CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_mat_scale_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_scale_f32.c 00009 * 00010 * Description: Multiplies a floating-point matrix by a scalar. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupMatrix 00037 */ 00038 00039 /** 00040 * @defgroup MatrixScale Matrix Scale 00041 * 00042 * Multiplies a matrix by a scalar. This is accomplished by multiplying each element in the 00043 * matrix by the scalar. For example: 00044 * \image html MatrixScale.gif "Matrix Scaling of a 3 x 3 matrix" 00045 * 00046 * The function checks to make sure that the input and output matrices are of the same size. 00047 * 00048 * In the fixed-point Q15 and Q31 functions, <code>scale</code> is represented by 00049 * a fractional multiplication <code>scaleFract</code> and an arithmetic shift <code>shift</code>. 00050 * The shift allows the gain of the scaling operation to exceed 1.0. 00051 * The overall scale factor applied to the fixed-point data is 00052 * <pre> 00053 * scale = scaleFract * 2^shift. 00054 * </pre> 00055 */ 00056 00057 /** 00058 * @addtogroup MatrixScale 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief Floating-point matrix scaling. 00064 * @param[in] *pSrc points to input matrix structure 00065 * @param[in] scale scale factor to be applied 00066 * @param[out] *pDst points to output matrix structure 00067 * @return The function returns either <code>ARM_MATH_SIZE_MISMATCH</code> 00068 * or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00069 * 00070 */ 00071 00072 arm_status arm_mat_scale_f32( 00073 const arm_matrix_instance_f32 * pSrc, 00074 float32_t scale, 00075 arm_matrix_instance_f32 * pDst) 00076 { 00077 float32_t *pIn = pSrc->pData; /* input data matrix pointer */ 00078 float32_t *pOut = pDst->pData; /* output data matrix pointer */ 00079 uint32_t numSamples; /* total number of elements in the matrix */ 00080 uint32_t blkCnt; /* loop counters */ 00081 arm_status status; /* status of matrix scaling */ 00082 00083 #ifdef ARM_MATH_MATRIX_CHECK 00084 /* Check for matrix mismatch condition */ 00085 if((pSrc->numRows != pDst->numRows) || (pSrc->numCols != pDst->numCols)) 00086 { 00087 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00088 status = ARM_MATH_SIZE_MISMATCH; 00089 } 00090 else 00091 #endif 00092 { 00093 /* Total number of samples in the input matrix */ 00094 numSamples = (uint32_t) pSrc->numRows * pSrc->numCols; 00095 00096 /* Loop Unrolling */ 00097 blkCnt = numSamples >> 2; 00098 00099 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00100 ** a second loop below computes the remaining 1 to 3 samples. */ 00101 while(blkCnt > 0u) 00102 { 00103 /* C(m,n) = A(m,n) * scale */ 00104 /* Scaling and results are stored in the destination buffer. */ 00105 *pOut++ = (*pIn++) * scale; 00106 *pOut++ = (*pIn++) * scale; 00107 *pOut++ = (*pIn++) * scale; 00108 *pOut++ = (*pIn++) * scale; 00109 00110 /* Decrement the numSamples loop counter */ 00111 blkCnt--; 00112 } 00113 00114 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00115 ** No loop unrolling is used. */ 00116 blkCnt = numSamples % 0x4u; 00117 00118 while(blkCnt > 0u) 00119 { 00120 /* C(m,n) = A(m,n) * scale */ 00121 /* The results are stored in the destination buffer. */ 00122 *pOut++ = (*pIn++) * scale; 00123 00124 /* Decrement the loop counter */ 00125 blkCnt--; 00126 } 00127 00128 /* Set status as ARM_MATH_SUCCESS */ 00129 status = ARM_MATH_SUCCESS; 00130 } 00131 00132 /* Return to application */ 00133 return (status); 00134 } 00135 00136 /** 00137 * @} end of MatrixScale group 00138 */
Generated on Tue Jul 12 2022 14:13:53 by
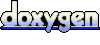