CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_cfft_radix4_init_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_cfft_radix4_init_q31.c 00009 * 00010 * Description: Radix-4 Decimation in Frequency Q31 FFT & IFFT initialization function 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.5 2010/04/26 00027 * incorporated review comments and updated with latest CMSIS layer 00028 * 00029 * Version 0.0.3 2010/03/10 00030 * Initial version 00031 * -------------------------------------------------------------------- */ 00032 00033 #include "arm_math.h" 00034 #include "arm_common_tables.h" 00035 00036 /** 00037 * @ingroup groupTransforms 00038 */ 00039 00040 /** 00041 * @addtogroup CFFT_CIFFT 00042 * @{ 00043 */ 00044 00045 /* 00046 * @brief Twiddle factors Table 00047 */ 00048 00049 /** 00050 * \par 00051 * Example code for Q31 Twiddle factors Generation:: 00052 * \par 00053 * <pre>for(i = 0; i< N; i++) 00054 * { 00055 * twiddleCoefQ31[2*i]= cos(i * 2*PI/(float)N); 00056 * twiddleCoefQ31[2*i+1]= sin(i * 2*PI/(float)N); 00057 * } </pre> 00058 * \par 00059 * where N = 1024 and PI = 3.14159265358979 00060 * \par 00061 * Cos and Sin values are interleaved fashion 00062 * \par 00063 * Convert Floating point to Q31(Fixed point 1.31): 00064 * round(twiddleCoefQ31(i) * pow(2, 31)) 00065 * 00066 */ 00067 00068 static const q31_t twiddleCoefQ31 [2048] = { 00069 0x7fffffff, 0x0, 0x7fff6216, 0xc90f88, 0x7ffd885a, 0x1921d20, 0x7ffa72d1, 00070 0x25b26d7, 00071 0x7ff62182, 0x3242abf, 0x7ff09478, 0x3ed26e6, 0x7fe9cbc0, 0x4b6195d, 00072 0x7fe1c76b, 0x57f0035, 00073 0x7fd8878e, 0x647d97c, 0x7fce0c3e, 0x710a345, 0x7fc25596, 0x7d95b9e, 00074 0x7fb563b3, 0x8a2009a, 00075 0x7fa736b4, 0x96a9049, 0x7f97cebd, 0xa3308bd, 0x7f872bf3, 0xafb6805, 00076 0x7f754e80, 0xbc3ac35, 00077 0x7f62368f, 0xc8bd35e, 0x7f4de451, 0xd53db92, 0x7f3857f6, 0xe1bc2e4, 00078 0x7f2191b4, 0xee38766, 00079 0x7f0991c4, 0xfab272b, 0x7ef05860, 0x1072a048, 0x7ed5e5c6, 0x1139f0cf, 00080 0x7eba3a39, 0x120116d5, 00081 0x7e9d55fc, 0x12c8106f, 0x7e7f3957, 0x138edbb1, 0x7e5fe493, 0x145576b1, 00082 0x7e3f57ff, 0x151bdf86, 00083 0x7e1d93ea, 0x15e21445, 0x7dfa98a8, 0x16a81305, 0x7dd6668f, 0x176dd9de, 00084 0x7db0fdf8, 0x183366e9, 00085 0x7d8a5f40, 0x18f8b83c, 0x7d628ac6, 0x19bdcbf3, 0x7d3980ec, 0x1a82a026, 00086 0x7d0f4218, 0x1b4732ef, 00087 0x7ce3ceb2, 0x1c0b826a, 0x7cb72724, 0x1ccf8cb3, 0x7c894bde, 0x1d934fe5, 00088 0x7c5a3d50, 0x1e56ca1e, 00089 0x7c29fbee, 0x1f19f97b, 0x7bf88830, 0x1fdcdc1b, 0x7bc5e290, 0x209f701c, 00090 0x7b920b89, 0x2161b3a0, 00091 0x7b5d039e, 0x2223a4c5, 0x7b26cb4f, 0x22e541af, 0x7aef6323, 0x23a6887f, 00092 0x7ab6cba4, 0x24677758, 00093 0x7a7d055b, 0x25280c5e, 0x7a4210d8, 0x25e845b6, 0x7a05eead, 0x26a82186, 00094 0x79c89f6e, 0x27679df4, 00095 0x798a23b1, 0x2826b928, 0x794a7c12, 0x28e5714b, 0x7909a92d, 0x29a3c485, 00096 0x78c7aba2, 0x2a61b101, 00097 0x78848414, 0x2b1f34eb, 0x78403329, 0x2bdc4e6f, 0x77fab989, 0x2c98fbba, 00098 0x77b417df, 0x2d553afc, 00099 0x776c4edb, 0x2e110a62, 0x77235f2d, 0x2ecc681e, 0x76d94989, 0x2f875262, 00100 0x768e0ea6, 0x3041c761, 00101 0x7641af3d, 0x30fbc54d, 0x75f42c0b, 0x31b54a5e, 0x75a585cf, 0x326e54c7, 00102 0x7555bd4c, 0x3326e2c3, 00103 0x7504d345, 0x33def287, 0x74b2c884, 0x34968250, 0x745f9dd1, 0x354d9057, 00104 0x740b53fb, 0x36041ad9, 00105 0x73b5ebd1, 0x36ba2014, 0x735f6626, 0x376f9e46, 0x7307c3d0, 0x382493b0, 00106 0x72af05a7, 0x38d8fe93, 00107 0x72552c85, 0x398cdd32, 0x71fa3949, 0x3a402dd2, 0x719e2cd2, 0x3af2eeb7, 00108 0x71410805, 0x3ba51e29, 00109 0x70e2cbc6, 0x3c56ba70, 0x708378ff, 0x3d07c1d6, 0x7023109a, 0x3db832a6, 00110 0x6fc19385, 0x3e680b2c, 00111 0x6f5f02b2, 0x3f1749b8, 0x6efb5f12, 0x3fc5ec98, 0x6e96a99d, 0x4073f21d, 00112 0x6e30e34a, 0x4121589b, 00113 0x6dca0d14, 0x41ce1e65, 0x6d6227fa, 0x427a41d0, 0x6cf934fc, 0x4325c135, 00114 0x6c8f351c, 0x43d09aed, 00115 0x6c242960, 0x447acd50, 0x6bb812d1, 0x452456bd, 0x6b4af279, 0x45cd358f, 00116 0x6adcc964, 0x46756828, 00117 0x6a6d98a4, 0x471cece7, 0x69fd614a, 0x47c3c22f, 0x698c246c, 0x4869e665, 00118 0x6919e320, 0x490f57ee, 00119 0x68a69e81, 0x49b41533, 0x683257ab, 0x4a581c9e, 0x67bd0fbd, 0x4afb6c98, 00120 0x6746c7d8, 0x4b9e0390, 00121 0x66cf8120, 0x4c3fdff4, 0x66573cbb, 0x4ce10034, 0x65ddfbd3, 0x4d8162c4, 00122 0x6563bf92, 0x4e210617, 00123 0x64e88926, 0x4ebfe8a5, 0x646c59bf, 0x4f5e08e3, 0x63ef3290, 0x4ffb654d, 00124 0x637114cc, 0x5097fc5e, 00125 0x62f201ac, 0x5133cc94, 0x6271fa69, 0x51ced46e, 0x61f1003f, 0x5269126e, 00126 0x616f146c, 0x53028518, 00127 0x60ec3830, 0x539b2af0, 0x60686ccf, 0x5433027d, 0x5fe3b38d, 0x54ca0a4b, 00128 0x5f5e0db3, 0x556040e2, 00129 0x5ed77c8a, 0x55f5a4d2, 0x5e50015d, 0x568a34a9, 0x5dc79d7c, 0x571deefa, 00130 0x5d3e5237, 0x57b0d256, 00131 0x5cb420e0, 0x5842dd54, 0x5c290acc, 0x58d40e8c, 0x5b9d1154, 0x59646498, 00132 0x5b1035cf, 0x59f3de12, 00133 0x5a82799a, 0x5a82799a, 0x59f3de12, 0x5b1035cf, 0x59646498, 0x5b9d1154, 00134 0x58d40e8c, 0x5c290acc, 00135 0x5842dd54, 0x5cb420e0, 0x57b0d256, 0x5d3e5237, 0x571deefa, 0x5dc79d7c, 00136 0x568a34a9, 0x5e50015d, 00137 0x55f5a4d2, 0x5ed77c8a, 0x556040e2, 0x5f5e0db3, 0x54ca0a4b, 0x5fe3b38d, 00138 0x5433027d, 0x60686ccf, 00139 0x539b2af0, 0x60ec3830, 0x53028518, 0x616f146c, 0x5269126e, 0x61f1003f, 00140 0x51ced46e, 0x6271fa69, 00141 0x5133cc94, 0x62f201ac, 0x5097fc5e, 0x637114cc, 0x4ffb654d, 0x63ef3290, 00142 0x4f5e08e3, 0x646c59bf, 00143 0x4ebfe8a5, 0x64e88926, 0x4e210617, 0x6563bf92, 0x4d8162c4, 0x65ddfbd3, 00144 0x4ce10034, 0x66573cbb, 00145 0x4c3fdff4, 0x66cf8120, 0x4b9e0390, 0x6746c7d8, 0x4afb6c98, 0x67bd0fbd, 00146 0x4a581c9e, 0x683257ab, 00147 0x49b41533, 0x68a69e81, 0x490f57ee, 0x6919e320, 0x4869e665, 0x698c246c, 00148 0x47c3c22f, 0x69fd614a, 00149 0x471cece7, 0x6a6d98a4, 0x46756828, 0x6adcc964, 0x45cd358f, 0x6b4af279, 00150 0x452456bd, 0x6bb812d1, 00151 0x447acd50, 0x6c242960, 0x43d09aed, 0x6c8f351c, 0x4325c135, 0x6cf934fc, 00152 0x427a41d0, 0x6d6227fa, 00153 0x41ce1e65, 0x6dca0d14, 0x4121589b, 0x6e30e34a, 0x4073f21d, 0x6e96a99d, 00154 0x3fc5ec98, 0x6efb5f12, 00155 0x3f1749b8, 0x6f5f02b2, 0x3e680b2c, 0x6fc19385, 0x3db832a6, 0x7023109a, 00156 0x3d07c1d6, 0x708378ff, 00157 0x3c56ba70, 0x70e2cbc6, 0x3ba51e29, 0x71410805, 0x3af2eeb7, 0x719e2cd2, 00158 0x3a402dd2, 0x71fa3949, 00159 0x398cdd32, 0x72552c85, 0x38d8fe93, 0x72af05a7, 0x382493b0, 0x7307c3d0, 00160 0x376f9e46, 0x735f6626, 00161 0x36ba2014, 0x73b5ebd1, 0x36041ad9, 0x740b53fb, 0x354d9057, 0x745f9dd1, 00162 0x34968250, 0x74b2c884, 00163 0x33def287, 0x7504d345, 0x3326e2c3, 0x7555bd4c, 0x326e54c7, 0x75a585cf, 00164 0x31b54a5e, 0x75f42c0b, 00165 0x30fbc54d, 0x7641af3d, 0x3041c761, 0x768e0ea6, 0x2f875262, 0x76d94989, 00166 0x2ecc681e, 0x77235f2d, 00167 0x2e110a62, 0x776c4edb, 0x2d553afc, 0x77b417df, 0x2c98fbba, 0x77fab989, 00168 0x2bdc4e6f, 0x78403329, 00169 0x2b1f34eb, 0x78848414, 0x2a61b101, 0x78c7aba2, 0x29a3c485, 0x7909a92d, 00170 0x28e5714b, 0x794a7c12, 00171 0x2826b928, 0x798a23b1, 0x27679df4, 0x79c89f6e, 0x26a82186, 0x7a05eead, 00172 0x25e845b6, 0x7a4210d8, 00173 0x25280c5e, 0x7a7d055b, 0x24677758, 0x7ab6cba4, 0x23a6887f, 0x7aef6323, 00174 0x22e541af, 0x7b26cb4f, 00175 0x2223a4c5, 0x7b5d039e, 0x2161b3a0, 0x7b920b89, 0x209f701c, 0x7bc5e290, 00176 0x1fdcdc1b, 0x7bf88830, 00177 0x1f19f97b, 0x7c29fbee, 0x1e56ca1e, 0x7c5a3d50, 0x1d934fe5, 0x7c894bde, 00178 0x1ccf8cb3, 0x7cb72724, 00179 0x1c0b826a, 0x7ce3ceb2, 0x1b4732ef, 0x7d0f4218, 0x1a82a026, 0x7d3980ec, 00180 0x19bdcbf3, 0x7d628ac6, 00181 0x18f8b83c, 0x7d8a5f40, 0x183366e9, 0x7db0fdf8, 0x176dd9de, 0x7dd6668f, 00182 0x16a81305, 0x7dfa98a8, 00183 0x15e21445, 0x7e1d93ea, 0x151bdf86, 0x7e3f57ff, 0x145576b1, 0x7e5fe493, 00184 0x138edbb1, 0x7e7f3957, 00185 0x12c8106f, 0x7e9d55fc, 0x120116d5, 0x7eba3a39, 0x1139f0cf, 0x7ed5e5c6, 00186 0x1072a048, 0x7ef05860, 00187 0xfab272b, 0x7f0991c4, 0xee38766, 0x7f2191b4, 0xe1bc2e4, 0x7f3857f6, 00188 0xd53db92, 0x7f4de451, 00189 0xc8bd35e, 0x7f62368f, 0xbc3ac35, 0x7f754e80, 0xafb6805, 0x7f872bf3, 00190 0xa3308bd, 0x7f97cebd, 00191 0x96a9049, 0x7fa736b4, 0x8a2009a, 0x7fb563b3, 0x7d95b9e, 0x7fc25596, 00192 0x710a345, 0x7fce0c3e, 00193 0x647d97c, 0x7fd8878e, 0x57f0035, 0x7fe1c76b, 0x4b6195d, 0x7fe9cbc0, 00194 0x3ed26e6, 0x7ff09478, 00195 0x3242abf, 0x7ff62182, 0x25b26d7, 0x7ffa72d1, 0x1921d20, 0x7ffd885a, 00196 0xc90f88, 0x7fff6216, 00197 0x0, 0x7fffffff, 0xff36f078, 0x7fff6216, 0xfe6de2e0, 0x7ffd885a, 0xfda4d929, 00198 0x7ffa72d1, 00199 0xfcdbd541, 0x7ff62182, 0xfc12d91a, 0x7ff09478, 0xfb49e6a3, 0x7fe9cbc0, 00200 0xfa80ffcb, 0x7fe1c76b, 00201 0xf9b82684, 0x7fd8878e, 0xf8ef5cbb, 0x7fce0c3e, 0xf826a462, 0x7fc25596, 00202 0xf75dff66, 0x7fb563b3, 00203 0xf6956fb7, 0x7fa736b4, 0xf5ccf743, 0x7f97cebd, 0xf50497fb, 0x7f872bf3, 00204 0xf43c53cb, 0x7f754e80, 00205 0xf3742ca2, 0x7f62368f, 0xf2ac246e, 0x7f4de451, 0xf1e43d1c, 0x7f3857f6, 00206 0xf11c789a, 0x7f2191b4, 00207 0xf054d8d5, 0x7f0991c4, 0xef8d5fb8, 0x7ef05860, 0xeec60f31, 0x7ed5e5c6, 00208 0xedfee92b, 0x7eba3a39, 00209 0xed37ef91, 0x7e9d55fc, 0xec71244f, 0x7e7f3957, 0xebaa894f, 0x7e5fe493, 00210 0xeae4207a, 0x7e3f57ff, 00211 0xea1debbb, 0x7e1d93ea, 0xe957ecfb, 0x7dfa98a8, 0xe8922622, 0x7dd6668f, 00212 0xe7cc9917, 0x7db0fdf8, 00213 0xe70747c4, 0x7d8a5f40, 0xe642340d, 0x7d628ac6, 0xe57d5fda, 0x7d3980ec, 00214 0xe4b8cd11, 0x7d0f4218, 00215 0xe3f47d96, 0x7ce3ceb2, 0xe330734d, 0x7cb72724, 0xe26cb01b, 0x7c894bde, 00216 0xe1a935e2, 0x7c5a3d50, 00217 0xe0e60685, 0x7c29fbee, 0xe02323e5, 0x7bf88830, 0xdf608fe4, 0x7bc5e290, 00218 0xde9e4c60, 0x7b920b89, 00219 0xdddc5b3b, 0x7b5d039e, 0xdd1abe51, 0x7b26cb4f, 0xdc597781, 0x7aef6323, 00220 0xdb9888a8, 0x7ab6cba4, 00221 0xdad7f3a2, 0x7a7d055b, 0xda17ba4a, 0x7a4210d8, 0xd957de7a, 0x7a05eead, 00222 0xd898620c, 0x79c89f6e, 00223 0xd7d946d8, 0x798a23b1, 0xd71a8eb5, 0x794a7c12, 0xd65c3b7b, 0x7909a92d, 00224 0xd59e4eff, 0x78c7aba2, 00225 0xd4e0cb15, 0x78848414, 0xd423b191, 0x78403329, 0xd3670446, 0x77fab989, 00226 0xd2aac504, 0x77b417df, 00227 0xd1eef59e, 0x776c4edb, 0xd13397e2, 0x77235f2d, 0xd078ad9e, 0x76d94989, 00228 0xcfbe389f, 0x768e0ea6, 00229 0xcf043ab3, 0x7641af3d, 0xce4ab5a2, 0x75f42c0b, 0xcd91ab39, 0x75a585cf, 00230 0xccd91d3d, 0x7555bd4c, 00231 0xcc210d79, 0x7504d345, 0xcb697db0, 0x74b2c884, 0xcab26fa9, 0x745f9dd1, 00232 0xc9fbe527, 0x740b53fb, 00233 0xc945dfec, 0x73b5ebd1, 0xc89061ba, 0x735f6626, 0xc7db6c50, 0x7307c3d0, 00234 0xc727016d, 0x72af05a7, 00235 0xc67322ce, 0x72552c85, 0xc5bfd22e, 0x71fa3949, 0xc50d1149, 0x719e2cd2, 00236 0xc45ae1d7, 0x71410805, 00237 0xc3a94590, 0x70e2cbc6, 0xc2f83e2a, 0x708378ff, 0xc247cd5a, 0x7023109a, 00238 0xc197f4d4, 0x6fc19385, 00239 0xc0e8b648, 0x6f5f02b2, 0xc03a1368, 0x6efb5f12, 0xbf8c0de3, 0x6e96a99d, 00240 0xbedea765, 0x6e30e34a, 00241 0xbe31e19b, 0x6dca0d14, 0xbd85be30, 0x6d6227fa, 0xbcda3ecb, 0x6cf934fc, 00242 0xbc2f6513, 0x6c8f351c, 00243 0xbb8532b0, 0x6c242960, 0xbadba943, 0x6bb812d1, 0xba32ca71, 0x6b4af279, 00244 0xb98a97d8, 0x6adcc964, 00245 0xb8e31319, 0x6a6d98a4, 0xb83c3dd1, 0x69fd614a, 0xb796199b, 0x698c246c, 00246 0xb6f0a812, 0x6919e320, 00247 0xb64beacd, 0x68a69e81, 0xb5a7e362, 0x683257ab, 0xb5049368, 0x67bd0fbd, 00248 0xb461fc70, 0x6746c7d8, 00249 0xb3c0200c, 0x66cf8120, 0xb31effcc, 0x66573cbb, 0xb27e9d3c, 0x65ddfbd3, 00250 0xb1def9e9, 0x6563bf92, 00251 0xb140175b, 0x64e88926, 0xb0a1f71d, 0x646c59bf, 0xb0049ab3, 0x63ef3290, 00252 0xaf6803a2, 0x637114cc, 00253 0xaecc336c, 0x62f201ac, 0xae312b92, 0x6271fa69, 0xad96ed92, 0x61f1003f, 00254 0xacfd7ae8, 0x616f146c, 00255 0xac64d510, 0x60ec3830, 0xabccfd83, 0x60686ccf, 0xab35f5b5, 0x5fe3b38d, 00256 0xaa9fbf1e, 0x5f5e0db3, 00257 0xaa0a5b2e, 0x5ed77c8a, 0xa975cb57, 0x5e50015d, 0xa8e21106, 0x5dc79d7c, 00258 0xa84f2daa, 0x5d3e5237, 00259 0xa7bd22ac, 0x5cb420e0, 0xa72bf174, 0x5c290acc, 0xa69b9b68, 0x5b9d1154, 00260 0xa60c21ee, 0x5b1035cf, 00261 0xa57d8666, 0x5a82799a, 0xa4efca31, 0x59f3de12, 0xa462eeac, 0x59646498, 00262 0xa3d6f534, 0x58d40e8c, 00263 0xa34bdf20, 0x5842dd54, 0xa2c1adc9, 0x57b0d256, 0xa2386284, 0x571deefa, 00264 0xa1affea3, 0x568a34a9, 00265 0xa1288376, 0x55f5a4d2, 0xa0a1f24d, 0x556040e2, 0xa01c4c73, 0x54ca0a4b, 00266 0x9f979331, 0x5433027d, 00267 0x9f13c7d0, 0x539b2af0, 0x9e90eb94, 0x53028518, 0x9e0effc1, 0x5269126e, 00268 0x9d8e0597, 0x51ced46e, 00269 0x9d0dfe54, 0x5133cc94, 0x9c8eeb34, 0x5097fc5e, 0x9c10cd70, 0x4ffb654d, 00270 0x9b93a641, 0x4f5e08e3, 00271 0x9b1776da, 0x4ebfe8a5, 0x9a9c406e, 0x4e210617, 0x9a22042d, 0x4d8162c4, 00272 0x99a8c345, 0x4ce10034, 00273 0x99307ee0, 0x4c3fdff4, 0x98b93828, 0x4b9e0390, 0x9842f043, 0x4afb6c98, 00274 0x97cda855, 0x4a581c9e, 00275 0x9759617f, 0x49b41533, 0x96e61ce0, 0x490f57ee, 0x9673db94, 0x4869e665, 00276 0x96029eb6, 0x47c3c22f, 00277 0x9592675c, 0x471cece7, 0x9523369c, 0x46756828, 0x94b50d87, 0x45cd358f, 00278 0x9447ed2f, 0x452456bd, 00279 0x93dbd6a0, 0x447acd50, 0x9370cae4, 0x43d09aed, 0x9306cb04, 0x4325c135, 00280 0x929dd806, 0x427a41d0, 00281 0x9235f2ec, 0x41ce1e65, 0x91cf1cb6, 0x4121589b, 0x91695663, 0x4073f21d, 00282 0x9104a0ee, 0x3fc5ec98, 00283 0x90a0fd4e, 0x3f1749b8, 0x903e6c7b, 0x3e680b2c, 0x8fdcef66, 0x3db832a6, 00284 0x8f7c8701, 0x3d07c1d6, 00285 0x8f1d343a, 0x3c56ba70, 0x8ebef7fb, 0x3ba51e29, 0x8e61d32e, 0x3af2eeb7, 00286 0x8e05c6b7, 0x3a402dd2, 00287 0x8daad37b, 0x398cdd32, 0x8d50fa59, 0x38d8fe93, 0x8cf83c30, 0x382493b0, 00288 0x8ca099da, 0x376f9e46, 00289 0x8c4a142f, 0x36ba2014, 0x8bf4ac05, 0x36041ad9, 0x8ba0622f, 0x354d9057, 00290 0x8b4d377c, 0x34968250, 00291 0x8afb2cbb, 0x33def287, 0x8aaa42b4, 0x3326e2c3, 0x8a5a7a31, 0x326e54c7, 00292 0x8a0bd3f5, 0x31b54a5e, 00293 0x89be50c3, 0x30fbc54d, 0x8971f15a, 0x3041c761, 0x8926b677, 0x2f875262, 00294 0x88dca0d3, 0x2ecc681e, 00295 0x8893b125, 0x2e110a62, 0x884be821, 0x2d553afc, 0x88054677, 0x2c98fbba, 00296 0x87bfccd7, 0x2bdc4e6f, 00297 0x877b7bec, 0x2b1f34eb, 0x8738545e, 0x2a61b101, 0x86f656d3, 0x29a3c485, 00298 0x86b583ee, 0x28e5714b, 00299 0x8675dc4f, 0x2826b928, 0x86376092, 0x27679df4, 0x85fa1153, 0x26a82186, 00300 0x85bdef28, 0x25e845b6, 00301 0x8582faa5, 0x25280c5e, 0x8549345c, 0x24677758, 0x85109cdd, 0x23a6887f, 00302 0x84d934b1, 0x22e541af, 00303 0x84a2fc62, 0x2223a4c5, 0x846df477, 0x2161b3a0, 0x843a1d70, 0x209f701c, 00304 0x840777d0, 0x1fdcdc1b, 00305 0x83d60412, 0x1f19f97b, 0x83a5c2b0, 0x1e56ca1e, 0x8376b422, 0x1d934fe5, 00306 0x8348d8dc, 0x1ccf8cb3, 00307 0x831c314e, 0x1c0b826a, 0x82f0bde8, 0x1b4732ef, 0x82c67f14, 0x1a82a026, 00308 0x829d753a, 0x19bdcbf3, 00309 0x8275a0c0, 0x18f8b83c, 0x824f0208, 0x183366e9, 0x82299971, 0x176dd9de, 00310 0x82056758, 0x16a81305, 00311 0x81e26c16, 0x15e21445, 0x81c0a801, 0x151bdf86, 0x81a01b6d, 0x145576b1, 00312 0x8180c6a9, 0x138edbb1, 00313 0x8162aa04, 0x12c8106f, 0x8145c5c7, 0x120116d5, 0x812a1a3a, 0x1139f0cf, 00314 0x810fa7a0, 0x1072a048, 00315 0x80f66e3c, 0xfab272b, 0x80de6e4c, 0xee38766, 0x80c7a80a, 0xe1bc2e4, 00316 0x80b21baf, 0xd53db92, 00317 0x809dc971, 0xc8bd35e, 0x808ab180, 0xbc3ac35, 0x8078d40d, 0xafb6805, 00318 0x80683143, 0xa3308bd, 00319 0x8058c94c, 0x96a9049, 0x804a9c4d, 0x8a2009a, 0x803daa6a, 0x7d95b9e, 00320 0x8031f3c2, 0x710a345, 00321 0x80277872, 0x647d97c, 0x801e3895, 0x57f0035, 0x80163440, 0x4b6195d, 00322 0x800f6b88, 0x3ed26e6, 00323 0x8009de7e, 0x3242abf, 0x80058d2f, 0x25b26d7, 0x800277a6, 0x1921d20, 00324 0x80009dea, 0xc90f88, 00325 0x80000000, 0x0, 0x80009dea, 0xff36f078, 0x800277a6, 0xfe6de2e0, 0x80058d2f, 00326 0xfda4d929, 00327 0x8009de7e, 0xfcdbd541, 0x800f6b88, 0xfc12d91a, 0x80163440, 0xfb49e6a3, 00328 0x801e3895, 0xfa80ffcb, 00329 0x80277872, 0xf9b82684, 0x8031f3c2, 0xf8ef5cbb, 0x803daa6a, 0xf826a462, 00330 0x804a9c4d, 0xf75dff66, 00331 0x8058c94c, 0xf6956fb7, 0x80683143, 0xf5ccf743, 0x8078d40d, 0xf50497fb, 00332 0x808ab180, 0xf43c53cb, 00333 0x809dc971, 0xf3742ca2, 0x80b21baf, 0xf2ac246e, 0x80c7a80a, 0xf1e43d1c, 00334 0x80de6e4c, 0xf11c789a, 00335 0x80f66e3c, 0xf054d8d5, 0x810fa7a0, 0xef8d5fb8, 0x812a1a3a, 0xeec60f31, 00336 0x8145c5c7, 0xedfee92b, 00337 0x8162aa04, 0xed37ef91, 0x8180c6a9, 0xec71244f, 0x81a01b6d, 0xebaa894f, 00338 0x81c0a801, 0xeae4207a, 00339 0x81e26c16, 0xea1debbb, 0x82056758, 0xe957ecfb, 0x82299971, 0xe8922622, 00340 0x824f0208, 0xe7cc9917, 00341 0x8275a0c0, 0xe70747c4, 0x829d753a, 0xe642340d, 0x82c67f14, 0xe57d5fda, 00342 0x82f0bde8, 0xe4b8cd11, 00343 0x831c314e, 0xe3f47d96, 0x8348d8dc, 0xe330734d, 0x8376b422, 0xe26cb01b, 00344 0x83a5c2b0, 0xe1a935e2, 00345 0x83d60412, 0xe0e60685, 0x840777d0, 0xe02323e5, 0x843a1d70, 0xdf608fe4, 00346 0x846df477, 0xde9e4c60, 00347 0x84a2fc62, 0xdddc5b3b, 0x84d934b1, 0xdd1abe51, 0x85109cdd, 0xdc597781, 00348 0x8549345c, 0xdb9888a8, 00349 0x8582faa5, 0xdad7f3a2, 0x85bdef28, 0xda17ba4a, 0x85fa1153, 0xd957de7a, 00350 0x86376092, 0xd898620c, 00351 0x8675dc4f, 0xd7d946d8, 0x86b583ee, 0xd71a8eb5, 0x86f656d3, 0xd65c3b7b, 00352 0x8738545e, 0xd59e4eff, 00353 0x877b7bec, 0xd4e0cb15, 0x87bfccd7, 0xd423b191, 0x88054677, 0xd3670446, 00354 0x884be821, 0xd2aac504, 00355 0x8893b125, 0xd1eef59e, 0x88dca0d3, 0xd13397e2, 0x8926b677, 0xd078ad9e, 00356 0x8971f15a, 0xcfbe389f, 00357 0x89be50c3, 0xcf043ab3, 0x8a0bd3f5, 0xce4ab5a2, 0x8a5a7a31, 0xcd91ab39, 00358 0x8aaa42b4, 0xccd91d3d, 00359 0x8afb2cbb, 0xcc210d79, 0x8b4d377c, 0xcb697db0, 0x8ba0622f, 0xcab26fa9, 00360 0x8bf4ac05, 0xc9fbe527, 00361 0x8c4a142f, 0xc945dfec, 0x8ca099da, 0xc89061ba, 0x8cf83c30, 0xc7db6c50, 00362 0x8d50fa59, 0xc727016d, 00363 0x8daad37b, 0xc67322ce, 0x8e05c6b7, 0xc5bfd22e, 0x8e61d32e, 0xc50d1149, 00364 0x8ebef7fb, 0xc45ae1d7, 00365 0x8f1d343a, 0xc3a94590, 0x8f7c8701, 0xc2f83e2a, 0x8fdcef66, 0xc247cd5a, 00366 0x903e6c7b, 0xc197f4d4, 00367 0x90a0fd4e, 0xc0e8b648, 0x9104a0ee, 0xc03a1368, 0x91695663, 0xbf8c0de3, 00368 0x91cf1cb6, 0xbedea765, 00369 0x9235f2ec, 0xbe31e19b, 0x929dd806, 0xbd85be30, 0x9306cb04, 0xbcda3ecb, 00370 0x9370cae4, 0xbc2f6513, 00371 0x93dbd6a0, 0xbb8532b0, 0x9447ed2f, 0xbadba943, 0x94b50d87, 0xba32ca71, 00372 0x9523369c, 0xb98a97d8, 00373 0x9592675c, 0xb8e31319, 0x96029eb6, 0xb83c3dd1, 0x9673db94, 0xb796199b, 00374 0x96e61ce0, 0xb6f0a812, 00375 0x9759617f, 0xb64beacd, 0x97cda855, 0xb5a7e362, 0x9842f043, 0xb5049368, 00376 0x98b93828, 0xb461fc70, 00377 0x99307ee0, 0xb3c0200c, 0x99a8c345, 0xb31effcc, 0x9a22042d, 0xb27e9d3c, 00378 0x9a9c406e, 0xb1def9e9, 00379 0x9b1776da, 0xb140175b, 0x9b93a641, 0xb0a1f71d, 0x9c10cd70, 0xb0049ab3, 00380 0x9c8eeb34, 0xaf6803a2, 00381 0x9d0dfe54, 0xaecc336c, 0x9d8e0597, 0xae312b92, 0x9e0effc1, 0xad96ed92, 00382 0x9e90eb94, 0xacfd7ae8, 00383 0x9f13c7d0, 0xac64d510, 0x9f979331, 0xabccfd83, 0xa01c4c73, 0xab35f5b5, 00384 0xa0a1f24d, 0xaa9fbf1e, 00385 0xa1288376, 0xaa0a5b2e, 0xa1affea3, 0xa975cb57, 0xa2386284, 0xa8e21106, 00386 0xa2c1adc9, 0xa84f2daa, 00387 0xa34bdf20, 0xa7bd22ac, 0xa3d6f534, 0xa72bf174, 0xa462eeac, 0xa69b9b68, 00388 0xa4efca31, 0xa60c21ee, 00389 0xa57d8666, 0xa57d8666, 0xa60c21ee, 0xa4efca31, 0xa69b9b68, 0xa462eeac, 00390 0xa72bf174, 0xa3d6f534, 00391 0xa7bd22ac, 0xa34bdf20, 0xa84f2daa, 0xa2c1adc9, 0xa8e21106, 0xa2386284, 00392 0xa975cb57, 0xa1affea3, 00393 0xaa0a5b2e, 0xa1288376, 0xaa9fbf1e, 0xa0a1f24d, 0xab35f5b5, 0xa01c4c73, 00394 0xabccfd83, 0x9f979331, 00395 0xac64d510, 0x9f13c7d0, 0xacfd7ae8, 0x9e90eb94, 0xad96ed92, 0x9e0effc1, 00396 0xae312b92, 0x9d8e0597, 00397 0xaecc336c, 0x9d0dfe54, 0xaf6803a2, 0x9c8eeb34, 0xb0049ab3, 0x9c10cd70, 00398 0xb0a1f71d, 0x9b93a641, 00399 0xb140175b, 0x9b1776da, 0xb1def9e9, 0x9a9c406e, 0xb27e9d3c, 0x9a22042d, 00400 0xb31effcc, 0x99a8c345, 00401 0xb3c0200c, 0x99307ee0, 0xb461fc70, 0x98b93828, 0xb5049368, 0x9842f043, 00402 0xb5a7e362, 0x97cda855, 00403 0xb64beacd, 0x9759617f, 0xb6f0a812, 0x96e61ce0, 0xb796199b, 0x9673db94, 00404 0xb83c3dd1, 0x96029eb6, 00405 0xb8e31319, 0x9592675c, 0xb98a97d8, 0x9523369c, 0xba32ca71, 0x94b50d87, 00406 0xbadba943, 0x9447ed2f, 00407 0xbb8532b0, 0x93dbd6a0, 0xbc2f6513, 0x9370cae4, 0xbcda3ecb, 0x9306cb04, 00408 0xbd85be30, 0x929dd806, 00409 0xbe31e19b, 0x9235f2ec, 0xbedea765, 0x91cf1cb6, 0xbf8c0de3, 0x91695663, 00410 0xc03a1368, 0x9104a0ee, 00411 0xc0e8b648, 0x90a0fd4e, 0xc197f4d4, 0x903e6c7b, 0xc247cd5a, 0x8fdcef66, 00412 0xc2f83e2a, 0x8f7c8701, 00413 0xc3a94590, 0x8f1d343a, 0xc45ae1d7, 0x8ebef7fb, 0xc50d1149, 0x8e61d32e, 00414 0xc5bfd22e, 0x8e05c6b7, 00415 0xc67322ce, 0x8daad37b, 0xc727016d, 0x8d50fa59, 0xc7db6c50, 0x8cf83c30, 00416 0xc89061ba, 0x8ca099da, 00417 0xc945dfec, 0x8c4a142f, 0xc9fbe527, 0x8bf4ac05, 0xcab26fa9, 0x8ba0622f, 00418 0xcb697db0, 0x8b4d377c, 00419 0xcc210d79, 0x8afb2cbb, 0xccd91d3d, 0x8aaa42b4, 0xcd91ab39, 0x8a5a7a31, 00420 0xce4ab5a2, 0x8a0bd3f5, 00421 0xcf043ab3, 0x89be50c3, 0xcfbe389f, 0x8971f15a, 0xd078ad9e, 0x8926b677, 00422 0xd13397e2, 0x88dca0d3, 00423 0xd1eef59e, 0x8893b125, 0xd2aac504, 0x884be821, 0xd3670446, 0x88054677, 00424 0xd423b191, 0x87bfccd7, 00425 0xd4e0cb15, 0x877b7bec, 0xd59e4eff, 0x8738545e, 0xd65c3b7b, 0x86f656d3, 00426 0xd71a8eb5, 0x86b583ee, 00427 0xd7d946d8, 0x8675dc4f, 0xd898620c, 0x86376092, 0xd957de7a, 0x85fa1153, 00428 0xda17ba4a, 0x85bdef28, 00429 0xdad7f3a2, 0x8582faa5, 0xdb9888a8, 0x8549345c, 0xdc597781, 0x85109cdd, 00430 0xdd1abe51, 0x84d934b1, 00431 0xdddc5b3b, 0x84a2fc62, 0xde9e4c60, 0x846df477, 0xdf608fe4, 0x843a1d70, 00432 0xe02323e5, 0x840777d0, 00433 0xe0e60685, 0x83d60412, 0xe1a935e2, 0x83a5c2b0, 0xe26cb01b, 0x8376b422, 00434 0xe330734d, 0x8348d8dc, 00435 0xe3f47d96, 0x831c314e, 0xe4b8cd11, 0x82f0bde8, 0xe57d5fda, 0x82c67f14, 00436 0xe642340d, 0x829d753a, 00437 0xe70747c4, 0x8275a0c0, 0xe7cc9917, 0x824f0208, 0xe8922622, 0x82299971, 00438 0xe957ecfb, 0x82056758, 00439 0xea1debbb, 0x81e26c16, 0xeae4207a, 0x81c0a801, 0xebaa894f, 0x81a01b6d, 00440 0xec71244f, 0x8180c6a9, 00441 0xed37ef91, 0x8162aa04, 0xedfee92b, 0x8145c5c7, 0xeec60f31, 0x812a1a3a, 00442 0xef8d5fb8, 0x810fa7a0, 00443 0xf054d8d5, 0x80f66e3c, 0xf11c789a, 0x80de6e4c, 0xf1e43d1c, 0x80c7a80a, 00444 0xf2ac246e, 0x80b21baf, 00445 0xf3742ca2, 0x809dc971, 0xf43c53cb, 0x808ab180, 0xf50497fb, 0x8078d40d, 00446 0xf5ccf743, 0x80683143, 00447 0xf6956fb7, 0x8058c94c, 0xf75dff66, 0x804a9c4d, 0xf826a462, 0x803daa6a, 00448 0xf8ef5cbb, 0x8031f3c2, 00449 0xf9b82684, 0x80277872, 0xfa80ffcb, 0x801e3895, 0xfb49e6a3, 0x80163440, 00450 0xfc12d91a, 0x800f6b88, 00451 0xfcdbd541, 0x8009de7e, 0xfda4d929, 0x80058d2f, 0xfe6de2e0, 0x800277a6, 00452 0xff36f078, 0x80009dea, 00453 0x0, 0x80000000, 0xc90f88, 0x80009dea, 0x1921d20, 0x800277a6, 0x25b26d7, 00454 0x80058d2f, 00455 0x3242abf, 0x8009de7e, 0x3ed26e6, 0x800f6b88, 0x4b6195d, 0x80163440, 00456 0x57f0035, 0x801e3895, 00457 0x647d97c, 0x80277872, 0x710a345, 0x8031f3c2, 0x7d95b9e, 0x803daa6a, 00458 0x8a2009a, 0x804a9c4d, 00459 0x96a9049, 0x8058c94c, 0xa3308bd, 0x80683143, 0xafb6805, 0x8078d40d, 00460 0xbc3ac35, 0x808ab180, 00461 0xc8bd35e, 0x809dc971, 0xd53db92, 0x80b21baf, 0xe1bc2e4, 0x80c7a80a, 00462 0xee38766, 0x80de6e4c, 00463 0xfab272b, 0x80f66e3c, 0x1072a048, 0x810fa7a0, 0x1139f0cf, 0x812a1a3a, 00464 0x120116d5, 0x8145c5c7, 00465 0x12c8106f, 0x8162aa04, 0x138edbb1, 0x8180c6a9, 0x145576b1, 0x81a01b6d, 00466 0x151bdf86, 0x81c0a801, 00467 0x15e21445, 0x81e26c16, 0x16a81305, 0x82056758, 0x176dd9de, 0x82299971, 00468 0x183366e9, 0x824f0208, 00469 0x18f8b83c, 0x8275a0c0, 0x19bdcbf3, 0x829d753a, 0x1a82a026, 0x82c67f14, 00470 0x1b4732ef, 0x82f0bde8, 00471 0x1c0b826a, 0x831c314e, 0x1ccf8cb3, 0x8348d8dc, 0x1d934fe5, 0x8376b422, 00472 0x1e56ca1e, 0x83a5c2b0, 00473 0x1f19f97b, 0x83d60412, 0x1fdcdc1b, 0x840777d0, 0x209f701c, 0x843a1d70, 00474 0x2161b3a0, 0x846df477, 00475 0x2223a4c5, 0x84a2fc62, 0x22e541af, 0x84d934b1, 0x23a6887f, 0x85109cdd, 00476 0x24677758, 0x8549345c, 00477 0x25280c5e, 0x8582faa5, 0x25e845b6, 0x85bdef28, 0x26a82186, 0x85fa1153, 00478 0x27679df4, 0x86376092, 00479 0x2826b928, 0x8675dc4f, 0x28e5714b, 0x86b583ee, 0x29a3c485, 0x86f656d3, 00480 0x2a61b101, 0x8738545e, 00481 0x2b1f34eb, 0x877b7bec, 0x2bdc4e6f, 0x87bfccd7, 0x2c98fbba, 0x88054677, 00482 0x2d553afc, 0x884be821, 00483 0x2e110a62, 0x8893b125, 0x2ecc681e, 0x88dca0d3, 0x2f875262, 0x8926b677, 00484 0x3041c761, 0x8971f15a, 00485 0x30fbc54d, 0x89be50c3, 0x31b54a5e, 0x8a0bd3f5, 0x326e54c7, 0x8a5a7a31, 00486 0x3326e2c3, 0x8aaa42b4, 00487 0x33def287, 0x8afb2cbb, 0x34968250, 0x8b4d377c, 0x354d9057, 0x8ba0622f, 00488 0x36041ad9, 0x8bf4ac05, 00489 0x36ba2014, 0x8c4a142f, 0x376f9e46, 0x8ca099da, 0x382493b0, 0x8cf83c30, 00490 0x38d8fe93, 0x8d50fa59, 00491 0x398cdd32, 0x8daad37b, 0x3a402dd2, 0x8e05c6b7, 0x3af2eeb7, 0x8e61d32e, 00492 0x3ba51e29, 0x8ebef7fb, 00493 0x3c56ba70, 0x8f1d343a, 0x3d07c1d6, 0x8f7c8701, 0x3db832a6, 0x8fdcef66, 00494 0x3e680b2c, 0x903e6c7b, 00495 0x3f1749b8, 0x90a0fd4e, 0x3fc5ec98, 0x9104a0ee, 0x4073f21d, 0x91695663, 00496 0x4121589b, 0x91cf1cb6, 00497 0x41ce1e65, 0x9235f2ec, 0x427a41d0, 0x929dd806, 0x4325c135, 0x9306cb04, 00498 0x43d09aed, 0x9370cae4, 00499 0x447acd50, 0x93dbd6a0, 0x452456bd, 0x9447ed2f, 0x45cd358f, 0x94b50d87, 00500 0x46756828, 0x9523369c, 00501 0x471cece7, 0x9592675c, 0x47c3c22f, 0x96029eb6, 0x4869e665, 0x9673db94, 00502 0x490f57ee, 0x96e61ce0, 00503 0x49b41533, 0x9759617f, 0x4a581c9e, 0x97cda855, 0x4afb6c98, 0x9842f043, 00504 0x4b9e0390, 0x98b93828, 00505 0x4c3fdff4, 0x99307ee0, 0x4ce10034, 0x99a8c345, 0x4d8162c4, 0x9a22042d, 00506 0x4e210617, 0x9a9c406e, 00507 0x4ebfe8a5, 0x9b1776da, 0x4f5e08e3, 0x9b93a641, 0x4ffb654d, 0x9c10cd70, 00508 0x5097fc5e, 0x9c8eeb34, 00509 0x5133cc94, 0x9d0dfe54, 0x51ced46e, 0x9d8e0597, 0x5269126e, 0x9e0effc1, 00510 0x53028518, 0x9e90eb94, 00511 0x539b2af0, 0x9f13c7d0, 0x5433027d, 0x9f979331, 0x54ca0a4b, 0xa01c4c73, 00512 0x556040e2, 0xa0a1f24d, 00513 0x55f5a4d2, 0xa1288376, 0x568a34a9, 0xa1affea3, 0x571deefa, 0xa2386284, 00514 0x57b0d256, 0xa2c1adc9, 00515 0x5842dd54, 0xa34bdf20, 0x58d40e8c, 0xa3d6f534, 0x59646498, 0xa462eeac, 00516 0x59f3de12, 0xa4efca31, 00517 0x5a82799a, 0xa57d8666, 0x5b1035cf, 0xa60c21ee, 0x5b9d1154, 0xa69b9b68, 00518 0x5c290acc, 0xa72bf174, 00519 0x5cb420e0, 0xa7bd22ac, 0x5d3e5237, 0xa84f2daa, 0x5dc79d7c, 0xa8e21106, 00520 0x5e50015d, 0xa975cb57, 00521 0x5ed77c8a, 0xaa0a5b2e, 0x5f5e0db3, 0xaa9fbf1e, 0x5fe3b38d, 0xab35f5b5, 00522 0x60686ccf, 0xabccfd83, 00523 0x60ec3830, 0xac64d510, 0x616f146c, 0xacfd7ae8, 0x61f1003f, 0xad96ed92, 00524 0x6271fa69, 0xae312b92, 00525 0x62f201ac, 0xaecc336c, 0x637114cc, 0xaf6803a2, 0x63ef3290, 0xb0049ab3, 00526 0x646c59bf, 0xb0a1f71d, 00527 0x64e88926, 0xb140175b, 0x6563bf92, 0xb1def9e9, 0x65ddfbd3, 0xb27e9d3c, 00528 0x66573cbb, 0xb31effcc, 00529 0x66cf8120, 0xb3c0200c, 0x6746c7d8, 0xb461fc70, 0x67bd0fbd, 0xb5049368, 00530 0x683257ab, 0xb5a7e362, 00531 0x68a69e81, 0xb64beacd, 0x6919e320, 0xb6f0a812, 0x698c246c, 0xb796199b, 00532 0x69fd614a, 0xb83c3dd1, 00533 0x6a6d98a4, 0xb8e31319, 0x6adcc964, 0xb98a97d8, 0x6b4af279, 0xba32ca71, 00534 0x6bb812d1, 0xbadba943, 00535 0x6c242960, 0xbb8532b0, 0x6c8f351c, 0xbc2f6513, 0x6cf934fc, 0xbcda3ecb, 00536 0x6d6227fa, 0xbd85be30, 00537 0x6dca0d14, 0xbe31e19b, 0x6e30e34a, 0xbedea765, 0x6e96a99d, 0xbf8c0de3, 00538 0x6efb5f12, 0xc03a1368, 00539 0x6f5f02b2, 0xc0e8b648, 0x6fc19385, 0xc197f4d4, 0x7023109a, 0xc247cd5a, 00540 0x708378ff, 0xc2f83e2a, 00541 0x70e2cbc6, 0xc3a94590, 0x71410805, 0xc45ae1d7, 0x719e2cd2, 0xc50d1149, 00542 0x71fa3949, 0xc5bfd22e, 00543 0x72552c85, 0xc67322ce, 0x72af05a7, 0xc727016d, 0x7307c3d0, 0xc7db6c50, 00544 0x735f6626, 0xc89061ba, 00545 0x73b5ebd1, 0xc945dfec, 0x740b53fb, 0xc9fbe527, 0x745f9dd1, 0xcab26fa9, 00546 0x74b2c884, 0xcb697db0, 00547 0x7504d345, 0xcc210d79, 0x7555bd4c, 0xccd91d3d, 0x75a585cf, 0xcd91ab39, 00548 0x75f42c0b, 0xce4ab5a2, 00549 0x7641af3d, 0xcf043ab3, 0x768e0ea6, 0xcfbe389f, 0x76d94989, 0xd078ad9e, 00550 0x77235f2d, 0xd13397e2, 00551 0x776c4edb, 0xd1eef59e, 0x77b417df, 0xd2aac504, 0x77fab989, 0xd3670446, 00552 0x78403329, 0xd423b191, 00553 0x78848414, 0xd4e0cb15, 0x78c7aba2, 0xd59e4eff, 0x7909a92d, 0xd65c3b7b, 00554 0x794a7c12, 0xd71a8eb5, 00555 0x798a23b1, 0xd7d946d8, 0x79c89f6e, 0xd898620c, 0x7a05eead, 0xd957de7a, 00556 0x7a4210d8, 0xda17ba4a, 00557 0x7a7d055b, 0xdad7f3a2, 0x7ab6cba4, 0xdb9888a8, 0x7aef6323, 0xdc597781, 00558 0x7b26cb4f, 0xdd1abe51, 00559 0x7b5d039e, 0xdddc5b3b, 0x7b920b89, 0xde9e4c60, 0x7bc5e290, 0xdf608fe4, 00560 0x7bf88830, 0xe02323e5, 00561 0x7c29fbee, 0xe0e60685, 0x7c5a3d50, 0xe1a935e2, 0x7c894bde, 0xe26cb01b, 00562 0x7cb72724, 0xe330734d, 00563 0x7ce3ceb2, 0xe3f47d96, 0x7d0f4218, 0xe4b8cd11, 0x7d3980ec, 0xe57d5fda, 00564 0x7d628ac6, 0xe642340d, 00565 0x7d8a5f40, 0xe70747c4, 0x7db0fdf8, 0xe7cc9917, 0x7dd6668f, 0xe8922622, 00566 0x7dfa98a8, 0xe957ecfb, 00567 0x7e1d93ea, 0xea1debbb, 0x7e3f57ff, 0xeae4207a, 0x7e5fe493, 0xebaa894f, 00568 0x7e7f3957, 0xec71244f, 00569 0x7e9d55fc, 0xed37ef91, 0x7eba3a39, 0xedfee92b, 0x7ed5e5c6, 0xeec60f31, 00570 0x7ef05860, 0xef8d5fb8, 00571 0x7f0991c4, 0xf054d8d5, 0x7f2191b4, 0xf11c789a, 0x7f3857f6, 0xf1e43d1c, 00572 0x7f4de451, 0xf2ac246e, 00573 0x7f62368f, 0xf3742ca2, 0x7f754e80, 0xf43c53cb, 0x7f872bf3, 0xf50497fb, 00574 0x7f97cebd, 0xf5ccf743, 00575 0x7fa736b4, 0xf6956fb7, 0x7fb563b3, 0xf75dff66, 0x7fc25596, 0xf826a462, 00576 0x7fce0c3e, 0xf8ef5cbb, 00577 0x7fd8878e, 0xf9b82684, 0x7fe1c76b, 0xfa80ffcb, 0x7fe9cbc0, 0xfb49e6a3, 00578 0x7ff09478, 0xfc12d91a, 00579 0x7ff62182, 0xfcdbd541, 0x7ffa72d1, 0xfda4d929, 0x7ffd885a, 0xfe6de2e0, 00580 0x7fff6216, 0xff36f078 00581 }; 00582 00583 /** 00584 * 00585 * @brief Initialization function for the Q31 CFFT/CIFFT. 00586 * @param[in,out] *S points to an instance of the Q31 CFFT/CIFFT structure. 00587 * @param[in] fftLen length of the FFT. 00588 * @param[in] ifftFlag flag that selects forward (ifftFlag=0) or inverse (ifftFlag=1) transform. 00589 * @param[in] bitReverseFlag flag that enables (bitReverseFlag=1) or disables (bitReverseFlag=0) bit reversal of output. 00590 * @return The function returns ARM_MATH_SUCCESS if initialization is successful or ARM_MATH_ARGUMENT_ERROR if <code>fftLen</code> is not a supported value. 00591 * 00592 * \par Description: 00593 * \par 00594 * The parameter <code>ifftFlag</code> controls whether a forward or inverse transform is computed. 00595 * Set(=1) ifftFlag for calculation of CIFFT otherwise CFFT is calculated 00596 * \par 00597 * The parameter <code>bitReverseFlag</code> controls whether output is in normal order or bit reversed order. 00598 * Set(=1) bitReverseFlag for output to be in normal order otherwise output is in bit reversed order. 00599 * \par 00600 * The parameter <code>fftLen</code> Specifies length of CFFT/CIFFT process. Supported FFT Lengths are 16, 64, 256, 1024. 00601 * \par 00602 * This Function also initializes Twiddle factor table pointer and Bit reversal table pointer. 00603 */ 00604 00605 arm_status arm_cfft_radix4_init_q31( 00606 arm_cfft_radix4_instance_q31 * S, 00607 uint16_t fftLen, 00608 uint8_t ifftFlag, 00609 uint8_t bitReverseFlag) 00610 { 00611 /* Initialise the default arm status */ 00612 arm_status status = ARM_MATH_SUCCESS; 00613 /* Initialise the FFT length */ 00614 S->fftLen = fftLen; 00615 /* Initialise the Twiddle coefficient pointer */ 00616 S->pTwiddle = (q31_t *) twiddleCoefQ31 ; 00617 /* Initialise the Flag for selection of CFFT or CIFFT */ 00618 S->ifftFlag = ifftFlag; 00619 /* Initialise the Flag for calculation Bit reversal or not */ 00620 S->bitReverseFlag = bitReverseFlag; 00621 00622 /* Initializations of Instance structure depending on the FFT length */ 00623 switch (S->fftLen) 00624 { 00625 /* Initializations of structure parameters for 1024 point FFT */ 00626 case 1024u: 00627 /* Initialise the twiddle coef modifier value */ 00628 S->twidCoefModifier = 1u; 00629 /* Initialise the bit reversal table modifier */ 00630 S->bitRevFactor = 1u; 00631 /* Initialise the bit reversal table pointer */ 00632 S->pBitRevTable = (uint16_t*)armBitRevTable ; 00633 break; 00634 00635 case 256u: 00636 /* Initializations of structure parameters for 256 point FFT */ 00637 S->twidCoefModifier = 4u; 00638 S->bitRevFactor = 4u; 00639 S->pBitRevTable = (uint16_t*)&armBitRevTable [3]; 00640 break; 00641 00642 case 64u: 00643 /* Initializations of structure parameters for 64 point FFT */ 00644 S->twidCoefModifier = 16u; 00645 S->bitRevFactor = 16u; 00646 S->pBitRevTable = (uint16_t*)&armBitRevTable [15]; 00647 break; 00648 00649 case 16u: 00650 /* Initializations of structure parameters for 16 point FFT */ 00651 S->twidCoefModifier = 64u; 00652 S->bitRevFactor = 64u; 00653 S->pBitRevTable = (uint16_t*)&armBitRevTable [63]; 00654 break; 00655 00656 default: 00657 /* Reporting argument error if fftSize is not valid value */ 00658 status = ARM_MATH_ARGUMENT_ERROR; 00659 break; 00660 } 00661 00662 return (status); 00663 } 00664 00665 /** 00666 * @} end of CFFT_CIFFT group 00667 */
Generated on Tue Jul 12 2022 14:13:52 by
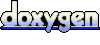