CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_cmplx_mult_cmplx_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_cmplx_mult_cmplx_f32.c 00009 * 00010 * Description: Floating-point complex-by-complex multiplication 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * -------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupCmplxMath 00031 */ 00032 00033 /** 00034 * @defgroup CmplxByCmplxMult Complex-by-Complex Multiplication 00035 * 00036 * Multiplies a complex vector by another complex vector and generates a complex result. 00037 * The data in the complex arrays is stored in an interleaved fashion 00038 * (real, imag, real, imag, ...). 00039 * The parameter <code>numSamples</code> represents the number of complex 00040 * samples processed. The complex arrays have a total of <code>2*numSamples</code> 00041 * real values. 00042 * 00043 * The underlying algorithm is used: 00044 * 00045 * <pre> 00046 * for(n=0; n<numSamples; n++) { 00047 * pDst[(2*n)+0] = pSrcA[(2*n)+0] * pSrcB[(2*n)+0] - pSrcA[(2*n)+1] * pSrcB[(2*n)+1]; 00048 * pDst[(2*n)+1] = pSrcA[(2*n)+0] * pSrcB[(2*n)+1] + pSrcA[(2*n)+1] * pSrcB[(2*n)+0]; 00049 * } 00050 * </pre> 00051 * 00052 * There are separate functions for floating-point, Q15, and Q31 data types. 00053 */ 00054 00055 /** 00056 * @addtogroup CmplxByCmplxMult 00057 * @{ 00058 */ 00059 00060 00061 /** 00062 * @brief Floating-point complex-by-complex multiplication 00063 * @param[in] *pSrcA points to the first input vector 00064 * @param[in] *pSrcB points to the second input vector 00065 * @param[out] *pDst points to the output vector 00066 * @param[in] numSamples number of complex samples in each vector 00067 * @return none. 00068 */ 00069 00070 void arm_cmplx_mult_cmplx_f32( 00071 float32_t * pSrcA, 00072 float32_t * pSrcB, 00073 float32_t * pDst, 00074 uint32_t numSamples) 00075 { 00076 float32_t a, b, c, d; /* Temporary variables to store real and imaginary values */ 00077 uint32_t blkCnt; /* loop counters */ 00078 00079 /* loop Unrolling */ 00080 blkCnt = numSamples >> 2u; 00081 00082 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00083 ** a second loop below computes the remaining 1 to 3 samples. */ 00084 while(blkCnt > 0u) 00085 { 00086 /* C[2 * i] = A[2 * i] * B[2 * i] - A[2 * i + 1] * B[2 * i + 1]. */ 00087 /* C[2 * i + 1] = A[2 * i] * B[2 * i + 1] + A[2 * i + 1] * B[2 * i]. */ 00088 a = *pSrcA++; 00089 b = *pSrcA++; 00090 c = *pSrcB++; 00091 d = *pSrcB++; 00092 00093 /* store the result in the destination buffer. */ 00094 *pDst++ = (a * c) - (b * d); 00095 *pDst++ = (a * d) + (b * c); 00096 00097 a = *pSrcA++; 00098 b = *pSrcA++; 00099 c = *pSrcB++; 00100 d = *pSrcB++; 00101 00102 *pDst++ = (a * c) - (b * d); 00103 *pDst++ = (a * d) + (b * c); 00104 00105 a = *pSrcA++; 00106 b = *pSrcA++; 00107 c = *pSrcB++; 00108 d = *pSrcB++; 00109 00110 *pDst++ = (a * c) - (b * d); 00111 *pDst++ = (a * d) + (b * c); 00112 00113 a = *pSrcA++; 00114 b = *pSrcA++; 00115 c = *pSrcB++; 00116 d = *pSrcB++; 00117 00118 *pDst++ = (a * c) - (b * d); 00119 *pDst++ = (a * d) + (b * c); 00120 00121 /* Decrement the numSamples loop counter */ 00122 blkCnt--; 00123 } 00124 00125 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00126 ** No loop unrolling is used. */ 00127 blkCnt = numSamples % 0x4u; 00128 00129 while(blkCnt > 0u) 00130 { 00131 /* C[2 * i] = A[2 * i] * B[2 * i] - A[2 * i + 1] * B[2 * i + 1]. */ 00132 /* C[2 * i + 1] = A[2 * i] * B[2 * i + 1] + A[2 * i + 1] * B[2 * i]. */ 00133 a = *pSrcA++; 00134 b = *pSrcA++; 00135 c = *pSrcB++; 00136 d = *pSrcB++; 00137 00138 /* store the result in the destination buffer. */ 00139 *pDst++ = (a * c) - (b * d); 00140 *pDst++ = (a * d) + (b * c); 00141 00142 /* Decrement the numSamples loop counter */ 00143 blkCnt--; 00144 } 00145 } 00146 00147 /** 00148 * @} end of CmplxByCmplxMult group 00149 */
Generated on Tue Jul 12 2022 14:13:52 by
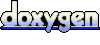