CMSIS DSP Library from CMSIS 2.0. See http://www.onarm.com/cmsis/ for full details
Dependents: K22F_DSP_Matrix_least_square BNO055-ELEC3810 1BNO055 ECE4180Project--Slave2 ... more
arm_biquad_cascade_df1_32x64_init_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 29. November 2010 00005 * $Revision: V1.0.3 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_biquad_cascade_df1_32x64_init_q31.c 00009 * 00010 * Description: High precision Q31 Biquad cascade filter initialization function. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3 00013 * 00014 * Version 1.0.3 2010/11/29 00015 * Re-organized the CMSIS folders and updated documentation. 00016 * 00017 * Version 1.0.2 2010/11/11 00018 * Documentation updated. 00019 * 00020 * Version 1.0.1 2010/10/05 00021 * Production release and review comments incorporated. 00022 * 00023 * Version 1.0.0 2010/09/20 00024 * Production release and review comments incorporated. 00025 * 00026 * Version 0.0.7 2010/06/10 00027 * Misra-C changes done 00028 * -------------------------------------------------------------------- */ 00029 00030 #include "arm_math.h" 00031 00032 /** 00033 * @ingroup groupFilters 00034 */ 00035 00036 /** 00037 * @addtogroup BiquadCascadeDF1_32x64 00038 * @{ 00039 */ 00040 00041 /** 00042 * @details 00043 * 00044 * @param[in,out] *S points to an instance of the high precision Q31 Biquad cascade filter structure. 00045 * @param[in] numStages number of 2nd order stages in the filter. 00046 * @param[in] *pCoeffs points to the filter coefficients. 00047 * @param[in] *pState points to the state buffer. 00048 * @param[in] postShift Shift to be applied after the accumulator. Varies according to the coefficients format. 00049 * @return none 00050 * 00051 * <b>Coefficient and State Ordering:</b> 00052 * 00053 * \par 00054 * The coefficients are stored in the array <code>pCoeffs</code> in the following order: 00055 * <pre> 00056 * {b10, b11, b12, a11, a12, b20, b21, b22, a21, a22, ...} 00057 * </pre> 00058 * where <code>b1x</code> and <code>a1x</code> are the coefficients for the first stage, 00059 * <code>b2x</code> and <code>a2x</code> are the coefficients for the second stage, 00060 * and so on. The <code>pCoeffs</code> array contains a total of <code>5*numStages</code> values. 00061 * 00062 * \par 00063 * The <code>pState</code> points to state variables array and size of each state variable is 1.63 format. 00064 * Each Biquad stage has 4 state variables <code>x[n-1], x[n-2], y[n-1],</code> and <code>y[n-2]</code>. 00065 * The state variables are arranged in the state array as: 00066 * <pre> 00067 * {x[n-1], x[n-2], y[n-1], y[n-2]} 00068 * </pre> 00069 * The 4 state variables for stage 1 are first, then the 4 state variables for stage 2, and so on. 00070 * The state array has a total length of <code>4*numStages</code> values. 00071 * The state variables are updated after each block of data is processed; the coefficients are untouched. 00072 */ 00073 00074 void arm_biquad_cas_df1_32x64_init_q31 ( 00075 arm_biquad_cas_df1_32x64_ins_q31 * S, 00076 uint8_t numStages, 00077 q31_t * pCoeffs, 00078 q63_t * pState, 00079 uint8_t postShift) 00080 { 00081 /* Assign filter stages */ 00082 S->numStages = numStages; 00083 00084 /* Assign postShift to be applied to the output */ 00085 S->postShift = postShift; 00086 00087 /* Assign coefficient pointer */ 00088 S->pCoeffs = pCoeffs; 00089 00090 /* Clear state buffer and size is always 4 * numStages */ 00091 memset(pState, 0, (4u * (uint32_t) numStages) * sizeof(q63_t)); 00092 00093 /* Assign state pointer */ 00094 S->pState = pState; 00095 } 00096 00097 /** 00098 * @} end of BiquadCascadeDF1_32x64 group 00099 */
Generated on Tue Jul 12 2022 14:13:52 by
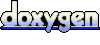