CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_offset_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_offset_f32.c 00009 * 00010 * Description: Floating-point vector offset. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.7 2010/06/10 00027 * Misra-C changes done 00028 * ---------------------------------------------------------------------------- */ 00029 00030 #include "arm_math.h" 00031 00032 /** 00033 * @ingroup groupMath 00034 */ 00035 00036 /** 00037 * @defgroup offset Vector Offset 00038 * 00039 * Adds a constant offset to each element of a vector. 00040 * 00041 * <pre> 00042 * pDst[n] = pSrc[n] + offset, 0 <= n < blockSize. 00043 * </pre> 00044 * 00045 * There are separate functions for floating-point, Q7, Q15, and Q31 data types. 00046 */ 00047 00048 /** 00049 * @addtogroup offset 00050 * @{ 00051 */ 00052 00053 /** 00054 * @brief Adds a constant offset to a floating-point vector. 00055 * @param *pSrc points to the input vector 00056 * @param offset is the offset to be added 00057 * @param *pDst points to the output vector 00058 * @param blockSize number of samples in the vector 00059 * @return none. 00060 */ 00061 00062 00063 void arm_offset_f32( 00064 float32_t * pSrc, 00065 float32_t offset, 00066 float32_t * pDst, 00067 uint32_t blockSize) 00068 { 00069 uint32_t blkCnt; /* loop counter */ 00070 00071 /*loop Unrolling */ 00072 blkCnt = blockSize >> 2u; 00073 00074 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00075 ** a second loop below computes the remaining 1 to 3 samples. */ 00076 while(blkCnt > 0u) 00077 { 00078 /* C = A + offset */ 00079 /* Add offset and then store the results in the destination buffer. */ 00080 *pDst++ = (*pSrc++) + offset; 00081 *pDst++ = (*pSrc++) + offset; 00082 *pDst++ = (*pSrc++) + offset; 00083 *pDst++ = (*pSrc++) + offset; 00084 00085 /* Decrement the loop counter */ 00086 blkCnt--; 00087 } 00088 00089 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00090 ** No loop unrolling is used. */ 00091 blkCnt = blockSize % 0x4u; 00092 00093 while(blkCnt > 0u) 00094 { 00095 /* C = A + offset */ 00096 /* Add offset and then store the result in the destination buffer. */ 00097 *pDst++ = (*pSrc++) + offset; 00098 00099 /* Decrement the loop counter */ 00100 blkCnt--; 00101 } 00102 } 00103 00104 /** 00105 * @} end of offset group 00106 */
Generated on Tue Jul 12 2022 14:13:54 by
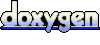