CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_mult_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mult_q31.c 00009 * 00010 * Description: Q31 vector multiplication. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupMath 00037 */ 00038 00039 /** 00040 * @addtogroup BasicMult 00041 * @{ 00042 */ 00043 00044 /** 00045 * @brief Q31 vector multiplication. 00046 * @param[in] *pSrcA points to the first input vector 00047 * @param[in] *pSrcB points to the second input vector 00048 * @param[out] *pDst points to the output vector 00049 * @param[in] blockSize number of samples in each vector 00050 * @return none. 00051 * 00052 * <b>Scaling and Overflow Behavior:</b> 00053 * \par 00054 * The function uses saturating arithmetic. 00055 * Results outside of the allowable Q31 range[0x80000000 0x7FFFFFFF] will be saturated. 00056 */ 00057 00058 void arm_mult_q31( 00059 q31_t * pSrcA, 00060 q31_t * pSrcB, 00061 q31_t * pDst, 00062 uint32_t blockSize) 00063 { 00064 uint32_t blkCnt; /* loop counters */ 00065 00066 /* loop Unrolling */ 00067 blkCnt = blockSize >> 2u; 00068 00069 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00070 ** a second loop below computes the remaining 1 to 3 samples. */ 00071 while(blkCnt > 0u) 00072 { 00073 /* C = A * B */ 00074 /* Multiply the inputs and then store the results in the destination buffer. */ 00075 *pDst++ = (q31_t) clip_q63_to_q31(((q63_t) (*pSrcA++) * (*pSrcB++)) >> 31); 00076 *pDst++ = (q31_t) clip_q63_to_q31(((q63_t) (*pSrcA++) * (*pSrcB++)) >> 31); 00077 *pDst++ = (q31_t) clip_q63_to_q31(((q63_t) (*pSrcA++) * (*pSrcB++)) >> 31); 00078 *pDst++ = (q31_t) clip_q63_to_q31(((q63_t) (*pSrcA++) * (*pSrcB++)) >> 31); 00079 00080 /* Decrement the blockSize loop counter */ 00081 blkCnt--; 00082 } 00083 00084 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00085 ** No loop unrolling is used. */ 00086 blkCnt = blockSize % 0x4u; 00087 00088 while(blkCnt > 0u) 00089 { 00090 /* C = A * B */ 00091 /* Multiply the inputs and then store the results in the destination buffer. */ 00092 *pDst++ = (q31_t) clip_q63_to_q31(((q63_t) (*pSrcA++) * (*pSrcB++)) >> 31); 00093 00094 /* Decrement the blockSize loop counter */ 00095 blkCnt--; 00096 } 00097 } 00098 00099 /** 00100 * @} end of BasicMult group 00101 */
Generated on Tue Jul 12 2022 14:13:54 by
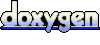