CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_cmplx_dot_prod_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_cmplx_dot_prod_q15.c 00009 * 00010 * Description: Processing function for the Q15 Complex Dot product 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * -------------------------------------------------------------------- */ 00026 00027 #include "arm_math.h" 00028 00029 /** 00030 * @ingroup groupCmplxMath 00031 */ 00032 00033 /** 00034 * @addtogroup cmplx_dot_prod 00035 * @{ 00036 */ 00037 00038 /** 00039 * @brief Q15 complex dot product 00040 * @param *pSrcA points to the first input vector 00041 * @param *pSrcB points to the second input vector 00042 * @param numSamples number of complex samples in each vector 00043 * @param *realResult real part of the result returned here 00044 * @param *imagResult imaginary part of the result returned here 00045 * @return none. 00046 * 00047 * <b>Scaling and Overflow Behavior:</b> 00048 * \par 00049 * The function is implemented using an internal 64-bit accumulator. 00050 * The intermediate 1.15 by 1.15 multiplications are performed with full precision and yield a 2.30 result. 00051 * These are accumulated in a 64-bit accumulator with 34.30 precision. 00052 * As a final step, the accumulators are converted to 8.24 format. 00053 * The return results <code>realResult</code> and <code>imagResult</code> are in 8.24 format. 00054 */ 00055 00056 void arm_cmplx_dot_prod_q15( 00057 q15_t * pSrcA, 00058 q15_t * pSrcB, 00059 uint32_t numSamples, 00060 q31_t * realResult, 00061 q31_t * imagResult) 00062 { 00063 q63_t real_sum = 0, imag_sum = 0; /* Temporary result storage */ 00064 uint32_t blkCnt; /* loop counter */ 00065 00066 00067 /*loop Unrolling */ 00068 blkCnt = numSamples >> 2u; 00069 00070 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00071 ** a second loop below computes the remaining 1 to 3 samples. */ 00072 while(blkCnt > 0u) 00073 { 00074 /* CReal = A[0]* B[0] + A[2]* B[2] + A[4]* B[4] + .....+ A[numSamples-2]* B[numSamples-2] */ 00075 real_sum += ((q31_t) * pSrcA++ * *pSrcB++); 00076 00077 /* CImag = A[1]* B[1] + A[3]* B[3] + A[5]* B[5] + .....+ A[numSamples-1]* B[numSamples-1] */ 00078 imag_sum += ((q31_t) * pSrcA++ * *pSrcB++); 00079 00080 real_sum += ((q31_t) * pSrcA++ * *pSrcB++); 00081 imag_sum += ((q31_t) * pSrcA++ * *pSrcB++); 00082 00083 real_sum += ((q31_t) * pSrcA++ * *pSrcB++); 00084 imag_sum += ((q31_t) * pSrcA++ * *pSrcB++); 00085 00086 real_sum += ((q31_t) * pSrcA++ * *pSrcB++); 00087 imag_sum += ((q31_t) * pSrcA++ * *pSrcB++); 00088 00089 /* Decrement the loop counter */ 00090 blkCnt--; 00091 } 00092 00093 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00094 ** No loop unrolling is used. */ 00095 blkCnt = numSamples % 0x4u; 00096 00097 while(blkCnt > 0u) 00098 { 00099 /* CReal = A[0]* B[0] + A[2]* B[2] + A[4]* B[4] + .....+ A[numSamples-2]* B[numSamples-2] */ 00100 real_sum += ((q31_t) * pSrcA++ * *pSrcB++); 00101 /* CImag = A[1]* B[1] + A[3]* B[3] + A[5]* B[5] + .....+ A[numSamples-1]* B[numSamples-1] */ 00102 imag_sum += ((q31_t) * pSrcA++ * *pSrcB++); 00103 00104 /* Decrement the loop counter */ 00105 blkCnt--; 00106 } 00107 00108 /* Store the real and imaginary results in 8.24 format */ 00109 /* Convert real data in 34.30 to 8.24 by 6 right shifts */ 00110 *realResult = (q31_t) (real_sum) >> 6; 00111 /* Convert imaginary data in 34.30 to 8.24 by 6 right shifts */ 00112 *imagResult = (q31_t) (imag_sum) >> 6; 00113 } 00114 00115 /** 00116 * @} end of cmplx_dot_prod group 00117 */
Generated on Tue Jul 12 2022 14:13:52 by
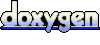