CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_mat_trans_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_trans_f32.c 00009 * 00010 * Description: Floating-point matrix transpose. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------- */ 00032 00033 /** 00034 * @defgroup MatrixTrans Matrix Transpose 00035 * 00036 * Tranposes a matrix. 00037 * Transposing an <code>M x N</code> matrix flips it around the center diagonal and results in an <code>N x M</code> matrix. 00038 * \image html MatrixTranspose.gif "Transpose of a 3 x 3 matrix" 00039 */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupMatrix 00045 */ 00046 00047 /** 00048 * @addtogroup MatrixTrans 00049 * @{ 00050 */ 00051 00052 /** 00053 * @brief Floating-point matrix transpose. 00054 * @param[in] *pSrc points to the input matrix 00055 * @param[out] *pDst points to the output matrix 00056 * @return The function returns either <code>ARM_MATH_SIZE_MISMATCH</code> 00057 * or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00058 */ 00059 00060 00061 arm_status arm_mat_trans_f32( 00062 const arm_matrix_instance_f32 * pSrc, 00063 arm_matrix_instance_f32 * pDst) 00064 { 00065 float32_t *pIn = pSrc->pData; /* input data matrix pointer */ 00066 float32_t *pOut = pDst->pData; /* output data matrix pointer */ 00067 float32_t *px; /* Temporary output data matrix pointer */ 00068 uint16_t nRows = pSrc->numRows; /* number of rows */ 00069 uint16_t nColumns = pSrc->numCols; /* number of columns */ 00070 uint16_t blkCnt, i = 0u, row = nRows; /* loop counters */ 00071 arm_status status; /* status of matrix transpose */ 00072 00073 00074 #ifdef ARM_MATH_MATRIX_CHECK 00075 /* Check for matrix mismatch condition */ 00076 if((pSrc->numRows != pDst->numCols) || (pSrc->numCols != pDst->numRows)) 00077 { 00078 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00079 status = ARM_MATH_SIZE_MISMATCH; 00080 } 00081 else 00082 #endif 00083 { 00084 /* Matrix transpose by exchanging the rows with columns */ 00085 /* row loop */ 00086 do 00087 { 00088 /* Loop Unrolling */ 00089 blkCnt = nColumns >> 2; 00090 00091 /* The pointer px is set to starting address of the column being processed */ 00092 px = pOut + i; 00093 00094 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00095 ** a second loop below computes the remaining 1 to 3 samples. */ 00096 while(blkCnt > 0u) /* column loop */ 00097 { 00098 /* Read and store the input element in the destination */ 00099 *px = *pIn++; 00100 00101 /* Update the pointer px to point to the next row of the transposed matrix */ 00102 px += nRows; 00103 00104 /* Read and store the input element in the destination */ 00105 *px = *pIn++; 00106 00107 /* Update the pointer px to point to the next row of the transposed matrix */ 00108 px += nRows; 00109 00110 /* Read and store the input element in the destination */ 00111 *px = *pIn++; 00112 00113 /* Update the pointer px to point to the next row of the transposed matrix */ 00114 px += nRows; 00115 00116 /* Read and store the input element in the destination */ 00117 *px = *pIn++; 00118 00119 /* Update the pointer px to point to the next row of the transposed matrix */ 00120 px += nRows; 00121 00122 /* Decrement the column loop counter */ 00123 blkCnt--; 00124 } 00125 00126 /* Perform matrix transpose for last 3 samples here. */ 00127 blkCnt = nColumns % 0x4u; 00128 00129 while(blkCnt > 0u) 00130 { 00131 /* Read and store the input element in the destination */ 00132 *px = *pIn++; 00133 00134 /* Update the pointer px to point to the next row of the transposed matrix */ 00135 px += nRows; 00136 00137 /* Decrement the column loop counter */ 00138 blkCnt--; 00139 } 00140 00141 i++; 00142 00143 /* Decrement the row loop counter */ 00144 row--; 00145 00146 } while(row > 0u); /* row loop end */ 00147 00148 /* Set status as ARM_MATH_SUCCESS */ 00149 status = ARM_MATH_SUCCESS; 00150 } 00151 00152 /* Return to application */ 00153 return (status); 00154 } 00155 00156 /** 00157 * @} end of MatrixTrans group 00158 */
Generated on Tue Jul 12 2022 14:13:53 by
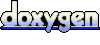