Basic Audio Signal Processing Library
Dependents: unzen_sample_nucleo_f746 skeleton_unzen_nucleo_f746 ifmag_noise_canceller synthesizer_f746
oscsincos.h
00001 namespace amakusa 00002 { 00003 /** 00004 * \brief Sine & Cosine Oscillator 00005 */ 00006 class OSCSinCos 00007 { 00008 public: 00009 /** 00010 * \brief constructor 00011 * \param freq Frequency in Hz. 00012 * \param Fs Sampling frequency in Hz. 00013 */ 00014 OSCSinCos( float freq, int Fs, int block_size ); 00015 /** 00016 * \brief wave generator method 00017 * \param c Pointer to the buffer to output the sine signal. 00018 * \param s Pointer to the buffer to output the cosine singal. If NULL, cosine signal will be skipped. 00019 * \params count Number of sample to be generated. 00020 */ 00021 void run( float *s, float *c ); 00022 /** 00023 * \brief wave generator method 00024 * \param c Pointer to the buffer to output the sine signal. 00025 * \params count Number of sample to be generated. 00026 */ 00027 void run( float *s ){run(s, 0);} 00028 /** 00029 * \brief set the oscillation furequncy. 00030 * \param freq Frequency in Hz. 00031 */ 00032 void setFrequency( float freq ); 00033 /** 00034 * \brief set the oscillator's internal phase 00035 * \param freq phase in radian. 00036 */ 00037 void setPhase( float phase ); 00038 /** 00039 * \brief get the oscillation furequncy. 00040 * \returns Frequency in Hz. 00041 */ 00042 float getFrequency(); 00043 /** 00044 * \brief get the internal phase 00045 * \returns Phase in radian 00046 */ 00047 float getPhase(); 00048 private: 00049 float deltaPhase; // deltaPhase per sample. 00050 int sampleFreq; // in Hz 00051 int phaseInSample; // internal phase [sample] 00052 int bs; // blockSize 00053 }; 00054 00055 } 00056
Generated on Tue Jul 12 2022 21:49:23 by
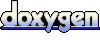