
Dummy program to demonstrate problems: working code
Dependencies: SLCD mbed-rtos mbed
Fork of MNG_TC by
MNG_TC.h
00001 // 8 Jul 00002 // did flowchart of states 00003 00004 // 7 Jul 00005 // done :decode should run once not every time tc received handle this 00006 // handle sd card with cdms team 00007 00008 // done :replace tc_list header with VAR_SPACE HEAD 00009 // done :delete data in rcv_tc 00010 // done :handle last tc in mbg and rcv_tc 00011 // done :delete all tc after pass 00012 00013 00014 //Jun 7 00015 //PROBLEM IN DELETING TC_string pointer not solved 00016 00017 // Jun 6 00018 // WHAT IS TC exec code in L1 ack ? 00019 // Removed class and introduced namespace 00020 00021 // Apil 15 00022 //added back printf statements 00023 //added back delete TC_string for debugging 00024 00025 // add number of tm packets while calling snd 00026 // function overloading z 00027 00028 // starting value of packet sequence count at each pass 00029 #define PSC_START_VALUE 1 00030 00031 // APID list 00032 #define APID_CALLSIGN 0 00033 #define APID_BAE 1 00034 #define APID_CDMS 2 00035 #define APID_SPEED 3 00036 00037 // HIGH PRIORITY TC - priority list 00038 // not correct values here 00039 #define HPTC1 5 00040 #define HPTC2 6 00041 // Add more entries above 00042 00043 // SIZE of tc in bytes 00044 #define TC_SHORT_SIZE 11 00045 #define TC_LONG_SIZE 135 00046 00047 // TMID list 00048 #define TMID_ACK_L1 10 00049 #define TM_SHORT_SIZE 13 00050 #define TM_TYPE1_SIZE 134 00051 00052 namespace MNG_TC 00053 { 00054 unsigned char psc = PSC_START_VALUE; 00055 unsigned int total_valid_TC = 0; 00056 bool all_crc_pass = true; 00057 bool no_missing_TC = true; 00058 unsigned int exec_count = 0; 00059 00060 //SECONDARY FUNCTIONS : [SHOULD NOT BE CALLED INDEPENDENTLY] 00061 //USED BY MEMBER FUNCTIONS FOUND BELOW 00062 00063 namespace L1_ACK{ 00064 00065 void generate_L1_ack_TM(TM_list *tm_ptr){ 00066 tm_ptr->next_TM = NULL; 00067 tm_ptr->TM_string = new unsigned char[TM_SHORT_SIZE]; 00068 // TMID 00069 tm_ptr->tmid = 0xA; 00070 tm_ptr->TM_string[0] = 0xaf; 00071 } 00072 00073 void execode_crc(bool all_pass, TM_list *tm_ptr){ 00074 00075 tm_ptr->TM_string[1] = ( all_crc_pass == true ) ? 0x01 : 0x00 ; 00076 00077 uint16_t crc_checksum = CRC::crc16_gen(tm_ptr->TM_string, TM_SHORT_SIZE-2); 00078 00079 tm_ptr->TM_string[TM_SHORT_SIZE-2] = (crc_checksum >> 8) & 0xff; 00080 tm_ptr->TM_string[TM_SHORT_SIZE-1] = crc_checksum & 0xff; 00081 } 00082 } 00083 00084 namespace EXECUTION{ 00085 00086 TM_list* Manage_CDMS(TC_list *ptr_tc){ 00087 00088 // DUMMY PROGRAM TO CREATE A SAMPLE TM 00089 00090 // allocate memory for new tm list 00091 TM_list *test_TM = new TM_list; 00092 00093 test_TM->next_TM = NULL; 00094 00095 // allocate memory for the tm string 00096 unsigned char *str = new unsigned char[TM_TYPE1_SIZE]; 00097 00098 00099 // frame type-1 is 0. [ (0 << 7) = 0 ] 00100 str[0] = 0; 00101 00102 // the tmid determines type-1, or 2 00103 // tmid : 0x1 belongs to type1 (dummy program) 00104 test_TM->tmid = 0x1; 00105 00106 // 4 bit TMID 00107 str[0] += (0x1) << 3; 00108 00109 // 20 bit seq. count 00110 str[0] += 0x7; 00111 str[1] = 0xff; 00112 str[2] = 0xff; 00113 00114 // random data 00115 for(int i = 3 ; i < (TM_TYPE1_SIZE-2) ; ++i ){ 00116 str[i] = 'a'; 00117 } 00118 00119 // APPEND CRC : ALL THE PROCESSES HAVE TO GENERATE AND APPEND CRC 00120 uint16_t crc_checksum = CRC::crc16_gen(str, TM_TYPE1_SIZE-2); 00121 str[TM_TYPE1_SIZE-2] = (crc_checksum >> 8) & 0xff; 00122 str[TM_TYPE1_SIZE-1] = crc_checksum & 0xff; 00123 00124 test_TM->TM_string = str; 00125 00126 return test_TM; 00127 } 00128 00129 // TM_list* RELAY(TC_list *tc_ptr){ 00130 // 00131 //// FIRST send long or short tc 00132 // unsigned char tc_len = ( tc_ptr->short_or_long ? TC_SHORT_SIZE : TC_LONG_SIZE ); 00133 // PC.putc( tc_len ); 00134 //// THE TARGET SHOULD READ 'n' MORE BYTES AS FOUND IN THE FIRST BYTE 00135 // 00136 //// SEND THE TC TO THE TARGET DEVICE [ALONG WITH CRC] 00137 // for(unsigned int i = 0 ; i < tc_len ; ++i){ 00138 // PC.putc( tc_ptr->TC_string[i] ); 00139 // } 00140 // 00141 //// WAIT FOR THE TM 00142 // TM_list* tm_head = new TM_list; 00143 // TM_list* tm_ptr = tm_head; 00144 // 00145 //// FIRST RECEIVE NUMBER OF TM'S TO BE RECEVIVED 00146 // unsigned char tm_num = PC.getc(); 00147 // for(unsigned int i = 0 ; i < tm_num ; ++i){ 00148 // 00149 //// THEN FOR EACH TM FIRST SEND TYPE-1 OR TYPE-2 i.e. NUMBER OF BYTES TO READ 00150 // unsigned char tm_len = PC.getc(); 00151 // tm_ptr->TM_string = new unsigned char[tm_len]; 00152 // for(unsigned int j = 0 ; j < tm_len ; ++j){ 00153 // tm_ptr->TM_string[j] = PC.getc(); 00154 // } 00155 // 00156 //// DECODE TMID FROM THE PACKET 00157 // if(tm_len == 134){ 00158 // unsigned char temp = tm_ptr->TM_string[0]; 00159 // tm_ptr->tmid = (temp >> 3) & 0xf; 00160 // } 00161 // else{ 00162 // unsigned char temp = tm_ptr->TM_string[4]; 00163 // tm_ptr->tmid = (temp >> 4) & 0xf; 00164 // } 00165 // 00166 //// ALLOCATE MEMORY FOR NEXT TM PACKET 00167 // if( i == tm_num-1 ){ 00168 // tm_ptr->next_TM = NULL; 00169 // } 00170 // else{ 00171 // tm_ptr->next_TM = new TM_list; 00172 // tm_ptr = tm_ptr->next_TM; 00173 // } 00174 // } 00175 // 00176 //// FILL IN THE tm_ptr AND RETURN 00177 // return tm_head; 00178 // } 00179 00180 bool detect_ack(TM_list *tm_ptr){ 00181 // printf("inside detect ack : "); 00182 if( tm_ptr != NULL ){ 00183 unsigned char tm_ack = tm_ptr->TM_string[3]; 00184 tm_ack &= 0xFF;//Ack or Nack can be decided just by checking [5:6] bits 00185 if( (tm_ack == 0xE0) || (tm_ack == 0xA0) || (tm_ack == 0xC0) ){ 00186 // printf("ack found\r\n"); 00187 return true; 00188 } 00189 else{ 00190 // printf("nack found\r\n"); 00191 return false; 00192 } 00193 } 00194 else{ 00195 // printf("nack null\r\n"); 00196 return false; 00197 } 00198 } 00199 00200 bool obosc_tc(TC_list *tc_ptr){ 00201 bool OBOSC = false; 00202 // check apid 00203 if(tc_ptr->apid == 2){ 00204 // check service type 00205 if( (tc_ptr->TC_string[2]) >> 4 == 0xB ){ 00206 // check service subtype 00207 switch( (tc_ptr->TC_string[2]) & 0xf ){ 00208 case 1: 00209 case 2: 00210 case 5: 00211 case 6: 00212 case 15: 00213 OBOSC = true; 00214 // printf("found obosc\r\n"); 00215 } 00216 } 00217 } 00218 00219 return OBOSC; 00220 } 00221 00222 TM_list* execute_obosc(TC_list *tc_ptr){ 00223 // printf("inside execute obosc\r\n"); 00224 unsigned char service_subtype = (tc_ptr->TC_string[2]) & 0x0F; 00225 unsigned char num = 0; 00226 unsigned char psc = 0x00; 00227 switch( service_subtype ){ 00228 case 0x01: 00229 // disable tc 00230 num = tc_ptr->TC_string[4]; 00231 psc = tc_ptr->TC_string[3]; 00232 00233 for(int i = 0 ; i < num ; ++i){ 00234 TC_list *tcp = VAR_SPACE::Head_node; 00235 while( tcp != NULL ){ 00236 if(tcp->packet_seq_count == psc){ 00237 tcp->enabled = false; 00238 ++psc; 00239 break; 00240 } 00241 } 00242 } 00243 break; 00244 case 0x02: 00245 // enable tc 00246 num = tc_ptr->TC_string[4]; 00247 psc = tc_ptr->TC_string[3]; 00248 00249 for(int i = 0 ; i < num ; ++i){ 00250 TC_list *tcp = VAR_SPACE::Head_node; 00251 while( tcp != NULL ){ 00252 if(tcp->packet_seq_count == psc){ 00253 tcp->enabled = true; 00254 ++psc; 00255 break; 00256 } 00257 } 00258 } 00259 break; 00260 case 0x05: 00261 // retry executin of tc 00262 psc = tc_ptr->TC_string[3]; 00263 00264 break; 00265 } 00266 // generate ackL234 00267 return NULL; 00268 } 00269 00270 bool sdCardOp(TC_list* tc_ptr){ 00271 bool readSD = false; 00272 00273 if(tc_ptr->apid == 2){ 00274 if( ( (tc_ptr->TC_string[2]) >> 4) == 0xF ){ 00275 switch( (tc_ptr->TC_string[2]) & 0xf ){ 00276 case 0: 00277 case 1: 00278 case 2: 00279 case 3: 00280 case 4: 00281 readSD = true; 00282 // printf("found sdcard op\r\n"); 00283 } 00284 } 00285 } 00286 return readSD; 00287 } 00288 00289 TM_list* CDMS_RLY_TMTC(TC_list* tc_ptr){ 00290 return NULL; 00291 } 00292 00293 bool execute_core(TC_list *tc_ptr){ 00294 printf("executing core psc = %u\r\n", psc); 00295 00296 if( !EXECUTION::sdCardOp(tc_ptr) ){ 00297 // printf("not sd card op\r\n"); 00298 TM_List *tm_ptr; 00299 00300 // call relay here 00301 tm_ptr = EXECUTION::CDMS_RLY_TMTC(tc_ptr); 00302 00303 // SEND DATA TO GS 00304 // snd_tm.head_pointer(tm_ptr); 00305 00306 // for rolling buffer : 00307 // send EoS only if TM to next tc has not arrived yet 00308 // else put the next TM itself. 00309 00310 if( EXECUTION::detect_ack(tm_ptr) ){ 00311 tc_ptr->exec_status = 1; 00312 } 00313 else{ 00314 tc_ptr->exec_status = 2; 00315 tc_ptr->enabled = false; 00316 if( tc_ptr->abort_on_nack ){ 00317 return false; 00318 } 00319 } 00320 00321 // DELETE THE TM AFTER USE 00322 while(tm_ptr != NULL){ 00323 TM_list *temp = tm_ptr->next_TM; 00324 delete tm_ptr; 00325 tm_ptr = temp; 00326 } 00327 } 00328 else{ 00329 // write sd card code 00330 read_TC(tc_ptr); 00331 } 00332 return true; 00333 } 00334 } 00335 00336 // MEMBER FUNCTIONS 00337 00338 /* 00339 @brief: INITIALISE THE HEAD NODE AND RESET THE VARIABLES 00340 @param: TC_list *head : head node pointer 00341 @return: none 00342 */ 00343 void init(){ 00344 // printf("inside init\r\n"); 00345 total_valid_TC = 0; 00346 all_crc_pass = true; 00347 no_missing_TC = true; 00348 psc = PSC_START_VALUE; 00349 exec_count = 0; 00350 } 00351 00352 00353 /* 00354 @brief: DELETE THE CRC FAILED TC FROM THE LIST TO FREE-UP MEMORY AND UPDATE 00355 THE TOTAL VALID TC AND GENERATE L1_ACK_TM 00356 @param: none 00357 @return: none 00358 */ 00359 void start_with(){ 00360 printf("inside start with\r\n"); 00361 TC_list *current_TC = VAR_SPACE::Head_node; 00362 00363 total_valid_TC = 0; 00364 all_crc_pass = true; 00365 00366 TM_List *l1_ack = new TM_List; 00367 TM_List *l1_ack_head = l1_ack; 00368 L1_ACK::generate_L1_ack_TM(l1_ack); 00369 00370 int TC_count = 0; 00371 // printf("outside while in start with\r\n"); 00372 // TC_list *zing = VAR_SPACE::Head_node; 00373 // while(zing != NULL){ 00374 //// printf("packet seq count = %u\r\n", zing->packet_seq_count); 00375 // zing = zing->next_TC; 00376 // } 00377 while(current_TC != NULL){ 00378 00379 unsigned char temp = 0; 00380 00381 // FILL PSC of the TC [ don't care whether crc pass or fail ] 00382 // PSC starts from 4th byte 00383 l1_ack->TM_string[3+TC_count] = current_TC->TC_string[0]; 00384 00385 // IF CRC PASS 00386 if( current_TC->crc_pass ){ 00387 ++total_valid_TC; 00388 printf("correct start with: psc = %u, short = %u\r\n", current_TC->packet_seq_count, (current_TC->short_or_long) ? 1 : 0 ); 00389 00390 // set the crc pass field in TC_STATUS ??? 00391 temp = l1_ack->TM_string[2]; 00392 temp |= ( 1 << (7-TC_count) ); 00393 l1_ack->TM_string[2] = temp; 00394 00395 // advance to the next node 00396 current_TC = current_TC->next_TC; 00397 } 00398 // if crc fail 00399 else{ 00400 printf("crc fail start with: psc = %u\r\n", current_TC->packet_seq_count); 00401 // unset the crc pass field in TC_STATUS 00402 temp = l1_ack->TM_string[2]; 00403 temp &= ~( 1 << (7-TC_count) ); 00404 l1_ack->TM_string[2] = temp; 00405 00406 current_TC = current_TC->next_TC; 00407 all_crc_pass = false; 00408 } 00409 ++TC_count; 00410 00411 // extend the TM linked list if TC_count > 7 00412 if(TC_count > 7){ 00413 TC_count = 0; 00414 00415 l1_ack->next_TM = new TM_List; 00416 l1_ack = l1_ack->next_TM; 00417 L1_ACK::generate_L1_ack_TM(l1_ack); 00418 00419 // FILL TC_EXEC_CODE 00420 // APPEND CRC TO THE TM 00421 L1_ACK::execode_crc( all_crc_pass, l1_ack ); 00422 } 00423 } 00424 00425 // FILL UP THE REMAINING FIELDS WITH ZEROS 00426 while(TC_count < 8){ 00427 l1_ack->TM_string[2] &= ~( 1 << (7-TC_count) ); 00428 // l1_ack->TM_string[3+TC_count] = 0; 00429 l1_ack->TM_string[3+TC_count] = 0x01; 00430 ++TC_count; 00431 } 00432 00433 // FILL TC_EXEC_CODE 00434 // APPEND CRC TO THE TM 00435 L1_ACK::execode_crc(all_crc_pass, l1_ack); 00436 00437 printf("Sending\r\n"); 00438 snd_tm.head_pointer(l1_ack_head); 00439 printf("Sent\r\n"); 00440 00441 // delete the TM 00442 l1_ack = l1_ack_head; 00443 while(l1_ack != NULL){ 00444 TM_List *temp = l1_ack->next_TM; 00445 00446 // print 00447 for(int i = 0 ; i < 13 ; ++i){ 00448 std::bitset<8> b = l1_ack->TM_string[i]; 00449 cout << b << " "; 00450 } 00451 cout << ENDL; 00452 00453 delete l1_ack; 00454 l1_ack = temp; 00455 } 00456 printf("finished start_with()\r\n"); 00457 } 00458 00459 /* 00460 @brief: check for missing tc, also check crc, i.e. 00461 if true execution can be started else have to wait 00462 @param: none 00463 @return: bool indicating whether there are missing tc 00464 */ 00465 bool check_for_missing_TC(void){ 00466 printf("checking for missing tc\r\n"); 00467 no_missing_TC = true; 00468 00469 // printf("total valid = %u\r\n", total_valid_TC); 00470 00471 for(unsigned char p = PSC_START_VALUE ; p < (total_valid_TC + PSC_START_VALUE) ; ++p){ 00472 bool flag = false; 00473 TC_list *node_ptr = VAR_SPACE::Head_node; 00474 // printf("checking for psc = %u\r\n", p); 00475 00476 while(node_ptr != NULL){ 00477 // printf("packet seq count = %u\t", node_ptr->packet_seq_count); 00478 if( (node_ptr->packet_seq_count == p) && (node_ptr->crc_pass) ){ 00479 flag = true; 00480 break; 00481 } 00482 else{ 00483 node_ptr = node_ptr->next_TC; 00484 } 00485 } 00486 // printf("\r\n"); 00487 if(flag == false){ 00488 no_missing_TC = false; 00489 break; 00490 } 00491 } 00492 00493 printf("returning from check for missing tc : %u\r\n", no_missing_TC ? 1 : 0); 00494 if(no_missing_TC){ 00495 return true; 00496 } 00497 else{ 00498 return false; 00499 } 00500 } 00501 00502 00503 00504 /* 00505 RUN start_with() before running execute_TC() 00506 V1.0 00507 @brief: EXECUTE THE LIST OF TCs, WITH A SEQUENCE BASED ON PSC 00508 SEND THE TC TO THE TARGET AND WAIT FOR THE TM 00509 THEN FORWARD IT TO GS 00510 @param: none 00511 @return: none 00512 */ 00513 TC_list *Second_head = NULL; 00514 void execute_TC(){ 00515 // EXECUTE ACCORDING TO THE PSC VALUE 00516 printf("inside execute tc : total valid tc = %u, psc = %u\r\n", total_valid_TC, psc); 00517 while( psc < (total_valid_TC+PSC_START_VALUE) ){ 00518 // printf("Iterating : psc = %u\r\n", psc); 00519 // check for new tc received 00520 bool exec_flag_local = false; 00521 if( VAR_SPACE::new_tc_received ){ 00522 printf("inaside new tc rx in obosc\r\n"); 00523 VAR_SPACE::new_tc_received = false; 00524 00525 VAR_SPACE::data_node = VAR_SPACE::head_data; 00526 Second_head = VAR_SPACE::last_node; 00527 COM_RCV_TC::rx_rcv_tc(); 00528 Second_head = Second_head->next_TC; 00529 exec_flag_local = true; 00530 } 00531 else if( VAR_SPACE::execute_obosc ){ 00532 printf("execute obosc flag found\r\n"); 00533 exec_flag_local = true; 00534 } 00535 if( exec_flag_local ){ 00536 exec_flag_local = false; 00537 00538 start_with(); 00539 00540 if( !check_for_missing_TC() ){ 00541 VAR_SPACE::rx_state = 3; 00542 printf("exiting from obosc\r\n"); 00543 return; 00544 } 00545 else{ 00546 printf("executing obosc\r\n"); 00547 // no missing tc : execute urgent 00548 TC_list *tcp = Second_head; 00549 while( tcp != NULL ){ 00550 if( EXECUTION::obosc_tc(tcp) ){ 00551 TM_list *tm_ptr = EXECUTION::execute_obosc(tcp); 00552 // snd_tm.head_pointer(tm_ptr); 00553 if( EXECUTION::detect_ack(tm_ptr) ){ 00554 tcp->exec_status = 1; 00555 } 00556 else{ 00557 tcp->exec_status = 2; 00558 if( tcp->abort_on_nack ){ 00559 tcp->enabled = false; 00560 VAR_SPACE::rx_state = 0; 00561 printf("exiting from obosc due to abort on nack\r\n"); 00562 VAR_SPACE::rx_state = 0; 00563 return; 00564 } 00565 } 00566 } 00567 tcp = tcp->next_TC; 00568 } 00569 } 00570 VAR_SPACE::execute_obosc = false; 00571 VAR_SPACE::rx_state = 2; 00572 } 00573 00574 TC_list *tc_ptr = VAR_SPACE::Head_node; 00575 // FIND THE TC CORRESPONDING TO THE PSC VALUE 00576 while(tc_ptr != NULL){ 00577 // printf("in while : psc = %u\r\n", psc); 00578 if( (tc_ptr->packet_seq_count == psc) && (tc_ptr->crc_pass)){ 00579 // THE TC WITH THE REQUIRED PSC HAS BEEN FOUND, NOW EXECUTE 00580 00581 if( (tc_ptr->exec_status == 0) || ((tc_ptr->exec_status == 2) && (tc_ptr->enabled)) ){ 00582 // execute tc 00583 00584 ++exec_count; 00585 if( EXECUTION::execute_core(tc_ptr) ){ 00586 // THE TC WITH APPROPRIATE PSC IS EXECUTED, START OVER 00587 break; 00588 } 00589 else{ 00590 printf("returning due to abort on nack\r\n"); 00591 // do something for the unexecuted telecommands 00592 VAR_SPACE::rx_state = 0; 00593 return; 00594 } 00595 } 00596 00597 else{ 00598 // either (execution failed and disabled) or (successful executed) hence break 00599 break; 00600 } 00601 } 00602 tc_ptr = tc_ptr->next_TC; 00603 } 00604 ++psc; 00605 } 00606 VAR_SPACE::rx_state = 0; 00607 printf("exiting after successfully executing tc, total executed = %u\r\n", exec_count); 00608 } 00609 }
Generated on Thu Jul 21 2022 03:45:37 by
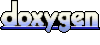