
Dummy program to demonstrate problems: working code
Dependencies: SLCD mbed-rtos mbed
Fork of MNG_TC by
compression.h
00001 int disk_write(const uint8_t *, uint64_t); 00002 uint64_t RTC_TIME; //need to be changed to uint_64 00003 00004 00005 namespace Science_TMframe { 00006 00007 #define OUTLENGTH 360 //length of the output frame after convolution 00008 #define SDcard_block 512 //block size of the sd card 00009 00010 Convolution ConvObj; //object which stores the frame after convolution 00011 bool fresh[3] = {true,true,true}; // True only for the first time 00012 unsigned char frames[3][134] = {0}; // "frame" stores the address of the current frame...."first_frame_address" stores the address of the first node made. 00013 unsigned int FCN[4] = {0}; //frame count number 00014 unsigned int data_starting_point[3] = {8,5,10}; 00015 unsigned int max_data[3] = {124,127,122}; //number of bytes in each frame excluding TMID,FCN,first_header_point,crc 00016 unsigned char TM_convoluted_data[270] = {0}; //270 bytes is the size after convolution of 1072 bits 00017 unsigned char complete_frame[SDcard_block] = {0}; 00018 uint64_t SDC_address = 10; 00019 bool SCH_FCCH_FLAG = true; 00020 00021 00022 void add_SCH_FCCH(){ 00023 int i = 0; 00024 complete_frame[0] = 0x0a; 00025 complete_frame[1] = 0x3f;; 00026 complete_frame[2] = 0x46; 00027 complete_frame[3] = 0xb4; 00028 complete_frame[4] = 0x00; 00029 00030 for(i = 149 ; i < 159 ; i ++){ 00031 complete_frame[i] = 0 ; 00032 } 00033 00034 complete_frame[159] = 0x0a; 00035 complete_frame[160] = 0x3f;; 00036 complete_frame[161] = 0x46; 00037 complete_frame[162] = 0xb4; 00038 complete_frame[163] = 0x00; 00039 00040 for(i = 308 ; i < 318 ; i ++){ 00041 complete_frame[i] = 0 ; 00042 } 00043 00044 } 00045 00046 void making_frameHeader(unsigned char TMID){ 00047 00048 unsigned char frame_type_identifier = 0; // not conform about the values , yet to be done 00049 frames[TMID][0] = (frame_type_identifier<<7) + ( (TMID + 1)<<3 ) + ( (FCN[TMID]>>24) & 0x7 ); //frame number should be less than 2^23 since 23 bits are assigned for that 00050 frames[TMID][1] = ((FCN[TMID]>>16) & 0xff ); 00051 frames[TMID][2] = ( (FCN[TMID]>>8 )& 0xff ); 00052 frames[TMID][3] = ( FCN[TMID] & 0xff ); // first bit for (frame identifier), next 4 for (TMID) and next 27 for FCN 00053 00054 if(TMID == 0){ 00055 frames[TMID][5] =( (RTC_TIME>>29) & 0xff ); 00056 frames[TMID][6] =( (RTC_TIME>>21) & 0xff ); 00057 frames[TMID][7] =( (RTC_TIME>>13) & 0xff ); 00058 00059 }else if(TMID == 2){ 00060 frames[TMID][5] =( (RTC_TIME>>32) & 0xff ); 00061 frames[TMID][6] =( (RTC_TIME>>24) & 0xff ); 00062 frames[TMID][7] =( (RTC_TIME>>16) & 0xff ); 00063 frames[TMID][8] =( (RTC_TIME>>8 ) & 0xff ); 00064 frames[TMID][9] =( (RTC_TIME ) & 0xff ); 00065 } 00066 00067 } 00068 00069 void convolution (unsigned char * ptr){ 00070 00071 ConvObj.convolutionEncode(ptr , TM_convoluted_data); 00072 ConvObj.convolutionEncode(ptr + 67, TM_convoluted_data + 135); 00073 00074 } 00075 00076 /* 00077 @brief : take the address of array of LCR or HCR and stores it into a frame 00078 @parameters: type->L or H , deprnding on wheather it is LCR or HCR respectively 00079 @return: nothing 00080 */ 00081 00082 // type 2 yet to be done 00083 void making_frame(unsigned char TMID ,unsigned char type, unsigned char* pointer){ 00084 00085 TMID--; //TMID goes from 1 to 3 , convinient to ue from 0 to 2 00086 static int frame_space_number[3] = {0}; //this variable represents the register number of the frame in which LCR or HCR data to be written not including header 00087 int packet_len = 0; 00088 int copy_count = 0 ; 00089 00090 switch(int(TMID)){ 00091 case 0: //SCP 00092 if(type == 'L'){ //below threshold 00093 packet_len = 22; 00094 } 00095 else if(type == 'H'){ //above threshold 00096 packet_len = 26; 00097 } 00098 break; 00099 00100 case 1: //SFP above threshold 00101 packet_len = 35; 00102 break; 00103 00104 case 2: //SFP below threshold 00105 packet_len = 23; 00106 break; 00107 } 00108 00109 if(SCH_FCCH_FLAG){ 00110 add_SCH_FCCH(); 00111 SCH_FCCH_FLAG = false; 00112 } 00113 00114 if(fresh[TMID]){ 00115 //welcome to first frame 00116 making_frameHeader(TMID); 00117 frames[TMID][4] = 0; 00118 fresh[TMID] = false; 00119 } 00120 00121 00122 while(copy_count < packet_len){ // 22 bytes is the size of the LCR 00123 frames[TMID][ frame_space_number[TMID] + data_starting_point[TMID] ]= *(pointer + copy_count); 00124 frame_space_number[TMID]++; 00125 copy_count++; 00126 if( frame_space_number[TMID] == max_data[TMID] ){ //frame space number can go from 0 to 126 as data is written from 0+5 to 126+5 00127 FCN[TMID]++; 00128 // convolution and save frame in the sd card 00129 00130 // copying crc in 132 and 133 00131 int temp_crc; 00132 temp_crc = CRC::crc16_gen(frames[TMID],132); 00133 frames[TMID][132] = temp_crc>>8; 00134 frames[TMID][133] = temp_crc & 0xff; 00135 00136 //convolution and interleaving 00137 convolution(frames[TMID]); 00138 interleave(TM_convoluted_data , complete_frame + 5); 00139 interleave(TM_convoluted_data+ 135,complete_frame + 164); 00140 SPI_mutex.lock(); 00141 disk_write(complete_frame , SDC_address); 00142 SPI_mutex.unlock(); 00143 SDC_address++; 00144 00145 00146 00147 //now save to the sd card TM_convoluted_data 00148 // std::bitset<8> b; 00149 // printf("\nthis is frame %d\n",TMID); //for printing frame 00150 // for(int j =0; j<134;j++){ 00151 // printf(" %d",frames[TMID][j]); 00152 //// b = frames[TMID][j]; 00153 //// cout<<b; 00154 // } 00155 00156 frame_space_number[TMID] = 0; 00157 making_frameHeader(TMID); 00158 frames[TMID][4]=packet_len - copy_count; 00159 //write time here also 00160 continue; 00161 } 00162 } 00163 00164 // printf("\nthis is frame %d\n",TMID); //for printing frame 00165 // for(int j =0; j<134;j++){ 00166 // printf(" %d",frames[TMID][j]); 00167 // } 00168 00169 } 00170 00171 00172 } 00173 00174 00175 00176 00177 00178 namespace Science_Data_Compression{ 00179 00180 # define PACKET_SEQUENCE_COUNT 1 //1 byte 00181 # define NUM_PROTON_BIN 17 //2 byte each 00182 # define NUM_ELECTRON_BIN 14 //2 byte each 00183 # define VETO 1 //2 byte 00184 # define FASTCHAIN 2 //4 byte each 00185 #define RAW_PACKET_LENGTH 73 //73 bytes 00186 00187 // #define PACKET_SEQ_COUNT 1 00188 // #define PROTON_BIN_SIZE 2 00189 // #define ELECTRON_BIN_SIZE 2 00190 // #define VETO 2 00191 // #define FAST_CHAIN 4 00192 00193 00194 /* 00195 @brief: read one uint16_t equivalent of first two chars from the stream. short int because 16 bits 00196 @param: pointer to the start of the short int 00197 @return: uint16_t 00198 */ 00199 00200 00201 unsigned int read_2byte(unsigned char* ptr){ 00202 unsigned int output = (unsigned int) *(ptr+1); 00203 output += ( (unsigned int)(*ptr) ) << 8; 00204 return output; 00205 } 00206 00207 /* 00208 @brief: read one int equivalent of first four chars from the stream. int because 32 bits 00209 @param: pointer to the start of the short int 00210 @return: unsigned int 00211 */ 00212 00213 unsigned int read_4byte(unsigned char* ptr){ 00214 unsigned int output = (unsigned int) *(ptr+3); 00215 output += (unsigned int)*(ptr+2)<<8; 00216 output += (unsigned int)*(ptr+1)<<16; 00217 output += (unsigned int)*(ptr)<<24; 00218 return output; 00219 } 00220 00221 unsigned int SFP_thresholds[35]={0 ,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100};//threashold values 00222 unsigned int SCP_thresholds[35]={0 ,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,0 ,0 ,0 }; 00223 unsigned int SFP_bin[35]; 00224 unsigned int SCP_bin[35]={0}; 00225 unsigned char SFP_outputBT[23]; //BT = below threshold 00226 unsigned char SFP_outputAT[35]; 00227 unsigned char SCP_outputLCR[22]; 00228 unsigned char SCP_outputHCR[26]; 00229 00230 00231 //********************************************************************************************************************************************************************** 00232 //lots of compression functions are listed below 00233 00234 00235 unsigned char SFP_compress4_BT(unsigned int input){ //for veto 00236 int de_4 = 0; 00237 unsigned char output; 00238 00239 if(input<= 3){ 00240 // DE = 0; 00241 output = 0x0; 00242 de_4 = 0; 00243 } 00244 else if(input <= 12){ 00245 // DE = 01; 00246 output = 0x1; 00247 de_4 = 2; 00248 } 00249 else if(input <= 48){ 00250 // DE = 10 00251 output = 0x2; 00252 de_4 = 4; 00253 } 00254 else { 00255 // DE = 11 00256 output = 0x3; 00257 de_4 = 6; 00258 } 00259 00260 unsigned short int temp = input >> de_4; 00261 output += (temp ) << 2; 00262 return output; 00263 } 00264 00265 00266 unsigned char SFP_compress5_BT(unsigned int input){ 00267 int de_4 = 0; //number by which bin value need to be shifted 00268 unsigned char output; 00269 00270 if(input <= 15){ 00271 // D = 0 [0] 00272 output = 0x0; 00273 de_4 = 0; 00274 } 00275 else if(input <= 60){ 00276 // D = 1 00277 output = 0x1; 00278 de_4 = 2; 00279 } 00280 00281 unsigned short int temp = input >> de_4; 00282 output += (temp ) << 1; 00283 00284 return output; 00285 }; 00286 00287 00288 unsigned char SFP_compress6_BT(unsigned int input){ 00289 int de_4 = 0;; 00290 unsigned char output; 00291 00292 if(input <= 31){ 00293 // E = 0 [0] 00294 output = 0x0; 00295 de_4 = 0; 00296 } 00297 else if(input <= 124){ 00298 // E = 1 00299 output = 0x1; 00300 de_4 = 2; 00301 } 00302 00303 00304 unsigned short int temp = input >> de_4; 00305 output += (temp ) << 1; 00306 00307 return output; 00308 }; 00309 00310 unsigned char SFP_compress7_AT(unsigned int input){ 00311 int de_4 = 0; 00312 unsigned char output; 00313 00314 if(input <= 31){ 00315 // DE = 00 [0] 00316 output = 0x0; 00317 de_4 = 0; 00318 } 00319 else if(input <= 124){ 00320 // DE = 01 [1] 00321 output = 0x1; 00322 de_4 = 2; 00323 } 00324 00325 else if(input <= 496){ 00326 // DE = 10 [2] 00327 output = 0x2; 00328 de_4 = 4; 00329 } 00330 00331 else if(input <= 1984){ 00332 // DE = 11 [3] 00333 output = 0x3; 00334 de_4 = 6; 00335 } 00336 00337 unsigned short int temp = input >> de_4; 00338 output += (temp ) << 2; 00339 00340 return output; 00341 00342 }; 00343 00344 00345 unsigned char SFP_compress8_AT(unsigned int input){ 00346 00347 int de_4 = 0;; 00348 unsigned char output; 00349 00350 if(input <= 63){ 00351 // DE = 00 [0] 00352 output = 0x0; 00353 de_4 = 0; 00354 } 00355 else if(input <= 252){ 00356 // DE = 01 [1] 00357 output = 0x1; 00358 de_4 = 2; 00359 } 00360 00361 else if(input <= 1008){ 00362 // DE = 10 [2] 00363 output = 0x2; 00364 de_4 = 4; 00365 } 00366 00367 else { 00368 // DE = 11 [3] 00369 output = 0x3; 00370 de_4 = 6; 00371 } 00372 00373 unsigned short int temp = input >> de_4; 00374 output += (temp ) << 2; 00375 00376 return output; 00377 }; 00378 00379 unsigned char SFP_compress5_AT(unsigned int input){ 00380 int de_4 = 0;; 00381 unsigned char output; 00382 00383 if(input <= 3){ 00384 // DE = 000 [0] 00385 output = 0x0; 00386 de_4 = 0; 00387 } 00388 else if(input <= 12){ 00389 // DE = 001 [1] 00390 output = 0x1; 00391 de_4 = 2; 00392 } 00393 00394 else if(input <= 48){ 00395 // DE = 010 [2] 00396 output = 0x2; 00397 de_4 = 4; 00398 } 00399 00400 else if(input <= 192) { 00401 // DE = 011 [3] 00402 output = 0x3; 00403 de_4 = 6; 00404 } 00405 00406 else if(input <= 768) { 00407 // DE = 100 [4] 00408 output = 0x4; 00409 de_4 = 8; 00410 } 00411 00412 else if(input <= 3072) { 00413 // DE = 101 [5] 00414 output = 0x5; 00415 de_4 = 10; 00416 } 00417 00418 else if(input <= 12288) { 00419 // DE = 110 [6] 00420 output = 0x6; 00421 de_4 = 12; 00422 } 00423 00424 else { 00425 // DE = 111 [7] 00426 output = 0x7; 00427 de_4 = 14; 00428 } 00429 00430 unsigned short int temp = input >> de_4; 00431 output += (temp ) << 3; 00432 00433 return output; 00434 } 00435 00436 unsigned char SFP_compress7FC_AT(unsigned int input){ // for fast chain above threshold 00437 int de_4 = 0;; 00438 unsigned char output; 00439 00440 if(input <= 15){ 00441 // DE = 000 [0] 00442 output = 0x0; 00443 de_4 = 0; 00444 } 00445 else if(input <= 60){ 00446 // DE = 001 [1] 00447 output = 0x1; 00448 de_4 = 2; 00449 } 00450 00451 else if(input <= 240){ 00452 // DE = 010 [2] 00453 output = 0x2; 00454 de_4 = 4; 00455 } 00456 00457 else if(input <= 960) { 00458 // DE = 011 [3] 00459 output = 0x3; 00460 de_4 = 6; 00461 } 00462 00463 else if(input <= 3840) { 00464 // DE = 100 [4] 00465 output = 0x4; 00466 de_4 = 8; 00467 } 00468 00469 else if(input <= 15360) { 00470 // DE = 101 [5] 00471 output = 0x5; 00472 de_4 = 10; 00473 } 00474 00475 else if(input <= 61440) { 00476 // DE = 110 [6] 00477 output = 0x6; 00478 de_4 = 12; 00479 } 00480 00481 else { 00482 // DE = 111 [7] 00483 output = 0x7; 00484 de_4 = 14; 00485 } 00486 00487 unsigned short int temp = input >> de_4; 00488 output += (temp ) << 3; 00489 00490 return output; 00491 } 00492 00493 00494 00495 //++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00496 unsigned char SCP_compress6(unsigned int input){ 00497 int ef_4; 00498 unsigned char output; 00499 00500 if(input <= 15){ 00501 // EF = 00 00502 output = 0x0; 00503 ef_4 = 0; 00504 } 00505 00506 else if(input <= 60 ){ 00507 // EF = 01 [1] 00508 output = 0x01; 00509 ef_4 = 2; 00510 } 00511 else if(input <= 240){ 00512 // EF = 10 [2] 00513 output = 0x02; 00514 ef_4 = 4; 00515 } 00516 else{ 00517 // EF = 11 [3] 00518 output = 0x03; 00519 ef_4 = 6; 00520 } 00521 00522 unsigned short int temp = input >> ef_4; 00523 output += (temp & 0xf) << 2; 00524 00525 return output; 00526 } 00527 00528 unsigned char SCP_compress5(unsigned int input){ 00529 00530 int de_4 = 0;; 00531 unsigned char output; 00532 00533 if(input <= 7){ 00534 // DE = 00 [0] 00535 output = 0x0; 00536 de_4 = 0; 00537 } 00538 00539 else if(input <= 28){ 00540 // DE = 01 [1] 00541 output = 0x01; 00542 de_4 = 2; 00543 } 00544 else if(input <= 112){ 00545 // DE = 10 [2] 00546 output = 0x02; 00547 de_4 = 4; 00548 } 00549 else{ 00550 // DE = 11 [3] 00551 output = 0x03; 00552 de_4 = 6; 00553 } 00554 00555 unsigned short int temp = input >> de_4; 00556 output += (temp & 0x7) << 2; 00557 00558 return output; 00559 } 00560 00561 unsigned char SCP_compress6h(unsigned int input) { 00562 00563 int ef_4; 00564 unsigned char output; 00565 00566 if(input <=7){ 00567 // EF = 000 [0] 00568 output = 0x00; 00569 ef_4 = 0; 00570 } 00571 00572 else if(input <=28){ 00573 // EF = 001 [1] 00574 output = 0x01; 00575 ef_4 = 2; 00576 } 00577 else if(input <= 112){ 00578 00579 // EF = 010 [2] 00580 output = 0x02; 00581 ef_4 = 4; 00582 } 00583 else if(input <= 448){ 00584 // EF = 011 [3] 00585 output = 0x03; 00586 ef_4 = 6; 00587 } 00588 else if(input <= 1792){ 00589 // EF = 100 [4] 00590 output = 0x04; 00591 ef_4 = 8; 00592 00593 } 00594 else if(input <= 7168){ 00595 // EF = 101 [5] 00596 output = 0x05; 00597 ef_4 = 10; 00598 00599 } 00600 else if(input <= 28672){ 00601 // EF = 110 [6] 00602 output = 0x06; 00603 ef_4 = 12; 00604 } 00605 else{ 00606 // EF = 111 [7] 00607 output = 0x07; 00608 ef_4 =14; 00609 } 00610 00611 unsigned short int temp = input >> ef_4; 00612 output += (temp & 0x7) << 3; 00613 00614 return output; 00615 00616 } 00617 00618 00619 unsigned char SCP_compress7h(unsigned int input) { 00620 00621 int fg_4; 00622 unsigned char output; 00623 00624 if(input <= 15){ 00625 // EF = 000 [0] 00626 output = 0x0; 00627 fg_4 = 0; 00628 } 00629 00630 else if(input <= 60){ 00631 // EF = 001 [1] 00632 output = 0x01; 00633 fg_4 = 2; 00634 } 00635 else if(input <= 240){ 00636 00637 // EF = 010 [2] 00638 output = 0x02; 00639 fg_4 = 4; 00640 } 00641 else if(input <= 960){ 00642 // EF = 011 [3] 00643 output = 0x03; 00644 fg_4 = 6; 00645 } 00646 else if(input <= 3840){ 00647 // EF = 100 [4] 00648 output = 0x04; 00649 fg_4 = 8; 00650 00651 } 00652 else if(input <= 15360){ 00653 // EF = 101 [5] 00654 output = 0x05; 00655 fg_4 = 10; 00656 00657 } 00658 else if(input <= 61440){ 00659 // EF = 110 [6] 00660 output = 0x06; 00661 fg_4 = 12; 00662 } 00663 else{ 00664 // EF = 111 [7] 00665 output = 0x07; 00666 fg_4 =14; 00667 } 00668 00669 unsigned short int temp = input >> fg_4; 00670 output += (temp & 0xf) << 3; 00671 00672 return output; 00673 00674 } 00675 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00676 void SCP_compress_data(); 00677 void SFP_compress_data(unsigned char* input){ 00678 00679 bool LCR = true; 00680 int i = 0; 00681 static int packet_no = 0; //takes value from 0 to 29 00682 //TRAVERSE THE LIST TO DETERMINE LCR OR HCR and stroing the values in proton_bin and electron bin 00683 SFP_bin[0] = *input; 00684 for(i=1 ; i<= NUM_PROTON_BIN + NUM_ELECTRON_BIN + VETO ; ++i){ //storing the bin values into an array name bin 00685 SFP_bin[i]=read_2byte(input+1+((i-1)<<1)); //proton bin and elecron bin are 2 byte, hence read_2byte and 00686 SCP_bin[i]+=SFP_bin[i]; 00687 if(SFP_bin[i] > SFP_thresholds[i]){ //fast cahin is 4 byte hence read_4byte 00688 LCR = false; // if a single value is above threshold then lcr becomes false 00689 i++; 00690 break; 00691 } 00692 } 00693 00694 for( ; i<= NUM_PROTON_BIN + NUM_ELECTRON_BIN + VETO ; ++i){ 00695 SCP_bin[i]+=SFP_bin[i]; 00696 SFP_bin[i] = read_2byte(input + 1 + ( (i-1)<<1) ); 00697 } 00698 00699 SFP_bin[i] = read_4byte(input+1+ ((i-1)<<1)) ; 00700 SCP_bin[i]+=SFP_bin[i]; 00701 00702 if(SFP_bin[i]>SFP_thresholds[i]) 00703 LCR = false; //since veto starts from location (input + 65) and (input + 69) 00704 00705 SFP_bin[i+1] = read_4byte(input+69); 00706 SCP_bin[i]+=SFP_bin[i]; 00707 00708 if(SFP_bin[i]>SFP_thresholds[i]) 00709 LCR = false; 00710 00711 00712 // printf("\n"); //for printing the sfp bin 00713 // for (i=0;i<35;i++){ 00714 // printf("sfp[%d] = %d",i,SFP_bin[i]); 00715 // } 00716 // printf("\n"); 00717 00718 if(LCR){ 00719 00720 SFP_outputBT[0] = (packet_no<<3) + ( SFP_compress5_BT(SFP_bin[1])>>2 ); 00721 SFP_outputBT[1] = ( SFP_compress5_BT(SFP_bin[1])<<6 ) + ( SFP_compress5_BT(SFP_bin[2])<<1 ) + ( SFP_compress5_BT(SFP_bin[3])>>4 ); 00722 SFP_outputBT[2] = ( SFP_compress5_BT(SFP_bin[3])<<4 ) + ( SFP_compress5_BT(SFP_bin[4])>>1 ); 00723 SFP_outputBT[3] = ( SFP_compress5_BT(SFP_bin[4])<<7 ) + ( SFP_compress5_BT(SFP_bin[5])<<2 ) + ( SFP_compress5_BT(SFP_bin[6])>>3 ); 00724 SFP_outputBT[4] = ( SFP_compress5_BT(SFP_bin[6])<<5 ) + ( SFP_compress5_BT(SFP_bin[7]) ); 00725 SFP_outputBT[5] = ( SFP_compress5_BT(SFP_bin[8])<<3 ) + ( SFP_compress5_BT(SFP_bin[9])>>2 ); 00726 SFP_outputBT[6] = ( SFP_compress5_BT(SFP_bin[9])<<6 ) + ( SFP_compress5_BT(SFP_bin[10])<<1 ) + ( SFP_compress5_BT(SFP_bin[11])>>4 ); 00727 SFP_outputBT[7] = ( SFP_compress5_BT(SFP_bin[11])<<4 ) + ( SFP_compress5_BT(SFP_bin[12])>>1 ); 00728 SFP_outputBT[8] = ( SFP_compress5_BT(SFP_bin[12])<<7 ) + ( SFP_compress5_BT(SFP_bin[13])<<2 ) + ( SFP_compress5_BT(SFP_bin[14])>>3 ); 00729 SFP_outputBT[9] = ( SFP_compress5_BT(SFP_bin[14])<<5 ) + ( SFP_compress5_BT(SFP_bin[15]) ); 00730 SFP_outputBT[10] = ( SFP_compress5_BT(SFP_bin[16])<<3 ) + ( SFP_compress5_BT(SFP_bin[17])>>2 ); 00731 SFP_outputBT[11] = ( SFP_compress5_BT(SFP_bin[17])<<6 ) + ( SFP_compress6_BT(SFP_bin[18]) ); 00732 SFP_outputBT[12] = ( SFP_compress6_BT(SFP_bin[19])<<2 ) + ( SFP_compress6_BT(SFP_bin[20])>>4 ); 00733 SFP_outputBT[13] = ( SFP_compress6_BT(SFP_bin[20])<<4 ) + ( SFP_compress5_BT(SFP_bin[21])>>1 ); 00734 SFP_outputBT[14] = ( SFP_compress5_BT(SFP_bin[21])<<7 ) + ( SFP_compress5_BT(SFP_bin[22])<<2 ) + ( SFP_compress5_BT(SFP_bin[23])>>3 ); 00735 SFP_outputBT[15] = ( SFP_compress5_BT(SFP_bin[23])<<5 ) + ( SFP_compress5_BT(SFP_bin[24]) ); 00736 SFP_outputBT[16] = ( SFP_compress5_BT(SFP_bin[25])<<3 ) + ( SFP_compress5_BT(SFP_bin[26])>>2 ); 00737 SFP_outputBT[17] = ( SFP_compress5_BT(SFP_bin[26])<<6 ) + ( SFP_compress5_BT(SFP_bin[27])<<1 ) + ( SFP_compress5_BT(SFP_bin[28])>>4); 00738 SFP_outputBT[18] = ( SFP_compress5_BT(SFP_bin[28])<<4 ) + ( SFP_compress5_BT(SFP_bin[29])>>1) ; 00739 SFP_outputBT[19] = ( SFP_compress5_BT(SFP_bin[29])<<7 ) + ( SFP_compress5_BT(SFP_bin[30])<<2 ) + ( SFP_compress5_BT(SFP_bin[31])>>3) ; 00740 SFP_outputBT[20] = ( SFP_compress5_BT(SFP_bin[31])<<5 ) + ( SFP_compress4_BT(SFP_bin[32])<<1) + ( SCP_compress5(SFP_bin[33])>>4 ) ; // 00741 SFP_outputBT[21] = ( SCP_compress5(SFP_bin[33])<<4 ) + ( SCP_compress5(SFP_bin[34])>>1 ); //here intentionally SCP_compress is used instead of SCP_compress5 is different than SFP_compress5 00742 SFP_outputBT[22] = ( SCP_compress5(SFP_bin[34])<<7 ); //7 bits are spare 00743 00744 Science_TMframe::making_frame(3,'L',SFP_outputBT); 00745 if(++packet_no == 30){ 00746 packet_no=0; 00747 SCP_compress_data(); 00748 for(i=0;i<PACKET_SEQUENCE_COUNT + NUM_PROTON_BIN + NUM_ELECTRON_BIN + VETO + FASTCHAIN;i++){ 00749 if(packet_no==0){ 00750 SCP_bin[i]=0; 00751 } 00752 } 00753 } 00754 00755 00756 } 00757 00758 else { 00759 00760 00761 SFP_outputAT[0] = (RTC_TIME>>27)&(0xff); 00762 SFP_outputAT[1] = (RTC_TIME>>19)&(0xff); 00763 SFP_outputAT[2] = (RTC_TIME>>11)&(0xff); 00764 SFP_outputAT[3] = (RTC_TIME>>3 )&(0xff); 00765 SFP_outputAT[4] = (RTC_TIME<<5 )&(0xff) + (packet_no); 00766 SFP_outputAT[5] = ( SFP_compress7_AT(SFP_bin[1])<<1 ) + ( SFP_compress7_AT(SFP_bin[2])>>6 ); 00767 SFP_outputAT[6] = ( SFP_compress7_AT(SFP_bin[2])<<2 ) + ( SFP_compress7_AT(SFP_bin[3])>>5 ); 00768 SFP_outputAT[7] = ( SFP_compress7_AT(SFP_bin[3])<<3 ) + ( SFP_compress7_AT(SFP_bin[4])>>4 ); 00769 SFP_outputAT[8] = ( SFP_compress7_AT(SFP_bin[4])<<4 ) + ( SFP_compress7_AT(SFP_bin[5])>>3 ); 00770 SFP_outputAT[9] = ( SFP_compress7_AT(SFP_bin[5])<<5 ) + ( SFP_compress7_AT(SFP_bin[6])>>2 ); 00771 SFP_outputAT[10] = ( SFP_compress7_AT(SFP_bin[6])<<6 ) + ( SFP_compress7_AT(SFP_bin[7])>>1 ); 00772 SFP_outputAT[11] = ( SFP_compress7_AT(SFP_bin[7])<<7 ) + ( SFP_compress7_AT(SFP_bin[8]) ); 00773 SFP_outputAT[12] = ( SFP_compress7_AT(SFP_bin[9])<<1 ) + ( SFP_compress7_AT(SFP_bin[10])>>6); 00774 SFP_outputAT[13] = ( SFP_compress7_AT(SFP_bin[10])<<2 ) + ( SFP_compress7_AT(SFP_bin[11])>>5); 00775 SFP_outputAT[14] = ( SFP_compress7_AT(SFP_bin[11])<<3 ) + ( SFP_compress7_AT(SFP_bin[12])>>4); 00776 SFP_outputAT[15] = ( SFP_compress7_AT(SFP_bin[12])<<4 ) + ( SFP_compress7_AT(SFP_bin[13])>>3); 00777 SFP_outputAT[16] = ( SFP_compress7_AT(SFP_bin[13])<<5 ) + ( SFP_compress7_AT(SFP_bin[14])>>2); 00778 SFP_outputAT[17] = ( SFP_compress7_AT(SFP_bin[14])<<6 ) + ( SFP_compress7_AT(SFP_bin[15])>>1); 00779 SFP_outputAT[18] = ( SFP_compress7_AT(SFP_bin[15])<<7 ) + ( SFP_compress7_AT(SFP_bin[16])); 00780 SFP_outputAT[19] = ( SFP_compress7_AT(SFP_bin[17])<<1 ) + ( SFP_compress8_AT(SFP_bin[18])>>7 ); 00781 SFP_outputAT[20] = ( SFP_compress8_AT(SFP_bin[18])<<1 ) + ( SFP_compress8_AT(SFP_bin[19])>>7 ); 00782 SFP_outputAT[21] = ( SFP_compress8_AT(SFP_bin[19])<<1 ) + ( SFP_compress8_AT(SFP_bin[20])>>7 ); 00783 SFP_outputAT[22] = ( SFP_compress8_AT(SFP_bin[20])<<1 ) + ( SFP_compress7_AT(SFP_bin[21])>>6 ); 00784 SFP_outputAT[23] = ( SFP_compress7_AT(SFP_bin[21])<<2 ) + ( SFP_compress7_AT(SFP_bin[22])>>5 ); 00785 SFP_outputAT[24] = ( SFP_compress7_AT(SFP_bin[22])<<3 ) + ( SFP_compress7_AT(SFP_bin[23])>>4 ); 00786 SFP_outputAT[25] = ( SFP_compress7_AT(SFP_bin[23])<<4 ) + ( SFP_compress7_AT(SFP_bin[24])>>3 ); 00787 SFP_outputAT[26] = ( SFP_compress7_AT(SFP_bin[24])<<5 ) + ( SFP_compress7_AT(SFP_bin[25])>>2 ); 00788 SFP_outputAT[27] = ( SFP_compress7_AT(SFP_bin[25])<<6 ) + ( SFP_compress7_AT(SFP_bin[26])>>1 ); 00789 SFP_outputAT[28] = ( SFP_compress7_AT(SFP_bin[26])<<7 ) + ( SFP_compress7_AT(SFP_bin[27]) ); 00790 SFP_outputAT[29] = ( SFP_compress7_AT(SFP_bin[28])<<1 ) + ( SFP_compress7_AT(SFP_bin[29])>>6); 00791 SFP_outputAT[30] = ( SFP_compress7_AT(SFP_bin[29])<<2 ) + ( SFP_compress7_AT(SFP_bin[30])>>5); 00792 SFP_outputAT[31] = ( SFP_compress7_AT(SFP_bin[30])<<3 ) +( SFP_compress7_AT(SFP_bin[31])>>4); 00793 SFP_outputAT[32] = ( SFP_compress7_AT(SFP_bin[31])<<4 ) +( SFP_compress5_AT(SFP_bin[32])>>1); 00794 SFP_outputAT[33] = ( SFP_compress5_AT(SFP_bin[32])<<7 ) +( SFP_compress7FC_AT(SFP_bin[33])); 00795 SFP_outputAT[34] = ( SFP_compress7FC_AT(SFP_bin[34])<<1 ); // 1 bit is spare 00796 00797 Science_TMframe::making_frame(2,'H',SFP_outputAT); 00798 if(++packet_no == 30){ 00799 packet_no=0; 00800 SCP_compress_data(); 00801 for(i=0;i<PACKET_SEQUENCE_COUNT + NUM_PROTON_BIN + NUM_ELECTRON_BIN + VETO + FASTCHAIN;i++){ 00802 if(packet_no==0){ 00803 SCP_bin[i]=0; 00804 } 00805 } 00806 } 00807 00808 } 00809 00810 } 00811 00812 00813 /* 00814 brief: takes the pointer of the raw data string and return the address of the array which stores the address of 30 packets. 00815 input: pointer to the raw data. 00816 output : void 00817 */ 00818 00819 void complete_compression(unsigned char *SRP,uint64_t x){ 00820 RTC_TIME = x; 00821 int i; //30 times because 3 second data 00822 00823 for(i=0;i<30;i++){ 00824 SFP_compress_data(SRP + 73*i); 00825 } 00826 00827 } 00828 00829 00830 00831 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00832 00833 00834 00835 /* 00836 @brief: compresses the given input stream and return output packet 00837 @param: pointer to input stream. Input stream always has the fixed size of RAW_PACKET_LENGTH 00838 @return: pointer to output stream. Output stream has the size of 22 or 26 bytes 00839 */ 00840 void SCP_compress_data(){ 00841 00842 bool LCR = true; 00843 int i = 0; 00844 00845 for(i=1;i<=PACKET_SEQUENCE_COUNT + NUM_PROTON_BIN + NUM_ELECTRON_BIN + VETO + FASTCHAIN ;i++){ 00846 if(SCP_bin[i]>SCP_thresholds[i]){ 00847 LCR = false; 00848 break; 00849 } 00850 } 00851 // printf("\n"); //for printing the scp bin 00852 // for (i=0;i<35;i++){ 00853 // printf(" scp[%d] = %d ",i,SCP_bin[i]); 00854 // } 00855 // printf("\n"); 00856 // compressing the data 00857 if(LCR){ 00858 SCP_outputLCR[0] = (RTC_TIME>>5)&(0xff); //first 13 bits for time tagging 00859 SCP_outputLCR[1] = (RTC_TIME<<3)&(0xff); //then 4 bits for attitude tag 00860 SCP_outputLCR[2] = 0x00; //only attitude tag is left 00861 SCP_outputLCR[2] += ( SCP_compress5(SCP_bin[0])<<1 ) + ( SCP_compress5(SCP_bin[1])>>4 ); 00862 SCP_outputLCR[3] = ( SCP_compress5(SCP_bin[1])<<4 ) + ( SCP_compress5(SCP_bin[2])>>1 ); 00863 SCP_outputLCR[4] = ( SCP_compress5(SCP_bin[2])<<7 ) + ( SCP_compress5(SCP_bin[3])<<2 ) + ( SCP_compress5(SCP_bin[4])>>3 ); 00864 SCP_outputLCR[5] = ( SCP_compress5(SCP_bin[4])<<5 ) + ( SCP_compress5(SCP_bin[5]) ); 00865 SCP_outputLCR[6] = ( SCP_compress5(SCP_bin[6])<<3 ) + ( SCP_compress5(SCP_bin[7])>>2 ); 00866 SCP_outputLCR[7] = ( SCP_compress5(SCP_bin[7])<<6 ) + ( SCP_compress5(SCP_bin[8])<<1 ) + ( SCP_compress5(SCP_bin[9])>>4 ); 00867 SCP_outputLCR[8] = ( SCP_compress5(SCP_bin[9])<<4 ) + ( SCP_compress5(SCP_bin[10])>>1 ); 00868 SCP_outputLCR[9] = ( SCP_compress5(SCP_bin[10])<<7 ) + ( SCP_compress5(SCP_bin[11])<<2) + ( SCP_compress5(SCP_bin[12])>>3 ); 00869 SCP_outputLCR[10] = ( SCP_compress5(SCP_bin[12])<<5 ) + ( SCP_compress5(SCP_bin[13]) ); 00870 SCP_outputLCR[11] = ( SCP_compress5(SCP_bin[14])<<3 ) + ( SCP_compress5(SCP_bin[15])>>2 ); 00871 SCP_outputLCR[12] = ( SCP_compress5(SCP_bin[15])<<6 ) + ( SCP_compress5(SCP_bin[16])<<1) + ( SCP_compress6(SCP_bin[17])>>5 ); 00872 SCP_outputLCR[13] = ( SCP_compress6(SCP_bin[17])<<3 ) + ( SCP_compress6(SCP_bin[18])>>3 ); 00873 SCP_outputLCR[14] = ( SCP_compress5(SCP_bin[18])<<5 ) + ( SCP_compress6(SCP_bin[19])>>1 ); 00874 SCP_outputLCR[15] = ( SCP_compress6(SCP_bin[19])<<7 ) + ( SCP_compress5(SCP_bin[20])<<2 ) + ( SCP_compress5(SCP_bin[21])>>3 ); 00875 SCP_outputLCR[16] = ( SCP_compress5(SCP_bin[21])<<5 ) + ( SCP_compress5(SCP_bin[22]) ); 00876 SCP_outputLCR[17] = ( SCP_compress5(SCP_bin[23])<<3 ) + ( SCP_compress5(SCP_bin[24])>>2 ); 00877 SCP_outputLCR[18] = ( SCP_compress5(SCP_bin[24])<<6 ) + ( SCP_compress5(SCP_bin[25])<<1) + ( SCP_compress5(SCP_bin[26])>>4 ); 00878 SCP_outputLCR[19] = ( SCP_compress5(SCP_bin[26])<<4 ) + ( SCP_compress5(SCP_bin[27])>>1 ); 00879 SCP_outputLCR[20] = ( SCP_compress5(SCP_bin[27])<<7 ) + ( SCP_compress5(SCP_bin[28])<<2 ) + ( SCP_compress5(SCP_bin[29])>>3 ); 00880 SCP_outputLCR[21] = ( SCP_compress5(SCP_bin[29])<<5 ) + ( SCP_compress5(SCP_bin[30]) ); 00881 00882 Science_TMframe::making_frame(1,'L',SCP_outputLCR); 00883 } 00884 else{ 00885 00886 SCP_outputLCR[0] = (RTC_TIME>>5)&(0xff); //first 13 bits for time tagging 00887 SCP_outputLCR[1] = (RTC_TIME<<3)&(0xff); 00888 SCP_outputHCR[2] = 0x40; 00889 SCP_outputHCR[2] += ( SCP_compress6h(SCP_bin[0])<<6 ) ; 00890 SCP_outputHCR[3] = ( SCP_compress6h(SCP_bin[1])<<2 ) + ( SCP_compress6h(SCP_bin[2])>>4) ; 00891 SCP_outputHCR[4] = ( SCP_compress6h(SCP_bin[2])<<4 ) + ( SCP_compress6h(SCP_bin[3])>>2) ; 00892 SCP_outputHCR[5] = ( SCP_compress6h(SCP_bin[3])<<6 ) + ( SCP_compress6h(SCP_bin[4])) ; 00893 SCP_outputHCR[6] = ( SCP_compress6h(SCP_bin[5])<<2 ) + ( SCP_compress6h(SCP_bin[6])>>4) ; 00894 SCP_outputHCR[7] = ( SCP_compress6h(SCP_bin[6])<<4 ) + ( SCP_compress6h(SCP_bin[7])>>2) ; 00895 SCP_outputHCR[8] = ( SCP_compress6h(SCP_bin[7])<<6 ) + ( SCP_compress6h(SCP_bin[8])) ; 00896 SCP_outputHCR[9] = ( SCP_compress6h(SCP_bin[9])<<2 ) + ( SCP_compress6h(SCP_bin[10])>>4) ; 00897 SCP_outputHCR[10] = ( SCP_compress6h(SCP_bin[10])<<4 ) + ( SCP_compress6h(SCP_bin[11])>>2); 00898 SCP_outputHCR[11] = ( SCP_compress6h(SCP_bin[11])<<6 ) + ( SCP_compress6h(SCP_bin[12])) ; 00899 SCP_outputHCR[12] = ( SCP_compress6h(SCP_bin[13])<<2 ) + ( SCP_compress6h(SCP_bin[14])>>4) ; 00900 SCP_outputHCR[13] = ( SCP_compress6h(SCP_bin[14])<<4 ) + ( SCP_compress6h(SCP_bin[15])>>2) ; 00901 SCP_outputHCR[14] = ( SCP_compress6h(SCP_bin[15])<<6 ) + ( SCP_compress6h(SCP_bin[16])) ; 00902 SCP_outputHCR[15] = ( SCP_compress7h(SCP_bin[17])<<1 ) + ( SCP_compress7h(SCP_bin[18])>>6) ; 00903 SCP_outputHCR[16] = ( SCP_compress7h(SCP_bin[18])<<2 ) + ( SCP_compress7h(SCP_bin[19])>>5) ; 00904 SCP_outputHCR[17] = ( SCP_compress7h(SCP_bin[19])<<3 ) + ( SCP_compress6h(SCP_bin[20])>>3) ; 00905 SCP_outputHCR[18] = ( SCP_compress6h(SCP_bin[20])<<5 ) + ( SCP_compress6h(SCP_bin[21])>>1) ; 00906 SCP_outputHCR[19] = ( SCP_compress6h(SCP_bin[21])<<7 ) + ( SCP_compress6h(SCP_bin[22])<<1) + ( SCP_compress6h(SCP_bin[23])>>5) ; 00907 SCP_outputHCR[20] = ( SCP_compress6h(SCP_bin[23])<<3 ) + ( SCP_compress6h(SCP_bin[24])>>3) ; 00908 SCP_outputHCR[21] = ( SCP_compress6h(SCP_bin[24])<<5 ) + ( SCP_compress6h(SCP_bin[25])>>1) ; 00909 SCP_outputHCR[22] = ( SCP_compress6h(SCP_bin[25])<<7 ) + ( SCP_compress6h(SCP_bin[26])<<1) + ( SCP_compress6h(SCP_bin[27])>>5) ; 00910 SCP_outputHCR[23] = ( SCP_compress6h(SCP_bin[27])<<3 ) + ( SCP_compress6h(SCP_bin[28])>>3) ; 00911 SCP_outputHCR[24] = ( SCP_compress6h(SCP_bin[28])<<5 ) + ( SCP_compress6h(SCP_bin[29])>>1) ; 00912 SCP_outputHCR[25] = ( SCP_compress6h(SCP_bin[29])<<7 ) + ( SCP_compress6h(SCP_bin[30])<<1) ; //last bit is empty 00913 00914 /* for (i=0;i<26;i++){ 00915 printf("\nscp[%d] = %d",i,SCP_outputHCR[i]); 00916 }*/ 00917 Science_TMframe::making_frame(1,'H',SCP_outputHCR); 00918 } 00919 } 00920 00921 } 00922
Generated on Thu Jul 21 2022 03:45:37 by
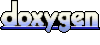