CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_scale_q7.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_scale_q7.c 00009 * 00010 * Description: Multiplies a Q7 vector by a scalar. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated 00025 * 00026 * Version 0.0.7 2010/06/10 00027 * Misra-C changes done 00028 * -------------------------------------------------------------------- */ 00029 00030 #include "arm_math.h" 00031 00032 /** 00033 * @ingroup groupMath 00034 */ 00035 00036 /** 00037 * @addtogroup scale 00038 * @{ 00039 */ 00040 00041 /** 00042 * @brief Multiplies a Q7 vector by a scalar. 00043 * @param[in] *pSrc points to the input vector 00044 * @param[in] scaleFract fractional portion of the scale value 00045 * @param[in] shift number of bits to shift the result by 00046 * @param[out] *pDst points to the output vector 00047 * @param[in] blockSize number of samples in the vector 00048 * @return none. 00049 * 00050 * <b>Scaling and Overflow Behavior:</b> 00051 * \par 00052 * The input data <code>*pSrc</code> and <code>scaleFract</code> are in 1.7 format. 00053 * These are multiplied to yield a 2.14 intermediate result and this is shifted with saturation to 1.7 format. 00054 */ 00055 00056 void arm_scale_q7( 00057 q7_t * pSrc, 00058 q7_t scaleFract, 00059 int8_t shift, 00060 q7_t * pDst, 00061 uint32_t blockSize) 00062 { 00063 int8_t kShift = 7 - shift; /* shift to apply after scaling */ 00064 uint32_t blkCnt; /* loop counter */ 00065 q7_t in1, in2, in3, in4, out1, out2, out3, out4; /* Temporary variables to store input & output */ 00066 00067 00068 /*loop Unrolling */ 00069 blkCnt = blockSize >> 2u; 00070 00071 00072 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00073 ** a second loop below computes the remaining 1 to 3 samples. */ 00074 while(blkCnt > 0u) 00075 { 00076 /* Reading 4 inputs from memory */ 00077 in1 = *pSrc++; 00078 in2 = *pSrc++; 00079 in3 = *pSrc++; 00080 in4 = *pSrc++; 00081 00082 /* C = A * scale */ 00083 /* Scale the inputs and then store the results in the temporary variables. */ 00084 out1 = (q7_t) (__SSAT(((in1) * scaleFract) >> kShift, 8)); 00085 out2 = (q7_t) (__SSAT(((in2) * scaleFract) >> kShift, 8)); 00086 out3 = (q7_t) (__SSAT(((in3) * scaleFract) >> kShift, 8)); 00087 out4 = (q7_t) (__SSAT(((in4) * scaleFract) >> kShift, 8)); 00088 00089 /* Packing the individual outputs into 32bit and storing in 00090 * destination buffer in single write */ 00091 *__SIMD32(pDst)++ = __PACKq7(out1, out2, out3, out4); 00092 00093 /* Decrement the loop counter */ 00094 blkCnt--; 00095 } 00096 00097 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00098 ** No loop unrolling is used. */ 00099 blkCnt = blockSize % 0x4u; 00100 00101 while(blkCnt > 0u) 00102 { 00103 /* C = A * scale */ 00104 /* Scale the input and then store the result in the destination buffer. */ 00105 *pDst++ = (q7_t) (__SSAT(((*pSrc++) * scaleFract) >> kShift, 8)); 00106 00107 /* Decrement the loop counter */ 00108 blkCnt--; 00109 } 00110 } 00111 00112 /** 00113 * @} end of scale group 00114 */
Generated on Tue Jul 12 2022 14:13:54 by
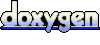