
SRK Version of mDot LoRa_Sensormode_SRK
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sensornode by
TaskLight.cpp
00001 /* 00002 * TaskLight.cpp 00003 * 00004 * Created on: May 27, 2016 00005 * Author: Adrian 00006 */ 00007 00008 #include "TaskLight.h " 00009 #include "rtos_idle.h" 00010 00011 00012 00013 TaskLight::TaskLight(MAX44009* max44009,Mutex* mutexI2C, Queue<MAX44009Message,LIGHT_QUEUE_LENGHT>* queue){ 00014 this->max44009 = max44009; 00015 setPriority(osPriorityNormal); 00016 setStackSize(DEFAULT_STACK_SIZE); 00017 setStackPointer(NULL); 00018 setMutex(mutexI2C); 00019 setQueue(queue); 00020 } 00021 00022 TaskLight::TaskLight(MAX44009* max44009,rtos::Mutex* mutexI2C, 00023 rtos::Queue<MAX44009Message,LIGHT_QUEUE_LENGHT>* queue, 00024 osPriority priority, uint32_t stackSize, unsigned char *stackPointer){ 00025 this->max44009 = max44009; 00026 setMutex(mutexI2C); 00027 setQueue(queue); 00028 setPriority(priority); 00029 setStackSize(stackSize); 00030 setStackPointer(stackPointer); 00031 setState(SLEEPING); 00032 } 00033 00034 TaskLight::~TaskLight() { 00035 // TODO Auto-generated destructor stub 00036 } 00037 00038 osStatus TaskLight::start(){ 00039 setState(RUNNING); 00040 this->thread = new rtos::Thread(callBack,this); 00041 this->thread->attach_idle_hook(NULL); 00042 } 00043 00044 osStatus TaskLight::stop(){ 00045 thread->terminate(); 00046 setState(SLEEPING); 00047 delete this->thread; 00048 } 00049 00050 void TaskLight::callBack(void const* data){ 00051 // WOODHAMMER METHOD of Casting! 00052 const TaskLight* constInstance = static_cast<const TaskLight* >(data); 00053 TaskLight* instance = const_cast<TaskLight*>(constInstance); 00054 00055 instance->measureLight(); 00056 } 00057 00058 void TaskLight::measureLight(){ 00059 MAX44009Message* max44009Message = new MAX44009Message(); 00060 00061 while(true){ 00062 mutexI2C->lock(osWaitForever); 00063 max44009Message->setLux(max44009->getLux()); 00064 mutexI2C->unlock(); 00065 00066 queue->put(max44009Message,osWaitForever); 00067 osDelay(LIGHT_TASK_DELAY_MS); 00068 } 00069 00070 } 00071 00072 void TaskLight::setQueue(Queue<MAX44009Message,LIGHT_QUEUE_LENGHT>* queue){ 00073 this->queue = queue; 00074 } 00075 00076 void TaskLight::setMutex(Mutex* mutex){ 00077 this->mutexI2C = mutex; 00078 } 00079 00080 void TaskLight::setPriority(osPriority priority){ 00081 this->priority = priority; 00082 } 00083 00084 void TaskLight::setStackSize(uint32_t stacksize){ 00085 this->stack_size = stacksize; 00086 } 00087 00088 void TaskLight::setStackPointer(unsigned char* stackPointer){ 00089 this->stack_pointer = stackPointer; 00090 } 00091 00092 void TaskLight::setState(TASK_STATE state){ 00093 this->state = state; 00094 } 00095 00096 TASK_STATE TaskLight::getState(){ 00097 return state; 00098 }
Generated on Wed Jul 13 2022 09:23:48 by
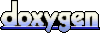