CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_lms_norm_init_q15.c
00001 /*----------------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_lms_norm_init_q15.c 00009 * 00010 * Description: Q15 NLMS initialization function. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated 00025 * 00026 * Version 0.0.7 2010/06/10 00027 * Misra-C changes done 00028 * ---------------------------------------------------------------------------*/ 00029 00030 #include "arm_math.h" 00031 #include "arm_common_tables.h" 00032 00033 /** 00034 * @addtogroup LMS_NORM 00035 * @{ 00036 */ 00037 00038 /** 00039 * @brief Initialization function for Q15 normalized LMS filter. 00040 * @param[in] *S points to an instance of the Q15 normalized LMS filter structure. 00041 * @param[in] numTaps number of filter coefficients. 00042 * @param[in] *pCoeffs points to coefficient buffer. 00043 * @param[in] *pState points to state buffer. 00044 * @param[in] mu step size that controls filter coefficient updates. 00045 * @param[in] blockSize number of samples to process. 00046 * @param[in] postShift bit shift applied to coefficients. 00047 * @return none. 00048 * 00049 * <b>Description:</b> 00050 * \par 00051 * <code>pCoeffs</code> points to the array of filter coefficients stored in time reversed order: 00052 * <pre> 00053 * {b[numTaps-1], b[numTaps-2], b[N-2], ..., b[1], b[0]} 00054 * </pre> 00055 * The initial filter coefficients serve as a starting point for the adaptive filter. 00056 * <code>pState</code> points to the array of state variables and size of array is 00057 * <code>numTaps+blockSize-1</code> samples, where <code>blockSize</code> is the number of input samples processed 00058 * by each call to <code>arm_lms_norm_q15()</code>. 00059 */ 00060 00061 void arm_lms_norm_init_q15( 00062 arm_lms_norm_instance_q15 * S, 00063 uint16_t numTaps, 00064 q15_t * pCoeffs, 00065 q15_t * pState, 00066 q15_t mu, 00067 uint32_t blockSize, 00068 uint8_t postShift) 00069 { 00070 /* Assign filter taps */ 00071 S->numTaps = numTaps; 00072 00073 /* Assign coefficient pointer */ 00074 S->pCoeffs = pCoeffs; 00075 00076 /* Clear state buffer and size is always blockSize + numTaps - 1 */ 00077 memset(pState, 0, (numTaps + (blockSize - 1u)) * sizeof(q15_t)); 00078 00079 /* Assign post Shift value applied to coefficients */ 00080 S->postShift = postShift; 00081 00082 /* Assign state pointer */ 00083 S->pState = pState; 00084 00085 /* Assign Step size value */ 00086 S->mu = mu; 00087 00088 /* Initialize reciprocal pointer table */ 00089 S->recipTable = (q15_t*)armRecipTableQ15; 00090 00091 /* Initialise Energy to zero */ 00092 S->energy = 0; 00093 00094 /* Initialise x0 to zero */ 00095 S->x0 = 0; 00096 00097 } 00098 00099 /** 00100 * @} end of LMS_NORM group 00101 */
Generated on Tue Jul 12 2022 14:13:53 by
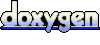