
SRK Version of mDot LoRa_Sensormode_SRK
Dependencies: libmDot mbed-rtos mbed
Fork of mDot_LoRa_Sensornode by
ApplicationConfig.h
00001 /* 00002 * ApplicationConfig.h 00003 * 00004 * Created on: Jun 3, 2016 00005 * Author: Adrian 00006 */ 00007 #include "BME280Config.h " 00008 #include "MPU9250Config.h " 00009 #include "uBloxConfig.h " 00010 #include "MAX44009Config.h " 00011 #include "SI1143Config.h " 00012 #include "LoRaConfig.h " 00013 #include "main.h" 00014 #ifndef APPLICATIONCONFIG_H_ 00015 #define APPLICATIONCONFIG_H_ 00016 00017 /** 00018 * Application Modes. Modes define different Usages of the LoRa sensor node 00019 */ 00020 enum APPLICATION_MODE { 00021 APPLICATION_MODE_1, //!< APPLICATION_MODE_1 00022 APPLICATION_MODE_2, //!< APPLICATION_MODE_2 00023 APPLICATION_MODE_3, //!< APPLICATION_MODE_3 00024 APPLICATION_MODE_4, //!< APPLICATION_MODE_4 00025 APPLICATION_MODE_5, //!< APPLICATION_MODE_5 00026 APPLICATION_MODE_6, //!< APPLICATION_MODE_6 00027 APPLICATION_MODE_7, //!< APPLICATION_MODE_7 00028 APPLICATION_MODE_8, //!< APPLICATION_MODE_8 00029 APPLICATION_MODE_9, //!< APPLICATION_MODE_9 00030 APPLICATION_MODE_10, //!< APPLICATION_MODE_10 00031 APPLICATION_MODE_11, //!< APPLICATION_MODE_11 00032 APPLICATION_MODE_99, //!< APPLICATION_MODE_99 00033 APPLICATION_MODE_TEST, //!< APPLICATION_MODE_TEST 00034 APPLICATION_MODE_TEST_MAX44009, //!< APPLICATION_MODE_TEST_MAX44009 00035 APPLICATION_MODE_TEST_BME280, //!< APPLICATION_MODE_TEST_BME280 00036 APPLICATION_MODE_TEST_MPU9250, //!< APPLICATION_MODE_TEST_MPU9250 00037 APPLICATION_MODE_TEST_SI1143, //!< APPLICATION_MODE_TEST_SI1143 00038 APPLICATION_MODE_TEST_uBlox, //!< APPLICATION_MODE_TEST_uBlox 00039 APPLICATION_MODE_LORA_MEASUREMENT,//!< APPLICATION_MODE_LORA_MEASUREMENT 00040 WEATHER_MEASUREMENT, //!< WEATHER_STATION 00041 ORIENTATATION_MEASUREMENT, //!< ORIENTATATION_SENSOR 00042 DISTANCE_MEASUREMENT, //!< DISTANCE_SENSOR 00043 SCS_MEASUREMENT, //!< SCS TESTING 00044 }; 00045 00046 00047 class ApplicationConfig { 00048 public: 00049 ApplicationConfig(); 00050 virtual ~ApplicationConfig(); 00051 00052 /** 00053 * @brief Generates a configuration according to the chosen APPLICATION_MODE 00054 * @param desiredMode the mode to build the configuration according to 00055 */ 00056 void build(APPLICATION_MODE desiredMode); 00057 00058 00059 /** 00060 * @brief Get Information about the MAX44009_MODE of the actual ApplicationConfig 00061 * @return the actual MAX44009_MODE 00062 */ 00063 MAX44009_MODE getMAX44009_MODE(); 00064 00065 /** 00066 * @brief Get Information about the BME280_MODE of the actual ApplicationConfig 00067 * @return the actual BME280_MODE 00068 */ 00069 BME280_MODE getBME280_MODE(); 00070 00071 /** 00072 * @brief Get Information about the MPU9250_MODE of the actual ApplicationConfig 00073 * @return the actual MPU9250_MODE 00074 */ 00075 MPU9250_MODE getMPU9250_MODE(); 00076 00077 /** 00078 * @brief Get Information about the SI1143_MODE of the actual ApplicationConfig 00079 * @return the actual SI1143_MODE 00080 */ 00081 SI1143_MODE getSI1143_MODE(); 00082 00083 /** 00084 * @brief Get Information about the uBLOX_MODE of the actual ApplicationConfig 00085 * @return the actual uBLOX_MODE 00086 */ 00087 uBLOX_MODE getuBlox_MODE(); 00088 00089 /** 00090 * @brief Get Information about the LORA_MODE of the actual ApplicationConfig 00091 * @return the actual LORA_MODE 00092 */ 00093 LORA_MODE getLORA_MODE(); 00094 00095 00096 /** 00097 * @brief Get Information about the TASK_STATE of the TaskLight in the actual ApplicationConfig 00098 * @return the actual TASK_STATE (RUNNING,SLEEPING) of TaskLight 00099 */ 00100 TASK_STATE getStateTaskLight(); 00101 00102 /** 00103 * @brief Get Information about the TASK_STATE of the TaskTemperature in the actual ApplicationConfig 00104 * @return the actual TASK_STATE (RUNNING,SLEEPING) of TaskTemperature 00105 */ 00106 TASK_STATE getStateTaskTemperature(); 00107 00108 /** 00109 * @brief Get Information about the TASK_STATE of the TaskPressure in the actual ApplicationConfig 00110 * @return the actual TASK_STATE (RUNNING,SLEEPING) of TaskPressure 00111 */ 00112 TASK_STATE getStateTaskPressure(); 00113 00114 /** 00115 * @brief Get Information about the TASK_STATE of the TaskHumidity in the actual ApplicationConfig 00116 * @return the actual TASK_STATE (RUNNING,SLEEPING) of TaskHumidity 00117 */ 00118 TASK_STATE getStateTaskHumidity(); 00119 00120 /** 00121 * @brief Get Information about the TASK_STATE of the TaskAcceleration in the actual ApplicationConfig 00122 * @return the actual TASK_STATE (RUNNING,SLEEPING) of TaskAcceleration 00123 */ 00124 TASK_STATE getStateTaskAcceleration(); 00125 00126 /** 00127 * @brief Get Information about the TASK_STATE of the TaskGyroscope in the actual ApplicationConfig 00128 * @return the actual TASK_STATE (RUNNING,SLEEPING) of TaskGyroscope 00129 */ 00130 TASK_STATE getStateTaskGyroscope(); 00131 00132 /** 00133 * @brief Get Information about the TASK_STATE of the TaskTesla in the actual ApplicationConfig 00134 * @return the actual TASK_STATE (RUNNING,SLEEPING) of TaskTesla 00135 */ 00136 TASK_STATE getStateTaskTesla(); 00137 00138 /** 00139 * @brief Get Information about the TASK_STATE of the TaskProximity in the actual ApplicationConfig 00140 * @return the actual TASK_STATE (RUNNING,SLEEPING) of TaskProximity 00141 */ 00142 TASK_STATE getStateTaskProximity(); 00143 00144 /** 00145 * @brief Get Information about the TASK_STATE of the TaskGPS in the actual ApplicationConfig 00146 * @return the actual TASK_STATE (RUNNING,SLEEPING) of TaskGPS 00147 */ 00148 TASK_STATE getStateTaskGPS(); 00149 00150 /** 00151 * @brief Get Information about the TASK_STATE of the TaskLoRaMeasurement in the actual ApplicationConfig 00152 * @return the actual TASK_STATE (RUNNING,SLEEPING) of TaskLoRaMeasurement 00153 */ 00154 TASK_STATE getStateTaskLoRaMeasurement(); 00155 00156 /** 00157 * @brief Get Information about the LORA_STATE of the LoRa Module in the actual ApplicationConfig 00158 * @return the actual LORA_STATE (ON,OFF) of TaskLoRaMeasurement 00159 */ 00160 LORA_STATE getStateLoRa(); 00161 00162 private: 00163 MAX44009_MODE max44009Mode; 00164 BME280_MODE bme280Mode; 00165 MPU9250_MODE mpu9250Mode; 00166 SI1143_MODE si1143Mode; 00167 uBLOX_MODE ubloxMode; 00168 LORA_MODE loraMode; 00169 00170 TASK_STATE stateTaskLight; 00171 TASK_STATE stateTaskTemperature; 00172 TASK_STATE stateTaskPressure; 00173 TASK_STATE stateTaskHumidity; 00174 TASK_STATE stateTaskAcceleration; 00175 TASK_STATE stateTaskGyroscope; 00176 TASK_STATE stateTaskTesla; 00177 TASK_STATE stateTaskProximity; 00178 TASK_STATE stateTaskGPS; 00179 TASK_STATE stateTaskLoraMeasurement; 00180 00181 LORA_STATE stateLoRa; 00182 00183 /** 00184 * @brief Sets the MAX44009_MODE for that the MAX44009 Sensor has to be initialized for the actual ApplicationConfig 00185 * @param the diseredMode 00186 */ 00187 void setMAX44009_MODE(MAX44009_MODE desiredMode); 00188 00189 /** 00190 * @brief Sets the BME280_MODE for that the BME280 Sensor has to be initialized for the actual ApplicationConfig 00191 * @param the diseredMode 00192 */ 00193 void setBME280_MODE(BME280_MODE desiredMode); 00194 00195 /** 00196 * @brief Sets the MPU9250_MODE for that the MPU9250 Sensor has to be initialized for the actual ApplicationConfig 00197 * @param the diseredMode 00198 */ 00199 void setMPU9250_MODE(MPU9250_MODE desiredMode); 00200 00201 /** 00202 * @brief Sets the SI1143_MODE for that the SI1143 Sensor has to be initialized for the actual ApplicationConfig 00203 * @param the diseredMode 00204 */ 00205 void setSI1143_MODE(SI1143_MODE desiredMode); 00206 00207 /** 00208 * @brief Sets the uBLOX_MODE for that the uBlox Sensor has to be initialized for the actual ApplicationConfig 00209 * @param the diseredMode 00210 */ 00211 void setuBlox_MODE(uBLOX_MODE desiredMode); 00212 00213 /** 00214 * @brief Sets the LORA_MODE for that the LORA device has to be initialized for the actual ApplicationConfig 00215 * @param the diseredMode 00216 */ 00217 void setLORA_MODE(LORA_MODE desiredMode); 00218 00219 00220 /** 00221 * @brief Sets the TASK_STATE (RUNNING,SLEEPING) of TaskLight for the actual ApplicationConfig 00222 * @param the desired State 00223 */ 00224 void setStateTaskLight(TASK_STATE desiredState); 00225 00226 /** 00227 * @brief Sets the TASK_STATE (RUNNING,SLEEPING) of TaskTemperature for the actual ApplicationConfig 00228 * @param the desired State 00229 */ 00230 void setStateTaskTemperature(TASK_STATE desiredState); 00231 00232 /** 00233 * @brief Sets the TASK_STATE (RUNNING,SLEEPING) of TaskPressure for the actual ApplicationConfig 00234 * @param the desired State 00235 */ 00236 void setStateTaskPressure(TASK_STATE desiredState); 00237 00238 /** 00239 * @brief Sets the TASK_STATE (RUNNING,SLEEPING) of TaskHumidity for the actual ApplicationConfig 00240 * @param the desired State 00241 */ 00242 void setStateTaskHumidity(TASK_STATE desiredState); 00243 00244 /** 00245 * @brief Sets the TASK_STATE (RUNNING,SLEEPING) of TaskAcceleration for the actual ApplicationConfig 00246 * @param the desired State 00247 */ 00248 void setStateTaskAcceleration(TASK_STATE desiredState); 00249 00250 /** 00251 * @brief Sets the TASK_STATE (RUNNING,SLEEPING) of TaskGyroscope for the actual ApplicationConfig 00252 * @param the desired State 00253 */ 00254 void setStateTaskGyroscope(TASK_STATE desiredState); 00255 00256 /** 00257 * @brief Sets the TASK_STATE (RUNNING,SLEEPING) of TaskTesla for the actual ApplicationConfig 00258 * @param the desired State 00259 */ 00260 void setStateTaskTesla(TASK_STATE desiredState); 00261 00262 /** 00263 * @brief Sets the TASK_STATE (RUNNING,SLEEPING) of TaskProximity for the actual ApplicationConfig 00264 * @param the desired State 00265 */ 00266 void setStateTaskProximity(TASK_STATE desiredState); 00267 00268 /** 00269 * @brief Sets the TASK_STATE (RUNNING,SLEEPING) of TaskGPS for the actual ApplicationConfig 00270 * @param the desired State 00271 */ 00272 void setStateTaskGPS(TASK_STATE desiredState); 00273 00274 /** 00275 * @brief Sets the TASK_STATE (RUNNING,SLEEPING) of TaskLoRaMeasurement for the actual ApplicationConfig 00276 * @param the desired State 00277 */ 00278 void setStateTaskLoRaMeasurement(TASK_STATE desiredState); 00279 /** 00280 * @brief Sets the LORA_STATE (ON,OFF) of LoRa Device for the actual ApplicationConfig 00281 * @param the desired State 00282 */ 00283 void setStateLoRa(LORA_STATE); 00284 00285 }; 00286 00287 #endif /* APPLICATIONCONFIG_H_ */
Generated on Wed Jul 13 2022 09:23:47 by
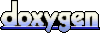