CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_cmplx_mult_cmplx_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_cmplx_mult_cmplx_q15.c 00009 * 00010 * Description: Q15 complex-by-complex multiplication 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * -------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupCmplxMath 00031 */ 00032 00033 /** 00034 * @addtogroup CmplxByCmplxMult 00035 * @{ 00036 */ 00037 00038 /** 00039 * @brief Q15 complex-by-complex multiplication 00040 * @param[in] *pSrcA points to the first input vector 00041 * @param[in] *pSrcB points to the second input vector 00042 * @param[out] *pDst points to the output vector 00043 * @param[in] numSamples number of complex samples in each vector 00044 * @return none. 00045 * 00046 * <b>Scaling and Overflow Behavior:</b> 00047 * \par 00048 * The function implements 1.15 by 1.15 multiplications and finally output is converted into 3.13 format. 00049 */ 00050 00051 void arm_cmplx_mult_cmplx_q15( 00052 q15_t * pSrcA, 00053 q15_t * pSrcB, 00054 q15_t * pDst, 00055 uint32_t numSamples) 00056 { 00057 q15_t a, b, c, d; /* Temporary variables to store real and imaginary values */ 00058 uint32_t blkCnt; /* loop counters */ 00059 00060 /* loop Unrolling */ 00061 blkCnt = numSamples >> 2u; 00062 00063 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00064 ** a second loop below computes the remaining 1 to 3 samples. */ 00065 while(blkCnt > 0u) 00066 { 00067 /* C[2 * i] = A[2 * i] * B[2 * i] - A[2 * i + 1] * B[2 * i + 1]. */ 00068 /* C[2 * i + 1] = A[2 * i] * B[2 * i + 1] + A[2 * i + 1] * B[2 * i]. */ 00069 a = *pSrcA++; 00070 b = *pSrcA++; 00071 c = *pSrcB++; 00072 d = *pSrcB++; 00073 00074 /* store the result in 3.13 format in the destination buffer. */ 00075 *pDst++ = 00076 (q15_t) (q31_t) (((q31_t) a * c) >> 17) - (((q31_t) b * d) >> 17); 00077 /* store the result in 3.13 format in the destination buffer. */ 00078 *pDst++ = 00079 (q15_t) (q31_t) (((q31_t) a * d) >> 17) + (((q31_t) b * c) >> 17); 00080 00081 a = *pSrcA++; 00082 b = *pSrcA++; 00083 c = *pSrcB++; 00084 d = *pSrcB++; 00085 00086 /* store the result in 3.13 format in the destination buffer. */ 00087 *pDst++ = 00088 (q15_t) (q31_t) (((q31_t) a * c) >> 17) - (((q31_t) b * d) >> 17); 00089 /* store the result in 3.13 format in the destination buffer. */ 00090 *pDst++ = 00091 (q15_t) (q31_t) (((q31_t) a * d) >> 17) + (((q31_t) b * c) >> 17); 00092 00093 a = *pSrcA++; 00094 b = *pSrcA++; 00095 c = *pSrcB++; 00096 d = *pSrcB++; 00097 00098 /* store the result in 3.13 format in the destination buffer. */ 00099 *pDst++ = 00100 (q15_t) (q31_t) (((q31_t) a * c) >> 17) - (((q31_t) b * d) >> 17); 00101 /* store the result in 3.13 format in the destination buffer. */ 00102 *pDst++ = 00103 (q15_t) (q31_t) (((q31_t) a * d) >> 17) + (((q31_t) b * c) >> 17); 00104 00105 a = *pSrcA++; 00106 b = *pSrcA++; 00107 c = *pSrcB++; 00108 d = *pSrcB++; 00109 00110 /* store the result in 3.13 format in the destination buffer. */ 00111 *pDst++ = 00112 (q15_t) (q31_t) (((q31_t) a * c) >> 17) - (((q31_t) b * d) >> 17); 00113 /* store the result in 3.13 format in the destination buffer. */ 00114 *pDst++ = 00115 (q15_t) (q31_t) (((q31_t) a * d) >> 17) + (((q31_t) b * c) >> 17); 00116 00117 /* Decrement the blockSize loop counter */ 00118 blkCnt--; 00119 } 00120 00121 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00122 ** No loop unrolling is used. */ 00123 blkCnt = numSamples % 0x4u; 00124 00125 while(blkCnt > 0u) 00126 { 00127 /* C[2 * i] = A[2 * i] * B[2 * i] - A[2 * i + 1] * B[2 * i + 1]. */ 00128 /* C[2 * i + 1] = A[2 * i] * B[2 * i + 1] + A[2 * i + 1] * B[2 * i]. */ 00129 a = *pSrcA++; 00130 b = *pSrcA++; 00131 c = *pSrcB++; 00132 d = *pSrcB++; 00133 00134 /* store the result in 3.13 format in the destination buffer. */ 00135 *pDst++ = 00136 (q15_t) (q31_t) (((q31_t) a * c) >> 17) - (((q31_t) b * d) >> 17); 00137 /* store the result in 3.13 format in the destination buffer. */ 00138 *pDst++ = 00139 (q15_t) (q31_t) (((q31_t) a * d) >> 17) + (((q31_t) b * c) >> 17); 00140 00141 /* Decrement the blockSize loop counter */ 00142 blkCnt--; 00143 } 00144 } 00145 00146 /** 00147 * @} end of CmplxByCmplxMult group 00148 */
Generated on Tue Jul 12 2022 14:13:52 by
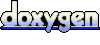