CONTINUOUS_MODE "CONTINUOUS MODE" 00021 #define UBLOX_POWER_SAVE_MODE "POWER SAVE MODE" 00022 #define UBLOX_ECO_MODE "POWER SAVE MODE" 00023 00024 /** 00025 * uBlox Modes. Modes define Sensor functionality 00026 */ 00027 enum uBLOX_MODE{ 00028 uBLOX_MODE_0,//!< uBLOX_MODE_0 00029 uBLOX_MODE_1,//!< uBLOX_MODE_1 00030 uBLOX_MODE_2,//!< uBLOX_MODE_2 00031 uBLOX_MODE_3,//!< uBLOX_MODE_3 00032 }; 00033 00034 /** 00035 * @class uBloxConfig 00036 * @brief A configuration container for the uBlox. 00037 * All its configuration values are stored an held inside 00038 * this Class. Depending on the uBLOX_MODE it sets all the configuration values. 00039 */ 00040 class uBloxConfig { 00041 public: 00042 uBloxConfig(); 00043 virtual ~uBloxConfig(); 00044 00045 /** 00046 * @brief Generates a configuration according to the chosen uBLOX_MODE. More exactly it builds 00047 * the strings that have to be sent at the initialization of the uBlox to run the uBlox to 00048 * run it in a specific configuration 00049 * @param desiredMode the mode to build the configuration according to 00050 */ 00051 void build(uBLOX_MODE); 00052 00053 std::vector< std::vector<uint8_t> > getInitialConfigurationString(); 00054 private: 00055 00056 std::map<std::string,std::vector<uint8_t> > configCommands; 00057 std::vector< std::vector<uint8_t> > initialCommands; 00058 00059 /** 00060 * @brief Connects a command and its string that consists of Bytes 00061 * @param command a command string describing the command 00062 * @param string the content of the command 00063 */ 00064 void connectCommandAndString(std::string command, uint8_t* string, uint8_t stringSize); 00065 00066 00067 }; 00068 00069 #endif /* UBLOXCONFIG_H_ */
Generated on Wed Jul 13 2022 09:23:48 by
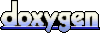