CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_rms_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_rms_q31.c 00009 * 00010 * Description: root mean square value of an array of Q31 type 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * ---------------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @addtogroup RMS 00031 * @{ 00032 */ 00033 00034 00035 /** 00036 * @brief Root Mean Square of the elements of a Q31 vector. 00037 * @param[in] *pSrc points to the input vector 00038 * @param[in] blockSize length of the input vector 00039 * @param[out] *pResult rms value returned here 00040 * @return none. 00041 * 00042 * @details 00043 * <b>Scaling and Overflow Behavior:</b> 00044 * 00045 *\par 00046 * The function is implemented using an internal 64-bit accumulator. 00047 * The input is represented in 1.31 format, and intermediate multiplication 00048 * yields a 2.62 format. 00049 * The accumulator maintains full precision of the intermediate multiplication results, 00050 * but provides only a single guard bit. 00051 * There is no saturation on intermediate additions. 00052 * If the accumulator overflows, it wraps around and distorts the result. 00053 * In order to avoid overflows completely, the input signal must be scaled down by 00054 * log2(blockSize) bits, as a total of blockSize additions are performed internally. 00055 * Finally, the 2.62 accumulator is right shifted by 31 bits to yield a 1.31 format value. 00056 * 00057 */ 00058 00059 void arm_rms_q31( 00060 q31_t * pSrc, 00061 uint32_t blockSize, 00062 q31_t * pResult) 00063 { 00064 q31_t *pIn1 = pSrc; /* SrcA pointer */ 00065 q63_t sum = 0; /* accumulator */ 00066 q31_t in; /* Temporary variable to store the input */ 00067 uint32_t blkCnt; /* loop counter */ 00068 00069 /*loop Unrolling */ 00070 blkCnt = blockSize >> 2u; 00071 00072 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00073 ** a second loop below computes the remaining 1 to 3 samples. */ 00074 while(blkCnt > 0u) 00075 { 00076 /* C = A[0] * A[0] + A[1] * A[1] + A[2] * A[2] + ... + A[blockSize-1] * A[blockSize-1] */ 00077 /* Compute sum of the squares and then store the result in a temporary variable, sum */ 00078 in = *pIn1++; 00079 sum += (q63_t) in *in; 00080 in = *pIn1++; 00081 sum += (q63_t) in *in; 00082 in = *pIn1++; 00083 sum += (q63_t) in *in; 00084 in = *pIn1++; 00085 sum += (q63_t) in *in; 00086 00087 /* Decrement the loop counter */ 00088 blkCnt--; 00089 } 00090 00091 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00092 ** No loop unrolling is used. */ 00093 blkCnt = blockSize % 0x4u; 00094 00095 while(blkCnt > 0u) 00096 { 00097 /* C = A[0] * A[0] + A[1] * A[1] + A[2] * A[2] + ... + A[blockSize-1] * A[blockSize-1] */ 00098 /* Compute sum of the squares and then store the results in a temporary variable, sum */ 00099 in = *pIn1++; 00100 sum += (q63_t) in *in; 00101 00102 /* Decrement the loop counter */ 00103 blkCnt--; 00104 } 00105 00106 /* Convert data in 2.62 to 1.31 by 31 right shifts */ 00107 sum = sum >> 31; 00108 00109 /* Compute Rms and store the result in the destination vector */ 00110 arm_sqrt_q31((q31_t) (sum / (int32_t) blockSize), pResult); 00111 } 00112 00113 /** 00114 * @} end of RMS group 00115 */
Generated on Tue Jul 12 2022 14:13:54 by
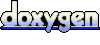