CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_pid_init_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_pid_init_f32.c 00009 * 00010 * Description: Floating-point PID Control initialization function 00011 * 00012 * 00013 * Target Processor: Cortex-M4/Cortex-M3 00014 * 00015 * Version 1.0.3 2010/11/29 00016 * Re-organized the CMSIS folders and updated documentation. 00017 * 00018 * Version 1.0.2 2010/11/11 00019 * Documentation updated. 00020 * 00021 * Version 1.0.1 2010/10/05 00022 * Production release and review comments incorporated. 00023 * 00024 * Version 1.0.0 2010/09/20 00025 * Production release and review comments incorporated. 00026 * ------------------------------------------------------------------- */ 00027 00028 #include "arm_math.h" 00029 00030 /** 00031 * @addtogroup PID 00032 * @{ 00033 */ 00034 00035 /** 00036 * @brief Initialization function for the floating-point PID Control. 00037 * @param[in,out] *S points to an instance of the PID structure. 00038 * @param[in] resetStateFlag flag to reset the state. 0 = no change in state & 1 = reset the state. 00039 * @return none. 00040 * \par Description: 00041 * \par 00042 * The <code>resetStateFlag</code> specifies whether to set state to zero or not. \n 00043 * The function computes the structure fields: <code>A0</code>, <code>A1</code> <code>A2</code> 00044 * using the proportional gain( \c Kp), integral gain( \c Ki) and derivative gain( \c Kd) 00045 * also sets the state variables to all zeros. 00046 */ 00047 00048 void arm_pid_init_f32( 00049 arm_pid_instance_f32 * S, 00050 int32_t resetStateFlag) 00051 { 00052 00053 /* Derived coefficient A0 */ 00054 S->A0 = S->Kp + S->Ki + S->Kd; 00055 00056 /* Derived coefficient A1 */ 00057 S->A1 = (-S->Kp) - ((float32_t) 2.0 * S->Kd); 00058 00059 /* Derived coefficient A2 */ 00060 S->A2 = S->Kd; 00061 00062 /* Check whether state needs reset or not */ 00063 if(resetStateFlag) 00064 { 00065 /* Clear the state buffer. The size will be always 3 samples */ 00066 memset(S->state, 0, 3u * sizeof(float32_t)); 00067 } 00068 00069 } 00070 00071 /** 00072 * @} end of PID group 00073 */
Generated on Tue Jul 12 2022 14:13:54 by
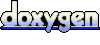