CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_var_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_var_f32.c 00009 * 00010 * Description: Variance of an array of F32 type. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * ---------------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupStats 00031 */ 00032 00033 /** 00034 * @defgroup variance Variance 00035 * 00036 * Calculates the variance of the elements in the input vector. 00037 * The underlying algorithm is used: 00038 * 00039 * <pre> 00040 * Result = (sumOfSquares - sum<sup>2</sup> / blockSize) / (blockSize - 1) 00041 * 00042 * where, sumOfSquares = pSrc[0] * pSrc[0] + pSrc[1] * pSrc[1] + ... + pSrc[blockSize-1] * pSrc[blockSize-1] 00043 * 00044 * sum = pSrc[0] + pSrc[1] + pSrc[2] + ... + pSrc[blockSize-1] 00045 * </pre> 00046 * 00047 * There are separate functions for floating point, Q31, and Q15 data types. 00048 */ 00049 00050 /** 00051 * @addtogroup variance 00052 * @{ 00053 */ 00054 00055 00056 /** 00057 * @brief Variance of the elements of a floating-point vector. 00058 * @param[in] *pSrc points to the input vector 00059 * @param[in] blockSize length of the input vector 00060 * @param[out] *pResult variance value returned here 00061 * @return none. 00062 * 00063 */ 00064 00065 00066 void arm_var_f32( 00067 float32_t * pSrc, 00068 uint32_t blockSize, 00069 float32_t * pResult) 00070 { 00071 float32_t sum = (float32_t) 0.0; /* Accumulator */ 00072 float32_t meanOfSquares, mean, in, squareOfMean; /* Temporary variables */ 00073 uint32_t blkCnt; /* loop counter */ 00074 float32_t *pIn; /* Temporary pointer */ 00075 00076 /* updating temporary pointer */ 00077 pIn = pSrc; 00078 00079 /*loop Unrolling */ 00080 blkCnt = blockSize >> 2u; 00081 00082 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00083 ** a second loop below computes the remaining 1 to 3 samples. */ 00084 while(blkCnt > 0u) 00085 { 00086 /* C = (A[0] * A[0] + A[1] * A[1] + ... + A[blockSize-1] * A[blockSize-1]) */ 00087 /* Compute Sum of squares of the input samples 00088 * and then store the result in a temporary variable, sum. */ 00089 in = *pSrc++; 00090 sum += in * in; 00091 in = *pSrc++; 00092 sum += in * in; 00093 in = *pSrc++; 00094 sum += in * in; 00095 in = *pSrc++; 00096 sum += in * in; 00097 00098 /* Decrement the loop counter */ 00099 blkCnt--; 00100 } 00101 00102 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00103 ** No loop unrolling is used. */ 00104 blkCnt = blockSize % 0x4u; 00105 00106 while(blkCnt > 0u) 00107 { 00108 /* C = (A[0] * A[0] + A[1] * A[1] + ... + A[blockSize-1] * A[blockSize-1]) */ 00109 /* Compute Sum of squares of the input samples 00110 * and then store the result in a temporary variable, sum. */ 00111 in = *pSrc++; 00112 sum += in * in; 00113 00114 /* Decrement the loop counter */ 00115 blkCnt--; 00116 } 00117 00118 /* Compute Mean of squares of the input samples 00119 * and then store the result in a temporary variable, meanOfSquares. */ 00120 meanOfSquares = sum / ((float32_t) blockSize - 1.0f); 00121 00122 /* Reset the accumulator */ 00123 sum = 0.0f; 00124 00125 /*loop Unrolling */ 00126 blkCnt = blockSize >> 2u; 00127 00128 /* Reset the input working pointer */ 00129 pSrc = pIn; 00130 00131 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00132 ** a second loop below computes the remaining 1 to 3 samples. */ 00133 while(blkCnt > 0u) 00134 { 00135 /* C = (A[0] + A[1] + A[2] + ... + A[blockSize-1]) */ 00136 /* Compute sum of all input values and then store the result in a temporary variable, sum. */ 00137 sum += *pSrc++; 00138 sum += *pSrc++; 00139 sum += *pSrc++; 00140 sum += *pSrc++; 00141 00142 /* Decrement the loop counter */ 00143 blkCnt--; 00144 } 00145 00146 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00147 ** No loop unrolling is used. */ 00148 blkCnt = blockSize % 0x4u; 00149 00150 while(blkCnt > 0u) 00151 { 00152 /* C = (A[0] + A[1] + A[2] + ... + A[blockSize-1]) */ 00153 /* Compute sum of all input values and then store the result in a temporary variable, sum. */ 00154 sum += *pSrc++; 00155 00156 /* Decrement the loop counter */ 00157 blkCnt--; 00158 } 00159 /* Compute mean of all input values */ 00160 mean = sum / (float32_t) blockSize; 00161 00162 /* Compute square of mean */ 00163 squareOfMean = (mean * mean) * (((float32_t) blockSize) / 00164 ((float32_t) blockSize - 1.0f)); 00165 00166 /* Compute variance and then store the result to the destination */ 00167 *pResult = meanOfSquares - squareOfMean; 00168 00169 } 00170 00171 /** 00172 * @} end of variance group 00173 */
Generated on Tue Jul 12 2022 14:13:55 by
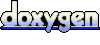