CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_cmplx_mag_squared_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_cmplx_mag_squared_q31.c 00009 * 00010 * Description: Q31 complex magnitude squared. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * ---------------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupCmplxMath 00031 */ 00032 00033 /** 00034 * @addtogroup cmplx_mag_squared 00035 * @{ 00036 */ 00037 00038 00039 /** 00040 * @brief Q31 complex magnitude squared 00041 * @param *pSrc points to the complex input vector 00042 * @param *pDst points to the real output vector 00043 * @param numSamples number of complex samples in the input vector 00044 * @return none. 00045 * 00046 * <b>Scaling and Overflow Behavior:</b> 00047 * \par 00048 * The function implements 1.31 by 1.31 multiplications and finally output is converted into 3.29 format. 00049 * Input down scaling is not required. 00050 */ 00051 00052 void arm_cmplx_mag_squared_q31( 00053 q31_t * pSrc, 00054 q31_t * pDst, 00055 uint32_t numSamples) 00056 { 00057 q31_t real, imag; /* Temporary variables to store real and imaginary values */ 00058 q31_t acc0, acc1; /* Accumulators */ 00059 uint32_t blkCnt; /* loop counter */ 00060 00061 /* loop Unrolling */ 00062 blkCnt = numSamples >> 2u; 00063 00064 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00065 ** a second loop below computes the remaining 1 to 3 samples. */ 00066 while(blkCnt > 0u) 00067 { 00068 /* C[0] = (A[0] * A[0] + A[1] * A[1]) */ 00069 real = *pSrc++; 00070 imag = *pSrc++; 00071 acc0 = (q31_t) (((q63_t) real * real) >> 33); 00072 acc1 = (q31_t) (((q63_t) imag * imag) >> 33); 00073 /* store the result in 3.29 format in the destination buffer. */ 00074 *pDst++ = acc0 + acc1; 00075 00076 real = *pSrc++; 00077 imag = *pSrc++; 00078 acc0 = (q31_t) (((q63_t) real * real) >> 33); 00079 acc1 = (q31_t) (((q63_t) imag * imag) >> 33); 00080 /* store the result in 3.29 format in the destination buffer. */ 00081 *pDst++ = acc0 + acc1; 00082 00083 real = *pSrc++; 00084 imag = *pSrc++; 00085 acc0 = (q31_t) (((q63_t) real * real) >> 33); 00086 acc1 = (q31_t) (((q63_t) imag * imag) >> 33); 00087 /* store the result in 3.29 format in the destination buffer. */ 00088 *pDst++ = acc0 + acc1; 00089 00090 real = *pSrc++; 00091 imag = *pSrc++; 00092 acc0 = (q31_t) (((q63_t) real * real) >> 33); 00093 acc1 = (q31_t) (((q63_t) imag * imag) >> 33); 00094 /* store the result in 3.29 format in the destination buffer. */ 00095 *pDst++ = acc0 + acc1; 00096 00097 /* Decrement the loop counter */ 00098 blkCnt--; 00099 } 00100 00101 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00102 ** No loop unrolling is used. */ 00103 blkCnt = numSamples % 0x4u; 00104 00105 while(blkCnt > 0u) 00106 { 00107 /* C[0] = (A[0] * A[0] + A[1] * A[1]) */ 00108 real = *pSrc++; 00109 imag = *pSrc++; 00110 acc0 = (q31_t) (((q63_t) real * real) >> 33); 00111 acc1 = (q31_t) (((q63_t) imag * imag) >> 33); 00112 /* store the result in 3.29 format in the destination buffer. */ 00113 *pDst++ = acc0 + acc1; 00114 00115 /* Decrement the loop counter */ 00116 blkCnt--; 00117 } 00118 } 00119 00120 /** 00121 * @} end of cmplx_mag_squared group 00122 */
Generated on Tue Jul 12 2022 14:13:52 by
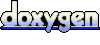