CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_min_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_min_q15.c 00009 * 00010 * Description: Minimum value of two Q15 arrays. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * ---------------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupStats 00031 */ 00032 00033 00034 /** 00035 * @addtogroup Min 00036 * @{ 00037 */ 00038 00039 00040 /** 00041 * @brief Minimum value of a Q15 vector. 00042 * @param[in] *pSrc points to the input vector 00043 * @param[in] blockSize length of the input vector 00044 * @param[out] *pResult minimum value returned here 00045 * @param[out] *pIndex index of minimum value returned here 00046 * @return none. 00047 * 00048 */ 00049 00050 void arm_min_q15( 00051 q15_t * pSrc, 00052 uint32_t blockSize, 00053 q15_t * pResult, 00054 uint32_t * pIndex) 00055 { 00056 q15_t minVal, out; /* Temporary variables to store the output value. */ 00057 uint32_t blkCnt, outIndex; /* loop counter */ 00058 00059 /* Initialise the index value to zero. */ 00060 outIndex = 0u; 00061 /* Load first input value that act as reference value for comparision */ 00062 out = *pSrc++; 00063 00064 /* Loop over blockSize number of values */ 00065 blkCnt = (blockSize - 1u); 00066 00067 do 00068 { 00069 /* Initialize minVal to the next consecutive values one by one */ 00070 minVal = *pSrc++; 00071 00072 /* compare for the minimum value */ 00073 if(out > minVal) 00074 { 00075 /* Update the minimum value and its index */ 00076 out = minVal; 00077 outIndex = blockSize - blkCnt; 00078 } 00079 00080 blkCnt--; 00081 00082 } while(blkCnt > 0u); 00083 00084 /* Store the minimum value and its index into destination pointers */ 00085 *pResult = out; 00086 *pIndex = outIndex; 00087 } 00088 00089 /** 00090 * @} end of Min group 00091 */
Generated on Tue Jul 12 2022 14:13:54 by
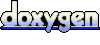