
Project
Dependencies: Hotboards_keypad TextLCD eeprom
Time.cpp
00001 #include "mbed.h" 00002 #include "Time.h" 00003 #include "LCDDisplay.h" 00004 #include <string> 00005 00006 int Date_Time_Setting(struct tm curt,char *str) 00007 { 00008 int len=0; 00009 len= strlen(str); 00010 // printf("len = %d",len); 00011 if(len < 14) 00012 return 0; 00013 00014 curt.tm_mday = ( str[0]-0x30)*10+(str[1]-0x30); 00015 curt.tm_mon = ( str[2]-0x30)*10+(str[3]-0x30) -1; 00016 curt.tm_year = (( str[4]-0x30)*1000 + (str[5]-0x30)*100 + (str[6]-0x30)*10 + (str[7]-0x30)) -1900 ; 00017 00018 curt.tm_hour=(str[8]-0x30)*10+(str[9]-0x30); 00019 curt.tm_min=(str[10]-0x30)*10+(str[11]-0x30); 00020 curt.tm_sec=(str[12]-0x30)*10+(str[13]-0x30); 00021 00022 time_t epoch = mktime(&curt); 00023 if (epoch == (time_t) -1) { 00024 error("Error in clock setting\n"); 00025 // Stop here 00026 } 00027 set_time(epoch); 00028 return 1; 00029 } 00030 00031 00032 int iSetTerminalTime(char *tstring) 00033 { 00034 int ret=0; 00035 struct tm curt; 00036 00037 ret = Date_Time_Setting(curt,tstring); 00038 00039 if(ret == 1) { 00040 wait(1); 00041 00042 Display_LCD(0,0," DATE-TIME "); 00043 Display_LCD(0,1," SET SUCCESS "); 00044 printf("Date Time Set succesfully\r\n"); 00045 00046 } else { 00047 Display_LCD(0,0," DATE-TIME "); 00048 Display_LCD(0,1," SET FAILURE "); 00049 printf("Date Time Set Failure \r\n"); 00050 00051 } 00052 wait(1); 00053 // Clear_LCD(); 00054 00055 return ret; 00056 } 00057 00058 00059 int chk_time (char *str) 00060 { 00061 int HH,MM,SS; 00062 HH=(str[0]-0x30)*10+(str[1]-0x30); 00063 MM=(str[2]-0x30)*10+(str[3]-0x30); 00064 SS=(str[4]-0x30)*10+(str[5]-0x30); 00065 if ( HH < 0 || HH > 23 || MM < 0 || MM > 59 || SS < 0 || SS > 59 ) 00066 return -1; 00067 return 1; 00068 } 00069 00070 int chk_date (char *str) 00071 { 00072 int epos_date=0,epos_month=0,epos_year=0; 00073 epos_date = ( str[0]-0x30)*10+(str[1]-0x30); 00074 epos_month = ( str[2]-0x30)*10+(str[3]-0x30); 00075 epos_year = ( str[4]-0x30)*1000+ (str[5]-0x30)*100 + (str[6]-0x30)*10 + (str[7]-0x30); 00076 00077 00078 if ( epos_month < 1 || epos_date < 1 || epos_date > 31 || epos_month > 12 || epos_year < 2008 ) return ERROR ; 00079 00080 else if(epos_month == 1 || epos_month == 3 || epos_month == 5 || epos_month == 7 || epos_month == 8 || epos_month ==10 ||epos_month == 12) { 00081 00082 if (epos_date > 31) 00083 return -1; 00084 } 00085 00086 else if (epos_month == 4 || epos_month == 6 || epos_month == 9 || epos_month == 11) { 00087 00088 if (epos_date > 30) 00089 return -1; 00090 } 00091 00092 else if (epos_month == 2 ) 00093 00094 { 00095 if ( !(epos_year%400) || (epos_year%100 != 0 && epos_year%4==0 ) ) { 00096 if (epos_date > 29 ) return -1; 00097 } 00098 00099 else if( epos_date > 28 ) return -1; 00100 } 00101 return 1; 00102 } 00103 00104 void Get_Date_Time(char *date_string,char *time_string, char *DTSTRING) 00105 { 00106 time_t curr_time; 00107 tm * curr_tm; 00108 time(&curr_time); 00109 curr_tm = localtime(&curr_time); 00110 strftime(date_string,10,"%Y%m%d",curr_tm); 00111 strftime(time_string,10,"%H%M%S",curr_tm); 00112 strftime(DTSTRING,20,"%Y%m%d%H%M%S",curr_tm); 00113 } 00114 00115 void Get_Date_Time(char *date_string,char *time_string) 00116 { 00117 time_t curr_time; 00118 tm * curr_tm; 00119 time(&curr_time); 00120 curr_tm = localtime(&curr_time); 00121 strftime(date_string,10,"%Y%m%d",curr_tm); 00122 strftime(time_string,10,"%H%M%S",curr_tm); 00123 00124 } 00125 00126 00127 void Get_Date_Time( char *DTSTRING) 00128 { 00129 time_t curr_time; 00130 tm * curr_tm; 00131 time(&curr_time); 00132 curr_tm = localtime(&curr_time); 00133 strftime(DTSTRING,20,"%Y%m%d%H%M%S",curr_tm); 00134 } 00135 00136 void Get_Date_Time_Trns( char *DTSTRING) 00137 { 00138 time_t curr_time; 00139 tm * curr_tm; 00140 time(&curr_time); 00141 curr_tm = localtime(&curr_time); 00142 strftime(DTSTRING,20,"%d%m%Y%H%M%S",curr_tm); 00143 } 00144 00145 00146 00147 int is_12_O_Clock_Night(void) 00148 { 00149 char dat[9]={'\0'}; 00150 char tim[9]={'\0'}; 00151 int HH,MM; 00152 Get_Date_Time(dat,tim); 00153 HH=(tim[0]-0x30)*10+(tim[1]-0x30); 00154 MM=(tim[2]-0x30)*10+(tim[3]-0x30); 00155 00156 if((HH==0) &&(MM <59)) 00157 { 00158 return 1; 00159 } 00160 else 00161 { 00162 return 0; 00163 } 00164 } 00165 00166 00167 int is_6_O_Clock(void) 00168 { 00169 char dat[9]={'\0'}; 00170 char tim[9]={'\0'}; 00171 int HH,MM; 00172 Get_Date_Time(dat,tim); 00173 HH=(tim[0]-0x30)*10+(tim[1]-0x30); 00174 MM=(tim[2]-0x30)*10+(tim[3]-0x30); 00175 00176 if((HH==6) &&(MM <30)) 00177 { 00178 return 1; 00179 } 00180 else 00181 { 00182 return 0; 00183 } 00184 }
Generated on Fri Jul 15 2022 15:37:46 by
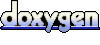