CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_fir_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_fir_q31.c 00009 * 00010 * Description: Q31 FIR filter processing function. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupFilters 00037 */ 00038 00039 /** 00040 * @addtogroup FIR 00041 * @{ 00042 */ 00043 00044 /** 00045 * @param[in] *S points to an instance of the Q31 FIR filter structure. 00046 * @param[in] *pSrc points to the block of input data. 00047 * @param[out] *pDst points to the block of output data. 00048 * @param[in] blockSize number of samples to process per call. 00049 * @return none. 00050 * 00051 * @details 00052 * <b>Scaling and Overflow Behavior:</b> 00053 * \par 00054 * The function is implemented using an internal 64-bit accumulator. 00055 * The accumulator has a 2.62 format and maintains full precision of the intermediate multiplication results but provides only a single guard bit. 00056 * Thus, if the accumulator result overflows it wraps around rather than clip. 00057 * In order to avoid overflows completely the input signal must be scaled down by log2(numTaps) bits. 00058 * After all multiply-accumulates are performed, the 2.62 accumulator is truncated to 1.32 format and then saturated to 1.31 format. 00059 * 00060 * \par 00061 * Refer to the function <code>arm_fir_fast_q31()</code> for a faster but less precise implementation of this filter. 00062 */ 00063 00064 void arm_fir_q31( 00065 const arm_fir_instance_q31 * S, 00066 q31_t * pSrc, 00067 q31_t * pDst, 00068 uint32_t blockSize) 00069 { 00070 q31_t *pState = S->pState; /* State pointer */ 00071 q31_t *pCoeffs = S->pCoeffs; /* Coefficient pointer */ 00072 q31_t *pStateCurnt; /* Points to the current sample of the state */ 00073 q31_t x0, x1, x2, x3; /* Temporary variables to hold state */ 00074 q31_t c0; /* Temporary variable to hold coefficient value */ 00075 q31_t *px; /* Temporary pointer for state */ 00076 q31_t *pb; /* Temporary pointer for coefficient buffer */ 00077 q63_t acc0, acc1, acc2, acc3; /* Accumulators */ 00078 uint32_t numTaps = S->numTaps; /* Number of filter coefficients in the filter */ 00079 uint32_t i, tapCnt, blkCnt; /* Loop counters */ 00080 00081 /* S->pState points to state array which contains previous frame (numTaps - 1) samples */ 00082 /* pStateCurnt points to the location where the new input data should be written */ 00083 pStateCurnt = &(S->pState[(numTaps - 1u)]); 00084 00085 /* Apply loop unrolling and compute 4 output values simultaneously. 00086 * The variables acc0 ... acc3 hold output values that are being computed: 00087 * 00088 * acc0 = b[numTaps-1] * x[n-numTaps-1] + b[numTaps-2] * x[n-numTaps-2] + b[numTaps-3] * x[n-numTaps-3] +...+ b[0] * x[0] 00089 * acc1 = b[numTaps-1] * x[n-numTaps] + b[numTaps-2] * x[n-numTaps-1] + b[numTaps-3] * x[n-numTaps-2] +...+ b[0] * x[1] 00090 * acc2 = b[numTaps-1] * x[n-numTaps+1] + b[numTaps-2] * x[n-numTaps] + b[numTaps-3] * x[n-numTaps-1] +...+ b[0] * x[2] 00091 * acc3 = b[numTaps-1] * x[n-numTaps+2] + b[numTaps-2] * x[n-numTaps+1] + b[numTaps-3] * x[n-numTaps] +...+ b[0] * x[3] 00092 */ 00093 blkCnt = blockSize >> 2; 00094 00095 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00096 ** a second loop below computes the remaining 1 to 3 samples. */ 00097 while(blkCnt > 0u) 00098 { 00099 /* Copy four new input samples into the state buffer */ 00100 *pStateCurnt++ = *pSrc++; 00101 *pStateCurnt++ = *pSrc++; 00102 *pStateCurnt++ = *pSrc++; 00103 *pStateCurnt++ = *pSrc++; 00104 00105 /* Set all accumulators to zero */ 00106 acc0 = 0; 00107 acc1 = 0; 00108 acc2 = 0; 00109 acc3 = 0; 00110 00111 /* Initialize state pointer */ 00112 px = pState; 00113 00114 /* Initialize coefficient pointer */ 00115 pb = pCoeffs; 00116 00117 /* Read the first three samples from the state buffer: 00118 * x[n-numTaps], x[n-numTaps-1], x[n-numTaps-2] */ 00119 x0 = *(px++); 00120 x1 = *(px++); 00121 x2 = *(px++); 00122 00123 /* Loop unrolling. Process 4 taps at a time. */ 00124 tapCnt = numTaps >> 2; 00125 i = tapCnt; 00126 00127 while(i > 0u) 00128 { 00129 /* Read the b[numTaps] coefficient */ 00130 c0 = *(pb++); 00131 00132 /* Read x[n-numTaps-3] sample */ 00133 x3 = *(px++); 00134 00135 /* acc0 += b[numTaps] * x[n-numTaps] */ 00136 acc0 += ((q63_t) x0 * c0); 00137 00138 /* acc1 += b[numTaps] * x[n-numTaps-1] */ 00139 acc1 += ((q63_t) x1 * c0); 00140 00141 /* acc2 += b[numTaps] * x[n-numTaps-2] */ 00142 acc2 += ((q63_t) x2 * c0); 00143 00144 /* acc3 += b[numTaps] * x[n-numTaps-3] */ 00145 acc3 += ((q63_t) x3 * c0); 00146 00147 /* Read the b[numTaps-1] coefficient */ 00148 c0 = *(pb++); 00149 00150 /* Read x[n-numTaps-4] sample */ 00151 x0 = *(px++); 00152 00153 /* Perform the multiply-accumulates */ 00154 acc0 += ((q63_t) x1 * c0); 00155 acc1 += ((q63_t) x2 * c0); 00156 acc2 += ((q63_t) x3 * c0); 00157 acc3 += ((q63_t) x0 * c0); 00158 00159 /* Read the b[numTaps-2] coefficient */ 00160 c0 = *(pb++); 00161 00162 /* Read x[n-numTaps-5] sample */ 00163 x1 = *(px++); 00164 00165 /* Perform the multiply-accumulates */ 00166 acc0 += ((q63_t) x2 * c0); 00167 acc1 += ((q63_t) x3 * c0); 00168 acc2 += ((q63_t) x0 * c0); 00169 acc3 += ((q63_t) x1 * c0); 00170 /* Read the b[numTaps-3] coefficients */ 00171 c0 = *(pb++); 00172 00173 /* Read x[n-numTaps-6] sample */ 00174 x2 = *(px++); 00175 00176 /* Perform the multiply-accumulates */ 00177 acc0 += ((q63_t) x3 * c0); 00178 acc1 += ((q63_t) x0 * c0); 00179 acc2 += ((q63_t) x1 * c0); 00180 acc3 += ((q63_t) x2 * c0); 00181 i--; 00182 } 00183 00184 /* If the filter length is not a multiple of 4, compute the remaining filter taps */ 00185 00186 i = numTaps - (tapCnt * 4u); 00187 while(i > 0u) 00188 { 00189 /* Read coefficients */ 00190 c0 = *(pb++); 00191 00192 /* Fetch 1 state variable */ 00193 x3 = *(px++); 00194 00195 /* Perform the multiply-accumulates */ 00196 acc0 += ((q63_t) x0 * c0); 00197 acc1 += ((q63_t) x1 * c0); 00198 acc2 += ((q63_t) x2 * c0); 00199 acc3 += ((q63_t) x3 * c0); 00200 00201 /* Reuse the present sample states for next sample */ 00202 x0 = x1; 00203 x1 = x2; 00204 x2 = x3; 00205 00206 /* Decrement the loop counter */ 00207 i--; 00208 } 00209 00210 /* Advance the state pointer by 4 to process the next group of 4 samples */ 00211 pState = pState + 4; 00212 00213 /* The results in the 4 accumulators are in 2.30 format. Convert to 1.31 00214 ** Then store the 4 outputs in the destination buffer. */ 00215 *pDst++ = (q31_t) (acc0 >> 31u); 00216 *pDst++ = (q31_t) (acc1 >> 31u); 00217 *pDst++ = (q31_t) (acc2 >> 31u); 00218 *pDst++ = (q31_t) (acc3 >> 31u); 00219 00220 /* Decrement the samples loop counter */ 00221 blkCnt--; 00222 } 00223 00224 00225 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00226 ** No loop unrolling is used. */ 00227 blkCnt = blockSize % 4u; 00228 00229 while(blkCnt > 0u) 00230 { 00231 /* Copy one sample at a time into state buffer */ 00232 *pStateCurnt++ = *pSrc++; 00233 00234 /* Set the accumulator to zero */ 00235 acc0 = 0; 00236 00237 /* Initialize state pointer */ 00238 px = pState; 00239 00240 /* Initialize Coefficient pointer */ 00241 pb = (pCoeffs); 00242 00243 i = numTaps; 00244 00245 /* Perform the multiply-accumulates */ 00246 do 00247 { 00248 acc0 += (q63_t) * (px++) * (*(pb++)); 00249 i--; 00250 } while(i > 0u); 00251 00252 /* The result is in 2.62 format. Convert to 1.31 00253 ** Then store the output in the destination buffer. */ 00254 *pDst++ = (q31_t) (acc0 >> 31u); 00255 00256 /* Advance state pointer by 1 for the next sample */ 00257 pState = pState + 1; 00258 00259 /* Decrement the samples loop counter */ 00260 blkCnt--; 00261 } 00262 00263 /* Processing is complete. 00264 ** Now copy the last numTaps - 1 samples to the satrt of the state buffer. 00265 ** This prepares the state buffer for the next function call. */ 00266 00267 /* Points to the start of the state buffer */ 00268 pStateCurnt = S->pState; 00269 00270 tapCnt = (numTaps - 1u) >> 2u; 00271 00272 /* copy data */ 00273 while(tapCnt > 0u) 00274 { 00275 *pStateCurnt++ = *pState++; 00276 *pStateCurnt++ = *pState++; 00277 *pStateCurnt++ = *pState++; 00278 *pStateCurnt++ = *pState++; 00279 00280 /* Decrement the loop counter */ 00281 tapCnt--; 00282 } 00283 00284 /* Calculate remaining number of copies */ 00285 tapCnt = (numTaps - 1u) % 0x4u; 00286 00287 /* Copy the remaining q31_t data */ 00288 while(tapCnt > 0u) 00289 { 00290 *pStateCurnt++ = *pState++; 00291 00292 /* Decrement the loop counter */ 00293 tapCnt--; 00294 } 00295 00296 } 00297 00298 /** 00299 * @} end of FIR group 00300 */
Generated on Tue Jul 12 2022 14:13:53 by
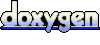