
SRK Version of mDot LoRa_Sensormode_SRK
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sensornode by
BME280Config.h
00001 /** 00002 * @file BME280Config.h 00003 * 00004 * @author Adrian 00005 * @date 24.05.2016 00006 * 00007 */ 00008 #include <stdint.h> 00009 00010 #ifndef APP_BME280CONFIG_H_ 00011 #define APP_BME280CONFIG_H_ 00012 00013 // CTRL_HUM Humidity over sampling Settings 00014 #define BME280_HUM_SKPPD 0b000 00015 #define BME280_HUM_OVRS_1 0b001 00016 #define BME280_HUM_OVRS_2 0b010 00017 #define BME280_HUM_OVRS_4 0b011 00018 #define BME280_HUM_OVRS_8 0b100 00019 #define BME280_HUM_OVRS_16 0b101 00020 00021 // CTRL_HUM Pressure over sampling Settings 00022 #define BME280_PRESS_SKPPD 0b000 00023 #define BME280_PRESS_OVRS_1 0b001 00024 #define BME280_PRESS_OVRS_2 0b010 00025 #define BME280_PRESS_OVRS_4 0b011 00026 #define BME280_PRESS_OVRS_8 0b100 00027 #define BME280_PRESS_OVRS_16 0b101 00028 00029 // CTRL_HUM Temperature over sampling Settings 00030 #define BME280_TEMP_SKPPD 0b000 00031 #define BME280_TEMP_OVRS_1 0b001 00032 #define BME280_TEMP_OVRS_2 0b010 00033 #define BME280_TEMP_OVRS_4 0b011 00034 #define BME280_TEMP_OVRS_8 0b100 00035 #define BME280_TEMP_OVRS_16 0b101 00036 00037 // CTRL_MEAS Settings Modes 00038 #define BME280_SLEEP_MODE 0b00 /**< Sleep mode */ 00039 #define BME280_FORCED_MODE 0b01 /**< Forced mode */ 00040 #define BME280_NORMAL_MODE 0b11 /**< Normal mode */ 00041 00042 //Oversampling Settings 00043 #define BME280_WHEAT_OVRS_T_P (BME280_TEMP_OVRS_1<<5)|(BME280_PRESS_OVRS_1<<2)|BME280_NORMAL_MODE 00044 #define BME280_WHEAT_OVRS_H BME280_HUM_OVRS_1 00045 00046 00047 /** 00048 * BME280 Modes. Modes define sensor functionality 00049 */ 00050 enum BME280_MODE{ 00051 BME280_MODE_0,//!< BME280_MODE_0 00052 BME280_MODE_1,//!< BME280_MODE_1 00053 BME280_MODE_2,//!< BME280_MODE_2 00054 BME280_MODE_3,//!< BME280_MODE_3 00055 BME280_MODE_4,//!< BME280_MODE_4 00056 BME280_MODE_5,//!< BME280_MODE_5 00057 BME280_MODE_6,//!< BME280_MODE_6 00058 BME280_MODE_7,//!< BME280_MODE_7 00059 BME280_MODE_8,//!< BME280_MODE_8 00060 BME280_MODE_9,//!< BME280_MODE_9 00061 }; 00062 00063 /** 00064 * @class BME280Config 00065 * @brief A configuration container for the BME280 Sensor. 00066 * All its configuration values are stored an held inside 00067 * this Class. Depending on the BME280_MODE it sets all the configuration values. 00068 */ 00069 class BME280Config { 00070 public: 00071 BME280Config(); 00072 virtual ~BME280Config(); 00073 00074 /** 00075 * @brief Generates a configuration according to the chosen BME280_MODE 00076 * @param desiredMode the mode to build the configuration according to 00077 */ 00078 void build(BME280_MODE); 00079 00080 /** 00081 * Gets the temperature measurements oversampling value for the configuration and initialization of the BME280 00082 * @return temperature oversampling register value 00083 */ 00084 uint8_t getOversamplingTemperature(); 00085 00086 /** 00087 * Gets the pressure measurements oversampling value for the configuration and initialization of the BME280 00088 * @return pressure oversampling register value 00089 */ 00090 uint8_t getOversamplingPressure(); 00091 00092 /** 00093 * Gets the humidity measurements oversampling value for the configuration and initialization of the BME280 00094 * @return humidity oversampling register value 00095 */ 00096 uint8_t getOversamplingHumidity(); 00097 00098 /** 00099 * Gets the sensor internal Mode 00100 * @return sensor internal Mode 00101 */ 00102 uint8_t getMode(); 00103 00104 private: 00105 uint8_t oversamplingTemperature; 00106 uint8_t oversamplingPressure; 00107 uint8_t oversamplingHumidity; 00108 00109 uint8_t mode; 00110 00111 00112 /** 00113 * @brief Sets the oversampling Value for Temperature measurements 00114 * @param oversamplingTemperature oversampling Value for Temperature measurements 00115 */ 00116 void setOversamplingTemperature(uint8_t oversamplingTemperature); 00117 00118 /** 00119 * @brief Sets the oversampling Value for Pressure measurements 00120 * @param oversamplingPressure oversampling Value for Pressure measurements 00121 */ 00122 void setOversamplingPressure(uint8_t oversamplingPressure); 00123 00124 /** 00125 * @brief Sets the oversampling Value for Humidity measurements 00126 * @param oversamplingHumidity oversampling Value for Humidity measurements 00127 */ 00128 void setOversamplingHumidity(uint8_t oversamplingHumidity); 00129 00130 /** 00131 * Set the internal mode of the BME280 00132 * @param desiredMode the internal mode of the BME280 00133 */ 00134 void setMode(uint8_t desiredMode); 00135 }; 00136 00137 #endif /* APP_BME280CONFIG_H_ */
Generated on Wed Jul 13 2022 09:23:47 by
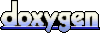