CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_fir_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_fir_f32.c 00009 * 00010 * Description: Floating-point FIR filter processing function. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 00035 /** 00036 * @ingroup groupFilters 00037 */ 00038 00039 /** 00040 * @defgroup FIR Finite Impulse Response (FIR) Filters 00041 * 00042 * This set of functions implements Finite Impulse Response (FIR) filters 00043 * for Q7, Q15, Q31, and floating-point data types. Fast versions of Q15 and Q31 are also provided. 00044 * The functions operate on blocks of input and output data and each call to the function processes 00045 * <code>blockSize</code> samples through the filter. <code>pSrc</code> and 00046 * <code>pDst</code> points to input and output arrays containing <code>blockSize</code> values. 00047 * 00048 * \par Algorithm: 00049 * The FIR filter algorithm is based upon a sequence of multiply-accumulate (MAC) operations. 00050 * Each filter coefficient <code>b[n]</code> is multiplied by a state variable which equals a previous input sample <code>x[n]</code>. 00051 * <pre> 00052 * y[n] = b[0] * x[n] + b[1] * x[n-1] + b[2] * x[n-2] + ...+ b[numTaps-1] * x[n-numTaps+1] 00053 * </pre> 00054 * \par 00055 * \image html FIR.gif "Finite Impulse Response filter" 00056 * \par 00057 * <code>pCoeffs</code> points to a coefficient array of size <code>numTaps</code>. 00058 * Coefficients are stored in time reversed order. 00059 * \par 00060 * <pre> 00061 * {b[numTaps-1], b[numTaps-2], b[N-2], ..., b[1], b[0]} 00062 * </pre> 00063 * \par 00064 * <code>pState</code> points to a state array of size <code>numTaps + blockSize - 1</code>. 00065 * Samples in the state buffer are stored in the following order. 00066 * \par 00067 * <pre> 00068 * {x[n-numTaps+1], x[n-numTaps], x[n-numTaps-1], x[n-numTaps-2]....x[0], x[1], ..., x[blockSize-1]} 00069 * </pre> 00070 * \par 00071 * Note that the length of the state buffer exceeds the length of the coefficient array by <code>blockSize-1</code>. 00072 * The increased state buffer length allows circular addressing, which is traditionally used in the FIR filters, 00073 * to be avoided and yields a significant speed improvement. 00074 * The state variables are updated after each block of data is processed; the coefficients are untouched. 00075 * \par Instance Structure 00076 * The coefficients and state variables for a filter are stored together in an instance data structure. 00077 * A separate instance structure must be defined for each filter. 00078 * Coefficient arrays may be shared among several instances while state variable arrays cannot be shared. 00079 * There are separate instance structure declarations for each of the 4 supported data types. 00080 * 00081 * \par Initialization Functions 00082 * There is also an associated initialization function for each data type. 00083 * The initialization function performs the following operations: 00084 * - Sets the values of the internal structure fields. 00085 * - Zeros out the values in the state buffer. 00086 * 00087 * \par 00088 * Use of the initialization function is optional. 00089 * However, if the initialization function is used, then the instance structure cannot be placed into a const data section. 00090 * To place an instance structure into a const data section, the instance structure must be manually initialized. 00091 * Set the values in the state buffer to zeros before static initialization. 00092 * The code below statically initializes each of the 4 different data type filter instance structures 00093 * <pre> 00094 *arm_fir_instance_f32 S = {numTaps, pState, pCoeffs}; 00095 *arm_fir_instance_q31 S = {numTaps, pState, pCoeffs}; 00096 *arm_fir_instance_q15 S = {numTaps, pState, pCoeffs}; 00097 *arm_fir_instance_q7 S = {numTaps, pState, pCoeffs}; 00098 * </pre> 00099 * 00100 * where <code>numTaps</code> is the number of filter coefficients in the filter; <code>pState</code> is the address of the state buffer; 00101 * <code>pCoeffs</code> is the address of the coefficient buffer. 00102 * 00103 * \par Fixed-Point Behavior 00104 * Care must be taken when using the fixed-point versions of the FIR filter functions. 00105 * In particular, the overflow and saturation behavior of the accumulator used in each function must be considered. 00106 * Refer to the function specific documentation below for usage guidelines. 00107 */ 00108 00109 /** 00110 * @addtogroup FIR 00111 * @{ 00112 */ 00113 00114 /** 00115 * 00116 * @param[in] *S points to an instance of the floating-point FIR filter structure. 00117 * @param[in] *pSrc points to the block of input data. 00118 * @param[out] *pDst points to the block of output data. 00119 * @param[in] blockSize number of samples to process per call. 00120 * @return none. 00121 * 00122 */ 00123 00124 void arm_fir_f32( 00125 const arm_fir_instance_f32 * S, 00126 float32_t * pSrc, 00127 float32_t * pDst, 00128 uint32_t blockSize) 00129 { 00130 float32_t *pState = S->pState; /* State pointer */ 00131 float32_t *pCoeffs = S->pCoeffs; /* Coefficient pointer */ 00132 float32_t *pStateCurnt; /* Points to the current sample of the state */ 00133 float32_t *px, *pb; /* Temporary pointers for state and coefficient buffers */ 00134 float32_t acc0, acc1, acc2, acc3; /* Accumulators */ 00135 float32_t x0, x1, x2, x3, c0; /* Temporary variables to hold state and coefficient values */ 00136 uint32_t numTaps = S->numTaps; /* Number of filter coefficients in the filter */ 00137 uint32_t i, tapCnt, blkCnt; /* Loop counters */ 00138 00139 /* S->pState points to state array which contains previous frame (numTaps - 1) samples */ 00140 /* pStateCurnt points to the location where the new input data should be written */ 00141 pStateCurnt = &(S->pState[(numTaps - 1u)]); 00142 00143 /* Apply loop unrolling and compute 4 output values simultaneously. 00144 * The variables acc0 ... acc3 hold output values that are being computed: 00145 * 00146 * acc0 = b[numTaps-1] * x[n-numTaps-1] + b[numTaps-2] * x[n-numTaps-2] + b[numTaps-3] * x[n-numTaps-3] +...+ b[0] * x[0] 00147 * acc1 = b[numTaps-1] * x[n-numTaps] + b[numTaps-2] * x[n-numTaps-1] + b[numTaps-3] * x[n-numTaps-2] +...+ b[0] * x[1] 00148 * acc2 = b[numTaps-1] * x[n-numTaps+1] + b[numTaps-2] * x[n-numTaps] + b[numTaps-3] * x[n-numTaps-1] +...+ b[0] * x[2] 00149 * acc3 = b[numTaps-1] * x[n-numTaps+2] + b[numTaps-2] * x[n-numTaps+1] + b[numTaps-3] * x[n-numTaps] +...+ b[0] * x[3] 00150 */ 00151 blkCnt = blockSize >> 2; 00152 00153 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00154 ** a second loop below computes the remaining 1 to 3 samples. */ 00155 while(blkCnt > 0u) 00156 { 00157 /* Copy four new input samples into the state buffer */ 00158 *pStateCurnt++ = *pSrc++; 00159 *pStateCurnt++ = *pSrc++; 00160 *pStateCurnt++ = *pSrc++; 00161 *pStateCurnt++ = *pSrc++; 00162 00163 /* Set all accumulators to zero */ 00164 acc0 = 0.0f; 00165 acc1 = 0.0f; 00166 acc2 = 0.0f; 00167 acc3 = 0.0f; 00168 00169 /* Initialize state pointer */ 00170 px = pState; 00171 00172 /* Initialize coeff pointer */ 00173 pb = (pCoeffs); 00174 00175 /* Read the first three samples from the state buffer: x[n-numTaps], x[n-numTaps-1], x[n-numTaps-2] */ 00176 x0 = *px++; 00177 x1 = *px++; 00178 x2 = *px++; 00179 00180 /* Loop unrolling. Process 4 taps at a time. */ 00181 tapCnt = numTaps >> 2u; 00182 00183 /* Loop over the number of taps. Unroll by a factor of 4. 00184 ** Repeat until we've computed numTaps-4 coefficients. */ 00185 while(tapCnt > 0u) 00186 { 00187 /* Read the b[numTaps-1] coefficient */ 00188 c0 = *(pb++); 00189 00190 /* Read x[n-numTaps-3] sample */ 00191 x3 = *(px++); 00192 00193 /* acc0 += b[numTaps-1] * x[n-numTaps] */ 00194 acc0 += x0 * c0; 00195 00196 /* acc1 += b[numTaps-1] * x[n-numTaps-1] */ 00197 acc1 += x1 * c0; 00198 00199 /* acc2 += b[numTaps-1] * x[n-numTaps-2] */ 00200 acc2 += x2 * c0; 00201 00202 /* acc3 += b[numTaps-1] * x[n-numTaps-3] */ 00203 acc3 += x3 * c0; 00204 00205 /* Read the b[numTaps-2] coefficient */ 00206 c0 = *(pb++); 00207 00208 /* Read x[n-numTaps-4] sample */ 00209 x0 = *(px++); 00210 00211 /* Perform the multiply-accumulate */ 00212 acc0 += x1 * c0; 00213 acc1 += x2 * c0; 00214 acc2 += x3 * c0; 00215 acc3 += x0 * c0; 00216 00217 /* Read the b[numTaps-3] coefficient */ 00218 c0 = *(pb++); 00219 00220 /* Read x[n-numTaps-5] sample */ 00221 x1 = *(px++); 00222 00223 /* Perform the multiply-accumulates */ 00224 acc0 += x2 * c0; 00225 acc1 += x3 * c0; 00226 acc2 += x0 * c0; 00227 acc3 += x1 * c0; 00228 00229 /* Read the b[numTaps-4] coefficient */ 00230 c0 = *(pb++); 00231 00232 /* Read x[n-numTaps-6] sample */ 00233 x2 = *(px++); 00234 00235 /* Perform the multiply-accumulates */ 00236 acc0 += x3 * c0; 00237 acc1 += x0 * c0; 00238 acc2 += x1 * c0; 00239 acc3 += x2 * c0; 00240 00241 tapCnt--; 00242 } 00243 00244 /* If the filter length is not a multiple of 4, compute the remaining filter taps */ 00245 tapCnt = numTaps % 0x4u; 00246 00247 while(tapCnt > 0u) 00248 { 00249 /* Read coefficients */ 00250 c0 = *(pb++); 00251 00252 /* Fetch 1 state variable */ 00253 x3 = *(px++); 00254 00255 /* Perform the multiply-accumulates */ 00256 acc0 += x0 * c0; 00257 acc1 += x1 * c0; 00258 acc2 += x2 * c0; 00259 acc3 += x3 * c0; 00260 00261 /* Reuse the present sample states for next sample */ 00262 x0 = x1; 00263 x1 = x2; 00264 x2 = x3; 00265 00266 /* Decrement the loop counter */ 00267 tapCnt--; 00268 } 00269 00270 /* Advance the state pointer by 4 to process the next group of 4 samples */ 00271 pState = pState + 4; 00272 00273 /* The results in the 4 accumulators, store in the destination buffer. */ 00274 *pDst++ = acc0; 00275 *pDst++ = acc1; 00276 *pDst++ = acc2; 00277 *pDst++ = acc3; 00278 00279 blkCnt--; 00280 } 00281 00282 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00283 ** No loop unrolling is used. */ 00284 blkCnt = blockSize % 0x4u; 00285 00286 while(blkCnt > 0u) 00287 { 00288 /* Copy one sample at a time into state buffer */ 00289 *pStateCurnt++ = *pSrc++; 00290 00291 /* Set the accumulator to zero */ 00292 acc0 = 0.0f; 00293 00294 /* Initialize state pointer */ 00295 px = pState; 00296 00297 /* Initialize Coefficient pointer */ 00298 pb = (pCoeffs); 00299 00300 i = numTaps; 00301 00302 /* Perform the multiply-accumulates */ 00303 do 00304 { 00305 acc0 += *px++ * *pb++; 00306 i--; 00307 00308 } while(i > 0u); 00309 00310 /* The result is store in the destination buffer. */ 00311 *pDst++ = acc0; 00312 00313 /* Advance state pointer by 1 for the next sample */ 00314 pState = pState + 1; 00315 00316 blkCnt--; 00317 } 00318 00319 /* Processing is complete. 00320 ** Now copy the last numTaps - 1 samples to the satrt of the state buffer. 00321 ** This prepares the state buffer for the next function call. */ 00322 00323 /* Points to the start of the state buffer */ 00324 pStateCurnt = S->pState; 00325 00326 tapCnt = (numTaps - 1u) >> 2u; 00327 00328 /* copy data */ 00329 while(tapCnt > 0u) 00330 { 00331 *pStateCurnt++ = *pState++; 00332 *pStateCurnt++ = *pState++; 00333 *pStateCurnt++ = *pState++; 00334 *pStateCurnt++ = *pState++; 00335 00336 /* Decrement the loop counter */ 00337 tapCnt--; 00338 } 00339 00340 /* Calculate remaining number of copies */ 00341 tapCnt = (numTaps - 1u) % 0x4u; 00342 00343 /* Copy the remaining q31_t data */ 00344 while(tapCnt > 0u) 00345 { 00346 *pStateCurnt++ = *pState++; 00347 00348 /* Decrement the loop counter */ 00349 tapCnt--; 00350 } 00351 } 00352 00353 /** 00354 * @} end of FIR group 00355 */
Generated on Tue Jul 12 2022 14:13:53 by
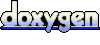