CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_biquad_cascade_df2T_init_f32.c
00001 /*----------------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_biquad_cascade_df2T_init_f32.c 00009 * 00010 * Description: Initialization function for the floating-point transposed 00011 * direct form II Biquad cascade filter. 00012 * 00013 * Target Processor: Cortex-M4/Cortex-M3 00014 * 00015 * Version 1.0.3 2010/11/29 00016 * Re-organized the CMSIS folders and updated documentation. 00017 * 00018 * Version 1.0.2 2010/11/11 00019 * Documentation updated. 00020 * 00021 * Version 1.0.1 2010/10/05 00022 * Production release and review comments incorporated. 00023 * 00024 * Version 1.0.0 2010/09/20 00025 * Production release and review comments incorporated 00026 * 00027 * Version 0.0.7 2010/06/10 00028 * Misra-C changes done 00029 * ---------------------------------------------------------------------------*/ 00030 00031 #include "arm_math.h" 00032 00033 /** 00034 * @ingroup groupFilters 00035 */ 00036 00037 /** 00038 * @addtogroup BiquadCascadeDF2T 00039 * @{ 00040 */ 00041 00042 /** 00043 * @brief Initialization function for the floating-point transposed direct form II Biquad cascade filter. 00044 * @param[in,out] *S points to an instance of the filter data structure. 00045 * @param[in] numStages number of 2nd order stages in the filter. 00046 * @param[in] *pCoeffs points to the filter coefficients. 00047 * @param[in] *pState points to the state buffer. 00048 * @return none 00049 * 00050 * <b>Coefficient and State Ordering:</b> 00051 * \par 00052 * The coefficients are stored in the array <code>pCoeffs</code> in the following order: 00053 * <pre> 00054 * {b10, b11, b12, a11, a12, b20, b21, b22, a21, a22, ...} 00055 * </pre> 00056 * 00057 * \par 00058 * where <code>b1x</code> and <code>a1x</code> are the coefficients for the first stage, 00059 * <code>b2x</code> and <code>a2x</code> are the coefficients for the second stage, 00060 * and so on. The <code>pCoeffs</code> array contains a total of <code>5*numStages</code> values. 00061 * 00062 * \par 00063 * The <code>pState</code> is a pointer to state array. 00064 * Each Biquad stage has 2 state variables <code>d1,</code> and <code>d2</code>. 00065 * The 2 state variables for stage 1 are first, then the 2 state variables for stage 2, and so on. 00066 * The state array has a total length of <code>2*numStages</code> values. 00067 * The state variables are updated after each block of data is processed; the coefficients are untouched. 00068 */ 00069 00070 void arm_biquad_cascade_df2T_init_f32( 00071 arm_biquad_cascade_df2T_instance_f32 * S, 00072 uint8_t numStages, 00073 float32_t * pCoeffs, 00074 float32_t * pState) 00075 { 00076 /* Assign filter stages */ 00077 S->numStages = numStages; 00078 00079 /* Assign coefficient pointer */ 00080 S->pCoeffs = pCoeffs; 00081 00082 /* Clear state buffer and size is always 2 * numStages */ 00083 memset(pState, 0, (2u * (uint32_t) numStages) * sizeof(float32_t)); 00084 00085 /* Assign state pointer */ 00086 S->pState = pState; 00087 } 00088 00089 /** 00090 * @} end of BiquadCascadeDF2T group 00091 */
Generated on Tue Jul 12 2022 14:13:52 by
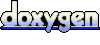