CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_max_q7.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_max_q7.c 00009 * 00010 * Description: Maximum value of a Q7 vector. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * ---------------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupStats 00031 */ 00032 00033 /** 00034 * @addtogroup Max 00035 * @{ 00036 */ 00037 00038 00039 /** 00040 * @brief Maximum value of a Q7 vector. 00041 * @param[in] *pSrc points to the input vector 00042 * @param[in] blockSize length of the input vector 00043 * @param[out] *pResult maximum value returned here 00044 * @param[out] *pIndex index of maximum value returned here 00045 * @return none. 00046 */ 00047 00048 void arm_max_q7( 00049 q7_t * pSrc, 00050 uint32_t blockSize, 00051 q7_t * pResult, 00052 uint32_t * pIndex) 00053 { 00054 q7_t res, maxVal, x0, x1, maxVal2, maxVal1; /* Temporary variables to store the output value. */ 00055 uint32_t blkCnt, index1, index2, index3, indx, indxMod; /* loop counter */ 00056 00057 /* Initialise the index value to zero. */ 00058 indx = 0u; 00059 00060 /* Load first input value that act as reference value for comparision */ 00061 res = *pSrc++; 00062 00063 /* Loop over blockSize number of values */ 00064 blkCnt = (blockSize - 1u) >> 2u; 00065 00066 while(blkCnt > 0u) 00067 { 00068 indxMod = blockSize - (blkCnt * 4u); 00069 00070 /* Load two input values for comparision */ 00071 x0 = *pSrc++; 00072 x1 = *pSrc++; 00073 00074 if(x0 < x1) 00075 { 00076 /* Update the minimum value and its index */ 00077 maxVal1 = x1; 00078 index1 = indxMod + 1u; 00079 } 00080 else 00081 { 00082 /* Update the minimum value and its index */ 00083 maxVal1 = x0; 00084 index1 = indxMod; 00085 } 00086 00087 /* Load two input values for comparision */ 00088 x0 = *pSrc++; 00089 x1 = *pSrc++; 00090 00091 if(x0 < x1) 00092 { 00093 /* Update the minimum value and its index */ 00094 maxVal2 = x1; 00095 index2 = indxMod + 3u; 00096 } 00097 else 00098 { 00099 /* Update the minimum value and its index */ 00100 maxVal2 = x0; 00101 index2 = indxMod + 2u; 00102 } 00103 00104 if(maxVal1 < maxVal2) 00105 { 00106 /* Update the minimum value and its index */ 00107 maxVal = maxVal2; 00108 index3 = index2; 00109 } 00110 else 00111 { 00112 /* Update the minimum value and its index */ 00113 maxVal = maxVal1; 00114 index3 = index1; 00115 } 00116 00117 if(res < maxVal) 00118 { 00119 /* Update the minimum value and its index */ 00120 res = maxVal; 00121 indx = index3; 00122 } 00123 00124 /* Decrement the loop counter */ 00125 blkCnt--; 00126 00127 } 00128 00129 blkCnt = (blockSize - 1u) % 0x04u; 00130 00131 while(blkCnt > 0u) 00132 { 00133 /* Initialize maxVal to the next consecutive values one by one */ 00134 maxVal = *pSrc++; 00135 00136 /* compare for the maximum value */ 00137 if(res < maxVal) 00138 { 00139 /* Update the maximum value and its index */ 00140 res = maxVal; 00141 indx = blockSize - blkCnt; 00142 } 00143 00144 /* Decrement the loop counter */ 00145 blkCnt--; 00146 } 00147 00148 /* Store the maximum value and its index into destination pointers */ 00149 *pResult = res; 00150 *pIndex = indx; 00151 } 00152 00153 /** 00154 * @} end of Max group 00155 */
Generated on Tue Jul 12 2022 14:13:54 by
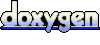