
DHT11 Temperature and humidity sensor using FRDM-K64f and Thingspeak
Dependencies: DHT ESP8266 mbed
main.cpp
00001 #include "mbed.h" 00002 #include "DHT.h" 00003 #include "ESP8266.h" 00004 00005 DHT sensor(D4, DHT11); 00006 ESP8266 wifi(PTC17, PTC16, 115200); 00007 Serial pc(USBTX,USBRX); 00008 DigitalOut RED(LED1); 00009 char snd[255],rcv[1000]; 00010 #define IP "184.106.153.149" // thingspeak.com IP Address 00011 void wifi_send(void); 00012 00013 int main() 00014 { 00015 pc.baud(115200); 00016 pc.printf("SET mode to AP\r\n"); 00017 wifi.SetMode(1); // set ESP mode to 1 00018 wifi.RcvReply(rcv, 1000); //receive a response from ESP 00019 pc.printf("%s",rcv); //Print the response onscreen 00020 pc.printf("Conneting to Wifi\r\n"); 00021 wifi.Join("ssid", "password"); // Your wifi username & Password 00022 wifi.RcvReply(rcv, 1000); //receive a response from ESP 00023 pc.printf("%s\n", rcv); //Print the response onscreen 00024 wait(8); //waits for response from ESP 00025 pc.printf("Getting IP\r\n"); //get IP addresss from the connected AP 00026 wifi.GetIP(rcv); //receive an IP address from the AP 00027 pc.printf("%s\n", rcv); 00028 while (1) 00029 { 00030 pc.printf("PLEASE STAY AWAY\r\n"); 00031 pc.printf("Sending WiFi information\n"); 00032 wifi_send(); 00033 RED=1; 00034 wait(2.0f); 00035 RED=0; 00036 wait(1.5f); 00037 } 00038 } 00039 void wifi_send(void){ 00040 int error = 0; 00041 float h = 0.0f, c = 0.0f; 00042 00043 wait(2.0f); 00044 error = sensor.readData(); 00045 if (0 == error) 00046 { 00047 c = sensor.ReadTemperature(CELCIUS); 00048 h = sensor.ReadHumidity(); 00049 //printf("Temperature in Celcius: %f\n", c); 00050 //printf("Humidity is %f\n", h); 00051 } 00052 else 00053 { 00054 printf("Error: %d\n", error); 00055 } 00056 //WIFI updates the Status to Thingspeak servers// 00057 strcpy(snd,"AT+CIPMUX=1\n");//Setting WiFi into MultiChannel mode 00058 wifi.SendCMD(snd); 00059 pc.printf(snd); 00060 wait(2.0); 00061 wifi.RcvReply(rcv, 1000); 00062 pc.printf("%s\n", rcv); 00063 wait(2); 00064 sprintf(snd,"AT+CIPSTART=4,\"TCP\",\"%s\",80\n",IP); //Initiate connection with THINGSPEAK server 00065 pc.printf(snd); 00066 wait(3.0); 00067 wifi.RcvReply(rcv, 1000); 00068 pc.printf("%s\n", rcv); 00069 wait(2); 00070 strcpy(snd,"AT+CIPSEND=4,47\n"); //Send Number of open connections,Characters to send 00071 wifi.SendCMD(snd); 00072 pc.printf(snd); 00073 wait(2.0); 00074 wifi.RcvReply(rcv, 1000); 00075 pc.printf("%s\n", rcv); 00076 wait(2); 00077 sprintf(snd,"GET http://api.thingspeak.com/update?api_key=**********&field1=%1.3f\n", c); //Post values to thingspeak 00078 pc.printf("%s",snd); 00079 wifi.SendCMD(snd); 00080 wait(2); 00081 sprintf(snd,"GET http://api.thingspeak.com/update?api_key=***********&field2=%1.3f\n", h); //Post values to thingspeak 00082 pc.printf("%s",snd); 00083 wifi.SendCMD(snd); 00084 wait(2); 00085 wifi.RcvReply(rcv, 1000); 00086 pc.printf("%s", rcv); 00087 wifi.SendCMD("AT+CIPCLOSE"); //Close the connection to server 00088 wifi.RcvReply(rcv, 1000); 00089 pc.printf("%s", rcv); 00090 }
Generated on Wed Jul 13 2022 19:41:26 by
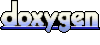