
Embed:
(wiki syntax)
Show/hide line numbers
Terminal.cpp
00001 /* mbed Terminal TextDisplay Library 00002 * Copyright (c) 2007-2009 sford 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 00006 #include "Terminal.h" 00007 00008 #include "mbed.h" 00009 00010 Terminal::Terminal(PinName tx, PinName rx) : _serial(tx, rx) { 00011 cls(); 00012 } 00013 00014 void Terminal::character(int column, int row, int c) { 00015 // Cursor Home <ESC>[{ROW};{COLUMN}H 00016 _serial.printf("\033[%d;%dH%c", row + 1, column + 1, c); 00017 } 00018 00019 int Terminal::columns() { 00020 return 80; 00021 } 00022 00023 int Terminal::rows() { 00024 return 35; 00025 } 00026 00027 void Terminal::cls() { 00028 _serial.printf("\033[2J"); 00029 } 00030 00031 void Terminal::foreground(int colour) { 00032 00033 /* Set Attribute Mode <ESC>[{n}m 00034 * - Sets display attribute settings. The following lists standard attributes: 00035 * 00036 * Foreground Colours 00037 * 30 Black 00038 * 31 Red 00039 * 32 Green 00040 * 33 Yellow 00041 * 34 Blue 00042 * 35 Magenta 00043 * 36 Cyan 00044 * 37 White 00045 */ 00046 int r = (colour >> 23) & 1; 00047 int g = (colour >> 15) & 1; 00048 int b = (colour >> 7) & 1; 00049 int bgr = (b << 2) | (g << 1) | (r << 0); 00050 int c = 30 + bgr; 00051 _serial.printf("\033[%dm", c); 00052 } 00053 00054 void Terminal::background(int colour) { 00055 00056 /* Background Colours 00057 * 40 Black 00058 * 41 Red 00059 * 42 Green 00060 * 43 Yellow 00061 * 44 Blue 00062 * 45 Magenta 00063 * 46 Cyan 00064 * 47 White 00065 */ 00066 int r = (colour >> 23) & 1; 00067 int g = (colour >> 15) & 1; 00068 int b = (colour >> 7) & 1; 00069 int bgr = (b << 2) | (g << 1) | (r << 0); 00070 int c = 40 + bgr; 00071 _serial.printf("\033[%dm", c); 00072 } 00073
Generated on Sat Jul 16 2022 07:20:35 by
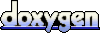