CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_min_q7.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_min_q7.c 00009 * 00010 * Description: Processing function for the Q7 Minimum. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * ---------------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupStats 00031 */ 00032 00033 /** 00034 * @addtogroup Min 00035 * @{ 00036 */ 00037 00038 00039 /** 00040 * @brief Minimum value of a Q7 vector. 00041 * @param[in] *pSrc points to the input vector 00042 * @param[in] blockSize length of the input vector 00043 * @param[out] *pResult minimum value returned here 00044 * @param[out] *pIndex index of minimum value returned here 00045 * @return none. 00046 * 00047 */ 00048 00049 void arm_min_q7( 00050 q7_t * pSrc, 00051 uint32_t blockSize, 00052 q7_t * pResult, 00053 uint32_t * pIndex) 00054 { 00055 q7_t minVal, minVal1, minVal2, res, x0, x1; /* Temporary variables to store the output value. */ 00056 uint32_t blkCnt, indx, index1, index2, index3, indxMod; /* loop counter */ 00057 00058 /* Initialise the index value to zero. */ 00059 indx = 0u; 00060 00061 /* Load first input value that act as reference value for comparision */ 00062 res = *pSrc++; 00063 00064 /* Loop over blockSize number of values */ 00065 blkCnt = (blockSize - 1u) >> 2u; 00066 00067 while(blkCnt > 0u) 00068 { 00069 indxMod = blockSize - (blkCnt * 4u); 00070 00071 /* Load two input values for comparision */ 00072 x0 = *pSrc++; 00073 x1 = *pSrc++; 00074 00075 if(x0 > x1) 00076 { 00077 /* Update the minimum value and its index */ 00078 minVal1 = x1; 00079 index1 = indxMod + 1u; 00080 } 00081 else 00082 { 00083 /* Update the minimum value and its index */ 00084 minVal1 = x0; 00085 index1 = indxMod; 00086 } 00087 00088 /* Load two input values for comparision */ 00089 x0 = *pSrc++; 00090 x1 = *pSrc++; 00091 00092 if(x0 > x1) 00093 { 00094 /* Update the minimum value and its index */ 00095 minVal2 = x1; 00096 index2 = indxMod + 3u; 00097 } 00098 else 00099 { 00100 /* Update the minimum value and its index */ 00101 minVal2 = x0; 00102 index2 = indxMod + 2u; 00103 } 00104 00105 if(minVal1 > minVal2) 00106 { 00107 /* Update the minimum value and its index */ 00108 minVal = minVal2; 00109 index3 = index2; 00110 } 00111 else 00112 { 00113 /* Update the minimum value and its index */ 00114 minVal = minVal1; 00115 index3 = index1; 00116 } 00117 00118 if(res > minVal) 00119 { 00120 /* Update the minimum value and its index */ 00121 res = minVal; 00122 indx = index3; 00123 } 00124 00125 /* Decrement the loop counter */ 00126 blkCnt--; 00127 00128 } 00129 00130 blkCnt = (blockSize - 1u) % 0x04u; 00131 00132 while(blkCnt > 0u) 00133 { 00134 /* Initialize minVal to the next consecutive values one by one */ 00135 minVal = *pSrc++; 00136 00137 /* compare for the minimum value */ 00138 if(res > minVal) 00139 { 00140 /* Update the minimum value and its index */ 00141 res = minVal; 00142 indx = blockSize - blkCnt; 00143 } 00144 00145 /* Decrement the loop counter */ 00146 blkCnt--; 00147 } 00148 00149 /* Store the minimum value and its index into destination pointers */ 00150 *pResult = res; 00151 *pIndex = indx; 00152 } 00153 00154 /** 00155 * @} end of Min group 00156 */
Generated on Tue Jul 12 2022 14:13:54 by
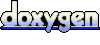