ID12RFID library, quickly modified to use interrupt driven reception instead so it doesn\'t block
Dependents: m3pi_WiiRacing m3pi_RFIDReader
ID12RFID.cpp
00001 /* mbed ID12 RFID Library 00002 * Copyright (c) 2007-2011, sford, http://mbed.org 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 // modified to use interrupts 00024 00025 #include "ID12RFID.h" 00026 00027 #include "mbed.h" 00028 00029 ID12RFID::ID12RFID(PinName rx) 00030 : _rfid(NC, rx) { 00031 nbuffer = 0; 00032 last = 0; 00033 _rfid.attach(this, &ID12RFID::handler); 00034 } 00035 00036 void ID12RFID::handler() { 00037 buffer[nbuffer] = _rfid.getc(); 00038 if(buffer == 0) { // waiting for '2' 00039 if(buffer[nbuffer] != 2) { // is not '2' 00040 return; // so ignore 00041 } 00042 } 00043 nbuffer++; 00044 00045 // 2 x x 8 chars x x x x 00046 if(nbuffer > 15) { 00047 last = 0; 00048 for(int i=7; i>=0; i--) { 00049 char c = buffer[10 - i]; // a ascii hex char 00050 int part = c - '0'; 00051 last |= part << (i * 4); 00052 } 00053 nbuffer = 0; 00054 } 00055 } 00056 00057 int ID12RFID::readable() { 00058 return (last > 0); //_rfid.readable(); 00059 } 00060 00061 int ID12RFID::read() { 00062 int t = last; 00063 last = 0; 00064 return t; 00065 }
Generated on Fri Jul 22 2022 11:12:21 by
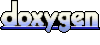