CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_mat_mult_fast_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_mult_fast_q31.c 00009 * 00010 * Description: Q31 matrix multiplication (fast variant). 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * -------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupMatrix 00031 */ 00032 00033 /** 00034 * @addtogroup MatrixMult 00035 * @{ 00036 */ 00037 00038 /** 00039 * @brief Q31 matrix multiplication (fast variant) 00040 * @param[in] *pSrcA points to the first input matrix structure 00041 * @param[in] *pSrcB points to the second input matrix structure 00042 * @param[out] *pDst points to output matrix structure 00043 * @return The function returns either 00044 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00045 * 00046 * @details 00047 * <b>Scaling and Overflow Behavior:</b> 00048 * 00049 * \par 00050 * The difference between the function arm_mat_mult_q31() and this fast variant is that 00051 * the fast variant use a 32-bit rather than a 64-bit accumulator. 00052 * The result of each 1.31 x 1.31 multiplication is truncated to 00053 * 2.30 format. These intermediate results are accumulated in a 32-bit register in 2.30 00054 * format. Finally, the accumulator is saturated and converted to a 1.31 result. 00055 * 00056 * \par 00057 * The fast version has the same overflow behavior as the standard version but provides 00058 * less precision since it discards the low 32 bits of each multiplication result. 00059 * In order to avoid overflows completely the input signals must be scaled down. 00060 * Scale down one of the input matrices by log2(numColsA) bits to 00061 * avoid overflows, as a total of numColsA additions are computed internally for each 00062 * output element. 00063 * 00064 * \par 00065 * See <code>arm_mat_mult_q31()</code> for a slower implementation of this function 00066 * which uses 64-bit accumulation to provide higher precision. 00067 */ 00068 00069 arm_status arm_mat_mult_fast_q31( 00070 const arm_matrix_instance_q31 * pSrcA, 00071 const arm_matrix_instance_q31 * pSrcB, 00072 arm_matrix_instance_q31 * pDst) 00073 { 00074 q31_t *pIn1 = pSrcA->pData; /* input data matrix pointer A */ 00075 q31_t *pIn2 = pSrcB->pData; /* input data matrix pointer B */ 00076 q31_t *pInA = pSrcA->pData; /* input data matrix pointer A */ 00077 // q31_t *pSrcB = pSrcB->pData; /* input data matrix pointer B */ 00078 q31_t *pOut = pDst->pData; /* output data matrix pointer */ 00079 q31_t *px; /* Temporary output data matrix pointer */ 00080 q31_t sum; /* Accumulator */ 00081 uint16_t numRowsA = pSrcA->numRows; /* number of rows of input matrix A */ 00082 uint16_t numColsB = pSrcB->numCols; /* number of columns of input matrix B */ 00083 uint16_t numColsA = pSrcA->numCols; /* number of columns of input matrix A */ 00084 uint16_t col, i = 0u, j, row = numRowsA, colCnt; /* loop counters */ 00085 arm_status status; /* status of matrix multiplication */ 00086 00087 00088 #ifdef ARM_MATH_MATRIX_CHECK 00089 /* Check for matrix mismatch condition */ 00090 if((pSrcA->numCols != pSrcB->numRows) || 00091 (pSrcA->numRows != pDst->numRows) || (pSrcB->numCols != pDst->numCols)) 00092 { 00093 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00094 status = ARM_MATH_SIZE_MISMATCH; 00095 } 00096 else 00097 #endif 00098 { 00099 /* The following loop performs the dot-product of each row in pSrcA with each column in pSrcB */ 00100 /* row loop */ 00101 do 00102 { 00103 /* Output pointer is set to starting address of the row being processed */ 00104 px = pOut + i; 00105 00106 /* For every row wise process, the column loop counter is to be initiated */ 00107 col = numColsB; 00108 00109 /* For every row wise process, the pIn2 pointer is set 00110 ** to the starting address of the pSrcB data */ 00111 pIn2 = pSrcB->pData; 00112 00113 j = 0u; 00114 00115 /* column loop */ 00116 do 00117 { 00118 /* Set the variable sum, that acts as accumulator, to zero */ 00119 sum = 0; 00120 00121 /* Initiate the pointer pIn1 to point to the starting address of pInA */ 00122 pIn1 = pInA; 00123 00124 /* Apply loop unrolling and compute 4 MACs simultaneously. */ 00125 colCnt = numColsA >> 2; 00126 00127 00128 /* matrix multiplication */ 00129 while(colCnt > 0u) 00130 { 00131 /* c(m,n) = a(1,1)*b(1,1) + a(1,2) * b(2,1) + .... + a(m,p)*b(p,n) */ 00132 /* Perform the multiply-accumulates */ 00133 sum = (q31_t) ((((q63_t) sum << 32) + 00134 ((q63_t) * pIn1++ * (*pIn2))) >> 32); 00135 pIn2 += numColsB; 00136 sum = (q31_t) ((((q63_t) sum << 32) + 00137 ((q63_t) * pIn1++ * (*pIn2))) >> 32); 00138 pIn2 += numColsB; 00139 sum = (q31_t) ((((q63_t) sum << 32) + 00140 ((q63_t) * pIn1++ * (*pIn2))) >> 32); 00141 pIn2 += numColsB; 00142 sum = (q31_t) ((((q63_t) sum << 32) + 00143 ((q63_t) * pIn1++ * (*pIn2))) >> 32); 00144 pIn2 += numColsB; 00145 00146 /* Decrement the loop counter */ 00147 colCnt--; 00148 } 00149 00150 /* If the columns of pSrcA is not a multiple of 4, compute any remaining output samples here. 00151 ** No loop unrolling is used. */ 00152 colCnt = numColsA % 0x4u; 00153 00154 while(colCnt > 0u) 00155 { 00156 /* c(m,n) = a(1,1)*b(1,1) + a(1,2) * b(2,1) + .... + a(m,p)*b(p,n) */ 00157 /* Perform the multiply-accumulates */ 00158 sum = (q31_t) ((((q63_t) sum << 32) + 00159 ((q63_t) * pIn1++ * (*pIn2))) >> 32); 00160 pIn2 += numColsB; 00161 00162 /* Decrement the loop counter */ 00163 colCnt--; 00164 } 00165 00166 /* Convert the result from 2.30 to 1.31 format and store in destination buffer */ 00167 *px++ = sum << 1; 00168 00169 /* Update the pointer pIn2 to point to the starting address of the next column */ 00170 j++; 00171 pIn2 = pSrcB->pData + j; 00172 00173 /* Decrement the column loop counter */ 00174 col--; 00175 00176 } while(col > 0u); 00177 00178 /* Update the pointer pInA to point to the starting address of the next row */ 00179 i = i + numColsB; 00180 pInA = pInA + numColsA; 00181 00182 /* Decrement the row loop counter */ 00183 row--; 00184 00185 } while(row > 0u); 00186 00187 /* set status as ARM_MATH_SUCCESS */ 00188 status = ARM_MATH_SUCCESS; 00189 } 00190 /* Return to application */ 00191 return (status); 00192 } 00193 00194 /** 00195 * @} end of MatrixMult group 00196 */
Generated on Tue Jul 12 2022 14:13:53 by
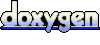