i2c driver for ITG3200 gyroscope sensor
Fork of ITG3200 by
Embed:
(wiki syntax)
Show/hide line numbers
ITG3200.cpp
00001 /** 00002 * @author Aaron Berk 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 ARM Limited 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * ITG-3200 triple axis, digital interface, gyroscope. 00029 * 00030 * Datasheet: 00031 * 00032 * http://invensense.com/mems/gyro/documents/PS-ITG-3200-00-01.4.pdf 00033 */ 00034 00035 /** 00036 * Includes 00037 */ 00038 #include "ITG3200.h" 00039 00040 ITG3200::ITG3200(I2C &i2c) : i2c_(i2c) { 00041 initialize(); 00042 } 00043 00044 ITG3200::ITG3200(PinName sda, PinName scl) : i2c_(sda, scl) { 00045 //400kHz, fast mode. 00046 //i2c_.frequency(400000); 00047 00048 initialize(); 00049 } 00050 00051 void ITG3200::initialize(){ 00052 //Set FS_SEL to 0x03 for proper operation. 00053 //See datasheet for details. 00054 char tx[2]; 00055 tx[0] = DLPF_FS_REG; 00056 //FS_SEL bits sit in bits 4 and 3 of DLPF_FS register. 00057 tx[1] = 0x03 << 3; 00058 00059 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00060 } 00061 00062 char ITG3200::getWhoAmI(void){ 00063 00064 //WhoAmI Register address. 00065 char tx = WHO_AM_I_REG; 00066 char rx; 00067 00068 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00069 00070 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00071 00072 return rx; 00073 00074 } 00075 00076 void ITG3200::setWhoAmI(char address){ 00077 00078 char tx[2]; 00079 tx[0] = WHO_AM_I_REG; 00080 tx[1] = address; 00081 00082 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00083 00084 } 00085 00086 char ITG3200::getSampleRateDivider(void){ 00087 00088 char tx = SMPLRT_DIV_REG; 00089 char rx; 00090 00091 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00092 00093 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00094 00095 return rx; 00096 00097 } 00098 00099 void ITG3200::setSampleRateDivider(char divider){ 00100 00101 char tx[2]; 00102 tx[0] = SMPLRT_DIV_REG; 00103 tx[1] = divider; 00104 00105 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00106 00107 } 00108 00109 int ITG3200::getInternalSampleRate(void){ 00110 00111 char tx = DLPF_FS_REG; 00112 char rx; 00113 00114 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00115 00116 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00117 00118 //DLPF_CFG == 0 -> sample rate = 8kHz. 00119 if(rx == 0){ 00120 return 8; 00121 } 00122 //DLPF_CFG = 1..7 -> sample rate = 1kHz. 00123 else if(rx >= 1 && rx <= 7){ 00124 return 1; 00125 } 00126 //DLPF_CFG = anything else -> something's wrong! 00127 else{ 00128 return -1; 00129 } 00130 00131 } 00132 00133 void ITG3200::setLpBandwidth(char bandwidth){ 00134 00135 char tx[2]; 00136 tx[0] = DLPF_FS_REG; 00137 //Bits 4,3 are required to be 0x03 for proper operation. 00138 tx[1] = bandwidth | (0x03 << 3); 00139 00140 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00141 00142 } 00143 00144 char ITG3200::getInterruptConfiguration(void){ 00145 00146 char tx = INT_CFG_REG; 00147 char rx; 00148 00149 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00150 00151 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00152 00153 return rx; 00154 00155 } 00156 00157 void ITG3200::setInterruptConfiguration(char config){ 00158 00159 char tx[2]; 00160 tx[0] = INT_CFG_REG; 00161 tx[1] = config; 00162 00163 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00164 00165 } 00166 00167 bool ITG3200::isPllReady(void){ 00168 00169 char tx = INT_STATUS; 00170 char rx; 00171 00172 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00173 00174 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00175 00176 //ITG_RDY bit is bit 4 of INT_STATUS register. 00177 if(rx & 0x04){ 00178 return true; 00179 } 00180 else{ 00181 return false; 00182 } 00183 00184 } 00185 00186 bool ITG3200::isRawDataReady(void){ 00187 00188 char tx = INT_STATUS; 00189 char rx; 00190 00191 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00192 00193 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00194 00195 //RAW_DATA_RDY bit is bit 1 of INT_STATUS register. 00196 if(rx & 0x01){ 00197 return true; 00198 } 00199 else{ 00200 return false; 00201 } 00202 00203 } 00204 00205 float ITG3200::getTemperature(void){ 00206 00207 char tx = TEMP_OUT_H_REG; 00208 char rx[2]; 00209 00210 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00211 00212 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, rx, 2); 00213 00214 int16_t temperature = ((int) rx[0] << 8) | ((int) rx[1]); 00215 //Offset = -35 degrees, 13200 counts. 280 counts/degrees C. 00216 return 35.0 + ((temperature + 13200)/280.0); 00217 00218 } 00219 00220 int ITG3200::getGyroX(void){ 00221 00222 char tx = GYRO_XOUT_H_REG; 00223 char rx[2]; 00224 00225 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00226 00227 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, rx, 2); 00228 00229 int16_t output = ((int) rx[0] << 8) | ((int) rx[1]); 00230 00231 return output; 00232 00233 } 00234 00235 int ITG3200::getGyroY(void){ 00236 00237 char tx = GYRO_YOUT_H_REG; 00238 char rx[2]; 00239 00240 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00241 00242 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, rx, 2); 00243 00244 int16_t output = ((int) rx[0] << 8) | ((int) rx[1]); 00245 00246 return output; 00247 00248 } 00249 00250 int ITG3200::getGyroZ(void){ 00251 00252 char tx = GYRO_ZOUT_H_REG; 00253 char rx[2]; 00254 00255 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00256 00257 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, rx, 2); 00258 00259 int16_t output = ((int) rx[0] << 8) | ((int) rx[1]); 00260 00261 return output; 00262 00263 } 00264 00265 char ITG3200::getPowerManagement(void){ 00266 00267 char tx = PWR_MGM_REG; 00268 char rx; 00269 00270 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00271 00272 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00273 00274 return rx; 00275 00276 } 00277 00278 void ITG3200::setPowerManagement(char config){ 00279 00280 char tx[2]; 00281 tx[0] = PWR_MGM_REG; 00282 tx[1] = config; 00283 00284 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00285 00286 }
Generated on Mon Jul 18 2022 04:50:06 by
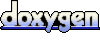