CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_mat_init_f32.c
00001 /* ---------------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_init_f32.c 00009 * 00010 * Description: Floating-point matrix initialization. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupMatrix 00037 */ 00038 00039 /** 00040 * @defgroup MatrixInit Matrix Initialization 00041 * 00042 * Initializes the underlying matrix data structure. 00043 * The functions set the <code>numRows</code>, 00044 * <code>numCols</code>, and <code>pData</code> fields 00045 * of the matrix data structure. 00046 */ 00047 00048 /** 00049 * @addtogroup MatrixInit 00050 * @{ 00051 */ 00052 00053 /** 00054 * @brief Floating-point matrix initialization. 00055 * @param[in,out] *S points to an instance of the floating-point matrix structure. 00056 * @param[in] nRows number of rows in the matrix. 00057 * @param[in] nColumns number of columns in the matrix. 00058 * @param[in] *pData points to the matrix data array. 00059 * @return none 00060 */ 00061 00062 void arm_mat_init_f32( 00063 arm_matrix_instance_f32 * S, 00064 uint16_t nRows, 00065 uint16_t nColumns, 00066 float32_t * pData) 00067 { 00068 /* Assign Number of Rows */ 00069 S->numRows = nRows; 00070 00071 /* Assign Number of Columns */ 00072 S->numCols = nColumns; 00073 00074 /* Assign Data pointer */ 00075 S->pData = pData; 00076 } 00077 00078 /** 00079 * @} end of MatrixInit group 00080 */
Generated on Tue Jul 12 2022 14:13:53 by
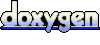