CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_mat_trans_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_trans_q31.c 00009 * 00010 * Description: Q31 matrix transpose. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupMatrix 00037 */ 00038 00039 /** 00040 * @addtogroup MatrixTrans 00041 * @{ 00042 */ 00043 00044 /* 00045 * @brief Q31 matrix transpose. 00046 * @param[in] *pSrc points to the input matrix 00047 * @param[out] *pDst points to the output matrix 00048 * @return The function returns either <code>ARM_MATH_SIZE_MISMATCH</code> 00049 * or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00050 */ 00051 00052 arm_status arm_mat_trans_q31( 00053 const arm_matrix_instance_q31 * pSrc, 00054 arm_matrix_instance_q31 * pDst) 00055 { 00056 q31_t *pIn = pSrc->pData; /* input data matrix pointer */ 00057 q31_t *pOut = pDst->pData; /* output data matrix pointer */ 00058 q31_t *px; /* Temporary output data matrix pointer */ 00059 uint16_t nRows = pSrc->numRows; /* number of nRows */ 00060 uint16_t nColumns = pSrc->numCols; /* number of nColumns */ 00061 uint16_t blkCnt, i = 0u, row = nRows; /* loop counters */ 00062 arm_status status; /* status of matrix transpose */ 00063 00064 00065 #ifdef ARM_MATH_MATRIX_CHECK 00066 /* Check for matrix mismatch condition */ 00067 if((pSrc->numRows != pDst->numCols) || (pSrc->numCols != pDst->numRows)) 00068 { 00069 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00070 status = ARM_MATH_SIZE_MISMATCH; 00071 } 00072 else 00073 #endif 00074 { 00075 /* Matrix transpose by exchanging the rows with columns */ 00076 /* row loop */ 00077 do 00078 { 00079 /* Apply loop unrolling and exchange the columns with row elements */ 00080 blkCnt = nColumns >> 2u; 00081 00082 /* The pointer px is set to starting address of the column being processed */ 00083 px = pOut + i; 00084 00085 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00086 ** a second loop below computes the remaining 1 to 3 samples. */ 00087 while(blkCnt > 0u) 00088 { 00089 /* Read and store the input element in the destination */ 00090 *px = *pIn++; 00091 00092 /* Update the pointer px to point to the next row of the transposed matrix */ 00093 px += nRows; 00094 00095 /* Read and store the input element in the destination */ 00096 *px = *pIn++; 00097 00098 /* Update the pointer px to point to the next row of the transposed matrix */ 00099 px += nRows; 00100 00101 /* Read and store the input element in the destination */ 00102 *px = *pIn++; 00103 00104 /* Update the pointer px to point to the next row of the transposed matrix */ 00105 px += nRows; 00106 00107 /* Read and store the input element in the destination */ 00108 *px = *pIn++; 00109 00110 /* Update the pointer px to point to the next row of the transposed matrix */ 00111 px += nRows; 00112 00113 /* Decrement the column loop counter */ 00114 blkCnt--; 00115 } 00116 00117 /* Perform matrix transpose for last 3 samples here. */ 00118 blkCnt = nColumns % 0x4u; 00119 00120 while(blkCnt > 0u) 00121 { 00122 /* Read and store the input element in the destination */ 00123 *px = *pIn++; 00124 00125 /* Update the pointer px to point to the next row of the transposed matrix */ 00126 px += nRows; 00127 00128 /* Decrement the column loop counter */ 00129 blkCnt--; 00130 } 00131 00132 i++; 00133 00134 /* Decrement the row loop counter */ 00135 row--; 00136 00137 } 00138 while(row > 0u); /* row loop end */ 00139 00140 /* set status as ARM_MATH_SUCCESS */ 00141 status = ARM_MATH_SUCCESS; 00142 } 00143 00144 /* Return to application */ 00145 return (status); 00146 } 00147 00148 /** 00149 * @} end of MatrixTrans group 00150 */
Generated on Tue Jul 12 2022 14:13:53 by
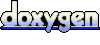