CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_sin_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_sin_f32.c 00009 * 00010 * Description: Fast sine calculation for floating-point values. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * -------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupFastMath 00031 */ 00032 00033 /** 00034 * @defgroup sin Sine 00035 * 00036 * Computes the trigonometric sine function using a combination of table lookup 00037 * and cubic interpolation. There are separate functions for 00038 * Q15, Q31, and floating-point data types. 00039 * The input to the floating-point version is in radians while the 00040 * fixed-point Q15 and Q31 have a scaled input with the range 00041 * [0 1) mapping to [0 2*pi). 00042 * 00043 * The implementation is based on table lookup using 256 values together with cubic interpolation. 00044 * The steps used are: 00045 * -# Calculation of the nearest integer table index 00046 * -# Fetch the four table values a, b, c, and d 00047 * -# Compute the fractional portion (fract) of the table index. 00048 * -# Calculation of wa, wb, wc, wd 00049 * -# The final result equals <code>a*wa + b*wb + c*wc + d*wd</code> 00050 * 00051 * where 00052 * <pre> 00053 * a=Table[index-1]; 00054 * b=Table[index+0]; 00055 * c=Table[index+1]; 00056 * d=Table[index+2]; 00057 * </pre> 00058 * and 00059 * <pre> 00060 * wa=-(1/6)*fract.^3 + (1/2)*fract.^2 - (1/3)*fract; 00061 * wb=(1/2)*fract.^3 - fract.^2 - (1/2)*fract + 1; 00062 * wc=-(1/2)*fract.^3+(1/2)*fract.^2+fract; 00063 * wd=(1/6)*fract.^3 - (1/6)*fract; 00064 * </pre> 00065 */ 00066 00067 /** 00068 * @addtogroup sin 00069 * @{ 00070 */ 00071 00072 00073 /** 00074 * \par 00075 * Example code for Generation of Floating-point Sin Table: 00076 * tableSize = 256; 00077 * <pre>for(n = -1; n < (tableSize + 1); n++) 00078 * { 00079 * sinTable[n+1]=sin(2*pi*n/tableSize); 00080 * }</pre> 00081 * \par 00082 * where pi value is 3.14159265358979 00083 */ 00084 00085 static const float32_t sinTable [259] = { 00086 -0.024541229009628296f, 0.000000000000000000f, 0.024541229009628296f, 00087 0.049067676067352295f, 0.073564566671848297f, 0.098017141222953796f, 00088 0.122410677373409270f, 0.146730467677116390f, 00089 0.170961886644363400f, 0.195090323686599730f, 0.219101235270500180f, 00090 0.242980182170867920f, 0.266712754964828490f, 0.290284663438797000f, 00091 0.313681751489639280f, 0.336889863014221190f, 00092 0.359895050525665280f, 0.382683426141738890f, 0.405241310596466060f, 00093 0.427555084228515630f, 0.449611335992813110f, 0.471396744251251220f, 00094 0.492898195981979370f, 0.514102756977081300f, 00095 0.534997642040252690f, 0.555570244789123540f, 0.575808167457580570f, 00096 0.595699310302734380f, 0.615231573581695560f, 0.634393274784088130f, 00097 0.653172850608825680f, 0.671558976173400880f, 00098 0.689540565013885500f, 0.707106769084930420f, 0.724247097969055180f, 00099 0.740951120853424070f, 0.757208824157714840f, 0.773010432720184330f, 00100 0.788346409797668460f, 0.803207516670227050f, 00101 0.817584812641143800f, 0.831469595432281490f, 0.844853579998016360f, 00102 0.857728600502014160f, 0.870086967945098880f, 0.881921291351318360f, 00103 0.893224298954010010f, 0.903989315032958980f, 00104 0.914209783077239990f, 0.923879504203796390f, 0.932992815971374510f, 00105 0.941544055938720700f, 0.949528157711029050f, 0.956940352916717530f, 00106 0.963776051998138430f, 0.970031261444091800f, 00107 0.975702106952667240f, 0.980785250663757320f, 0.985277652740478520f, 00108 0.989176511764526370f, 0.992479562759399410f, 0.995184719562530520f, 00109 0.997290432453155520f, 0.998795449733734130f, 00110 0.999698817729949950f, 1.000000000000000000f, 0.999698817729949950f, 00111 0.998795449733734130f, 0.997290432453155520f, 0.995184719562530520f, 00112 0.992479562759399410f, 0.989176511764526370f, 00113 0.985277652740478520f, 0.980785250663757320f, 0.975702106952667240f, 00114 0.970031261444091800f, 0.963776051998138430f, 0.956940352916717530f, 00115 0.949528157711029050f, 0.941544055938720700f, 00116 0.932992815971374510f, 0.923879504203796390f, 0.914209783077239990f, 00117 0.903989315032958980f, 0.893224298954010010f, 0.881921291351318360f, 00118 0.870086967945098880f, 0.857728600502014160f, 00119 0.844853579998016360f, 0.831469595432281490f, 0.817584812641143800f, 00120 0.803207516670227050f, 0.788346409797668460f, 0.773010432720184330f, 00121 0.757208824157714840f, 0.740951120853424070f, 00122 0.724247097969055180f, 0.707106769084930420f, 0.689540565013885500f, 00123 0.671558976173400880f, 0.653172850608825680f, 0.634393274784088130f, 00124 0.615231573581695560f, 0.595699310302734380f, 00125 0.575808167457580570f, 0.555570244789123540f, 0.534997642040252690f, 00126 0.514102756977081300f, 0.492898195981979370f, 0.471396744251251220f, 00127 0.449611335992813110f, 0.427555084228515630f, 00128 0.405241310596466060f, 0.382683426141738890f, 0.359895050525665280f, 00129 0.336889863014221190f, 0.313681751489639280f, 0.290284663438797000f, 00130 0.266712754964828490f, 0.242980182170867920f, 00131 0.219101235270500180f, 0.195090323686599730f, 0.170961886644363400f, 00132 0.146730467677116390f, 0.122410677373409270f, 0.098017141222953796f, 00133 0.073564566671848297f, 0.049067676067352295f, 00134 0.024541229009628296f, 0.000000000000000122f, -0.024541229009628296f, 00135 -0.049067676067352295f, -0.073564566671848297f, -0.098017141222953796f, 00136 -0.122410677373409270f, -0.146730467677116390f, 00137 -0.170961886644363400f, -0.195090323686599730f, -0.219101235270500180f, 00138 -0.242980182170867920f, -0.266712754964828490f, -0.290284663438797000f, 00139 -0.313681751489639280f, -0.336889863014221190f, 00140 -0.359895050525665280f, -0.382683426141738890f, -0.405241310596466060f, 00141 -0.427555084228515630f, -0.449611335992813110f, -0.471396744251251220f, 00142 -0.492898195981979370f, -0.514102756977081300f, 00143 -0.534997642040252690f, -0.555570244789123540f, -0.575808167457580570f, 00144 -0.595699310302734380f, -0.615231573581695560f, -0.634393274784088130f, 00145 -0.653172850608825680f, -0.671558976173400880f, 00146 -0.689540565013885500f, -0.707106769084930420f, -0.724247097969055180f, 00147 -0.740951120853424070f, -0.757208824157714840f, -0.773010432720184330f, 00148 -0.788346409797668460f, -0.803207516670227050f, 00149 -0.817584812641143800f, -0.831469595432281490f, -0.844853579998016360f, 00150 -0.857728600502014160f, -0.870086967945098880f, -0.881921291351318360f, 00151 -0.893224298954010010f, -0.903989315032958980f, 00152 -0.914209783077239990f, -0.923879504203796390f, -0.932992815971374510f, 00153 -0.941544055938720700f, -0.949528157711029050f, -0.956940352916717530f, 00154 -0.963776051998138430f, -0.970031261444091800f, 00155 -0.975702106952667240f, -0.980785250663757320f, -0.985277652740478520f, 00156 -0.989176511764526370f, -0.992479562759399410f, -0.995184719562530520f, 00157 -0.997290432453155520f, -0.998795449733734130f, 00158 -0.999698817729949950f, -1.000000000000000000f, -0.999698817729949950f, 00159 -0.998795449733734130f, -0.997290432453155520f, -0.995184719562530520f, 00160 -0.992479562759399410f, -0.989176511764526370f, 00161 -0.985277652740478520f, -0.980785250663757320f, -0.975702106952667240f, 00162 -0.970031261444091800f, -0.963776051998138430f, -0.956940352916717530f, 00163 -0.949528157711029050f, -0.941544055938720700f, 00164 -0.932992815971374510f, -0.923879504203796390f, -0.914209783077239990f, 00165 -0.903989315032958980f, -0.893224298954010010f, -0.881921291351318360f, 00166 -0.870086967945098880f, -0.857728600502014160f, 00167 -0.844853579998016360f, -0.831469595432281490f, -0.817584812641143800f, 00168 -0.803207516670227050f, -0.788346409797668460f, -0.773010432720184330f, 00169 -0.757208824157714840f, -0.740951120853424070f, 00170 -0.724247097969055180f, -0.707106769084930420f, -0.689540565013885500f, 00171 -0.671558976173400880f, -0.653172850608825680f, -0.634393274784088130f, 00172 -0.615231573581695560f, -0.595699310302734380f, 00173 -0.575808167457580570f, -0.555570244789123540f, -0.534997642040252690f, 00174 -0.514102756977081300f, -0.492898195981979370f, -0.471396744251251220f, 00175 -0.449611335992813110f, -0.427555084228515630f, 00176 -0.405241310596466060f, -0.382683426141738890f, -0.359895050525665280f, 00177 -0.336889863014221190f, -0.313681751489639280f, -0.290284663438797000f, 00178 -0.266712754964828490f, -0.242980182170867920f, 00179 -0.219101235270500180f, -0.195090323686599730f, -0.170961886644363400f, 00180 -0.146730467677116390f, -0.122410677373409270f, -0.098017141222953796f, 00181 -0.073564566671848297f, -0.049067676067352295f, 00182 -0.024541229009628296f, -0.000000000000000245f, 0.024541229009628296f 00183 }; 00184 00185 00186 /** 00187 * @brief Fast approximation to the trigonometric sine function for floating-point data. 00188 * @param[in] x input value in radians. 00189 * @return sin(x). 00190 */ 00191 00192 float32_t arm_sin_f32( 00193 float32_t x) 00194 { 00195 float32_t sinVal, fract, in; /* Temporary variables for input, output */ 00196 uint32_t index; /* Index variables */ 00197 uint32_t tableSize = (uint32_t) TABLE_SIZE; /* Initialise tablesize */ 00198 float32_t wa, wb, wc, wd; /* Cubic interpolation coefficients */ 00199 float32_t a, b, c, d; /* Four nearest output values */ 00200 float32_t *tablePtr; /* Pointer to table */ 00201 int32_t n; 00202 00203 /* input x is in radians */ 00204 /* Scale the input to [0 1] range from [0 2*PI] , divide input by 2*pi */ 00205 in = x * 0.159154943092f; 00206 00207 /* Calculation of floor value of input */ 00208 n = (int32_t) in; 00209 00210 /* Make negative values towards -infinity */ 00211 if(x < 0.0f) 00212 { 00213 n = n - 1; 00214 } 00215 00216 /* Map input value to [0 1] */ 00217 in = in - (float32_t) n; 00218 00219 /* Calculation of index of the table */ 00220 index = (uint32_t) (tableSize * in); 00221 00222 /* fractional value calculation */ 00223 fract = ((float32_t) tableSize * in) - (float32_t) index; 00224 00225 /* Initialise table pointer */ 00226 tablePtr = (float32_t *) & sinTable [index]; 00227 00228 /* Read four nearest values of output value from the sin table */ 00229 a = *tablePtr++; 00230 b = *tablePtr++; 00231 c = *tablePtr++; 00232 d = *tablePtr++; 00233 00234 /* Cubic interpolation process */ 00235 wa = -(((0.166666667f) * (fract * (fract * fract))) + 00236 ((0.3333333333333f) * fract)) + ((0.5f) * (fract * fract)); 00237 wb = (((0.5f) * (fract * (fract * fract))) - 00238 ((fract * fract) + ((0.5f) * fract))) + 1.0f; 00239 wc = (-((0.5f) * (fract * (fract * fract))) + 00240 ((0.5f) * (fract * fract))) + fract; 00241 wd = ((0.166666667f) * (fract * (fract * fract))) - 00242 ((0.166666667f) * fract); 00243 00244 /* Calculate sin value */ 00245 sinVal = ((a * wa) + (b * wb)) + ((c * wc) + (d * wd)); 00246 00247 /* Return the output value */ 00248 return (sinVal); 00249 00250 } 00251 00252 /** 00253 * @} end of sin group 00254 */
Generated on Tue Jul 12 2022 14:13:54 by
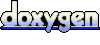