The code from https://github.com/vpcola/Nucleo
Embed:
(wiki syntax)
Show/hide line numbers
Shell.cpp
00001 #include "mbed.h" 00002 #include "cmsis_os.h" 00003 00004 #include <stdlib.h> 00005 #include <string.h> 00006 #include <vector> 00007 #include <string> 00008 #include "Shell.h" 00009 #include "Utility.h" 00010 #include "DHT.h" 00011 #include "SDFileSystem.h" 00012 #include "SPI_TFT_ILI9341.h" 00013 00014 00015 extern DHT dht; 00016 extern SDFileSystem sdcard; 00017 extern I2C i2c; 00018 extern SPI_TFT_ILI9341 TFT; 00019 extern std::vector<std::string> images; 00020 00021 void shellUsage(Stream * chp, const char *p) 00022 { 00023 chp->printf("Usage: %s\r\n", p); 00024 } 00025 00026 static void list_commands(Stream *chp, const ShellCommand *scp) { 00027 00028 while (scp->sc_name != NULL) { 00029 chp->printf("%s ", scp->sc_name); 00030 scp++; 00031 } 00032 } 00033 00034 static void cmd_info(Stream * chp, int argc, char *argv[]) 00035 { 00036 00037 (void)argv; 00038 if (argc > 0) { 00039 shellUsage(chp, "info"); 00040 return; 00041 } 00042 00043 long sp = __current_sp(); 00044 long pc = __current_pc(); 00045 00046 00047 heapinfo(chp); 00048 00049 } 00050 00051 00052 static ShellCommand local_commands[] = { 00053 {"info", cmd_info}, 00054 {NULL, NULL} 00055 }; 00056 00057 static bool cmdexec(const ShellCommand *scp, Stream * chp, 00058 char *name, int argc, char *argv[]) 00059 { 00060 while (scp->sc_name != NULL) { 00061 if (strcasecmp(scp->sc_name, name) == 0) { 00062 scp->sc_func(chp, argc, argv); 00063 return false; 00064 } 00065 scp++; 00066 } 00067 return true; 00068 } 00069 00070 void shellStart(const ShellConfig *p) 00071 { 00072 int n; 00073 Stream *chp = p->sc_channel; 00074 const ShellCommand *scp = p->sc_commands; 00075 char *lp, *cmd, *tokp, line[SHELL_MAX_LINE_LENGTH]; 00076 char *args[SHELL_MAX_ARGUMENTS + 1]; 00077 00078 chp->printf("\r\nEmbed/RX Shell\r\n"); 00079 while (true) { 00080 chp->printf(">> "); 00081 if (shellGetLine(chp, line, sizeof(line))) { 00082 chp->printf("\r\nlogout"); 00083 break; 00084 } 00085 lp = _strtok(line, " \t", &tokp); 00086 cmd = lp; 00087 n = 0; 00088 while ((lp = _strtok(NULL, " \t", &tokp)) != NULL) { 00089 if (n >= SHELL_MAX_ARGUMENTS) { 00090 chp->printf("too many arguments\r\n"); 00091 cmd = NULL; 00092 break; 00093 } 00094 args[n++] = lp; 00095 } 00096 args[n] = NULL; 00097 if (cmd != NULL) { 00098 if (strcasecmp(cmd, "exit") == 0) { 00099 if (n > 0) { 00100 shellUsage(chp, "exit"); 00101 continue; 00102 } 00103 // Break here breaks the outer loop 00104 // hence, we exit the shell. 00105 break; 00106 } 00107 else if (strcasecmp(cmd, "help") == 0) { 00108 if (n > 0) { 00109 shellUsage(chp, "help"); 00110 continue; 00111 } 00112 chp->printf("Commands: help exit "); 00113 list_commands(chp, local_commands); 00114 if (scp != NULL) 00115 list_commands(chp, scp); 00116 chp->printf("\r\n"); 00117 } 00118 else if (cmdexec(local_commands, chp, cmd, n, args) && 00119 ((scp == NULL) || cmdexec(scp, chp, cmd, n, args))) { 00120 chp->printf("%s", cmd); 00121 chp->printf(" ?\r\n"); 00122 } 00123 } 00124 } 00125 } 00126 00127 bool shellGetLine(Stream *chp, char *line, unsigned size) { 00128 char *p = line; 00129 00130 while (true) { 00131 char c; 00132 00133 if ((c = chp->getc()) == 0) 00134 return true; 00135 if (c == 4) { 00136 chp->printf("^D"); 00137 return true; 00138 } 00139 if (c == 8) { 00140 if (p != line) { 00141 chp->putc(c); 00142 chp->putc(0x20); 00143 chp->putc(c); 00144 p--; 00145 } 00146 continue; 00147 } 00148 if (c == '\r') { 00149 chp->printf("\r\n"); 00150 *p = 0; 00151 return false; 00152 } 00153 if (c < 0x20) 00154 continue; 00155 if (p < line + size - 1) { 00156 chp->putc(c); 00157 *p++ = (char)c; 00158 } 00159 } 00160 } 00161 00162 /** 00163 * \brief List Directories and files 00164 * \param none 00165 * \return int 00166 **/ 00167 00168 void cmd_ls(Stream * chp, int argc, char * argv[]) 00169 { 00170 DIR * dp; 00171 struct dirent * dirp; 00172 FILINFO fileInfo; 00173 char dirroot[256]; 00174 00175 if (argc >= 1) 00176 sprintf(dirroot, "/sd/%s", argv[0]); 00177 else 00178 sprintf(dirroot, "/sd"); 00179 00180 chp->printf("Listing directory [%s]\r\n", dirroot); 00181 00182 dp = opendir(dirroot); 00183 while((dirp = readdir(dp)) != NULL) 00184 { 00185 if (sdcard.stat(dirp->d_name, &fileInfo) == 0) 00186 { 00187 if (fileInfo.fattrib & AM_DIR ) 00188 chp->printf("<DIR>\t\t"); 00189 else 00190 chp->printf("%ld\t\t", fileInfo.fsize); 00191 } 00192 chp->printf("%s\r\n", dirp->d_name); 00193 } 00194 closedir(dp); 00195 } 00196 00197 void cmd_sensor(Stream * chp, int argc, char * argv[]) 00198 { 00199 float temperature, humidity; 00200 00201 00202 if (dht.readData() == ERROR_NONE) 00203 { 00204 temperature = dht.ReadTemperature(CELCIUS); 00205 humidity = dht.ReadHumidity(); 00206 00207 chp->printf("Temperature %.2fC \r\n", temperature); 00208 chp->printf("Humidity %.2f%% \r\n", humidity); 00209 chp->printf("Dew point %.2f\r\n", dht.CalcdewPoint(temperature, humidity)); 00210 } 00211 } 00212 00213 void cmd_load(Stream * chp, int argc, char * argv[]) 00214 { 00215 char filename[256]; 00216 00217 if (argc != 1) 00218 { 00219 shellUsage(chp, "load <bitmapfile>"); 00220 return; 00221 } 00222 00223 sprintf(filename, "/sd/%s", argv[0]); 00224 // Load a bitmap startup file 00225 int err = TFT.BMP_16(0,0, filename); 00226 if (err != 1) TFT.printf(" - Err: %d", err); 00227 00228 } 00229 00230 void cmd_rollimages(Stream * chp, int argc, char * argv[]) 00231 { 00232 for(std::vector<std::string>::iterator i = images.begin(); 00233 i != images.end(); i++) 00234 { 00235 chp->printf("Loading image %s...\r\n", (*i).c_str()); 00236 TFT.cls(); 00237 int err = TFT.BMP_16(0,0, (*i).c_str()); 00238 if (err != 1) chp->printf(" - Err: %d", err); 00239 00240 wait(1.0); 00241 heapinfo(chp); 00242 } 00243 } 00244
Generated on Tue Jul 12 2022 18:55:01 by
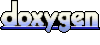