The tiny wav I/O module is the most simple wav file I/O module you've ever seen.
Dependents: SimpleWaveRecorderPlayer USBMSD_SD_HelloWorld_FRDM-KL25Z Application-SimpleWaveRecorderPlayerGenerator MbedClock ... more
wavfile.h
00001 /** 00002 * @file wavfile.h 00003 * @author Shinichiro Nakamura 00004 */ 00005 00006 /* 00007 * =============================================================== 00008 * Tiny WAV I/O Module 00009 * Version 0.0.1 00010 * =============================================================== 00011 * Copyright (c) 2011-2012 Shinichiro Nakamura 00012 * 00013 * Permission is hereby granted, free of charge, to any person 00014 * obtaining a copy of this software and associated documentation 00015 * files (the "Software"), to deal in the Software without 00016 * restriction, including without limitation the rights to use, 00017 * copy, modify, merge, publish, distribute, sublicense, and/or 00018 * sell copies of the Software, and to permit persons to whom the 00019 * Software is furnished to do so, subject to the following 00020 * conditions: 00021 * 00022 * The above copyright notice and this permission notice shall be 00023 * included in all copies or substantial portions of the Software. 00024 * 00025 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00026 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES 00027 * OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00028 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT 00029 * HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, 00030 * WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00031 * FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00032 * OTHER DEALINGS IN THE SOFTWARE. 00033 * =============================================================== 00034 */ 00035 00036 #ifndef WAVFILE_H 00037 #define WAVFILE_H 00038 00039 #include <stdio.h> 00040 #include <stdint.h> 00041 00042 #define WAVFILE_AUDIO_FORMAT_PCM (1) 00043 #define WAVFILE_AUDIO_FORMAT_EXTENSIBLE (65534) 00044 #define WAVFILE_MAXIMUM_CHANNELS (32) 00045 00046 #define WAVFILE_INFO_AUDIO_FORMAT(P) ((P)->audio_format) 00047 #define WAVFILE_INFO_NUM_CHANNELS(P) ((P)->num_channels) 00048 #define WAVFILE_INFO_SAMPLE_RATE(P) ((P)->sample_rate) 00049 #define WAVFILE_INFO_BYTE_RATE(P) ((P)->byte_rate) 00050 #define WAVFILE_INFO_BLOCK_ALIGN(P) ((P)->block_align) 00051 #define WAVFILE_INFO_BITS_PER_SAMPLE(P) ((P)->bits_per_sample) 00052 00053 #define WAVFILE_DATA_IS_END_OF_DATA(P) ((P)->num_channels == 0) 00054 #define WAVFILE_DATA_NUM_CHANNELS(P) ((P)->num_channels) 00055 #define WAVFILE_DATA_CHANNEL_DATA(P,CH) ((P)->channel_data[CH]) 00056 00057 #define WAVFILE_DEBUG_ENABLED (0) 00058 00059 typedef struct WAVFILE WAVFILE; 00060 00061 typedef struct { 00062 uint16_t audio_format; 00063 uint16_t num_channels; 00064 uint32_t sample_rate; 00065 uint32_t byte_rate; 00066 uint16_t block_align; 00067 uint16_t bits_per_sample; 00068 } wavfile_info_t; 00069 00070 typedef struct { 00071 uint16_t num_channels; 00072 double channel_data[WAVFILE_MAXIMUM_CHANNELS]; 00073 } wavfile_data_t; 00074 00075 /** 00076 */ 00077 enum WavFileResult { 00078 WavFileResultOK, 00079 WavFileResultErrorInvalidFileName, 00080 WavFileResultErrorMemoryAllocation, 00081 WavFileResultErrorFileOpen, 00082 WavFileResultErrorFileWrite, 00083 WavFileResultErrorBrokenChunkId, 00084 WavFileResultErrorBrokenChunkSize, 00085 WavFileResultErrorBrokenChunkData, 00086 WavFileResultErrorBrokenFormatId, 00087 WavFileResultErrorInvalidFormatId, 00088 WavFileResultErrorBrokenAudioFormat, 00089 WavFileResultErrorInvalidAudioFormat, 00090 WavFileResultErrorInvalidNumChannels, 00091 WavFileResultErrorBrokenNumChannels, 00092 WavFileResultErrorBrokenSampleRate, 00093 WavFileResultErrorBrokenByteRate, 00094 WavFileResultErrorInvalidByteRate, 00095 WavFileResultErrorBrokenBlockAlign, 00096 WavFileResultErrorBrokenBitsPerSample, 00097 WavFileResultErrorUnsupportedBitsPerSample, 00098 WavFileResultErrorAlreadyInfoChecked, 00099 WavFileResultErrorAlreadyDataChecked, 00100 WavFileResultErrorNoDataChunk, 00101 WavFileResultErrorInvalidMode, 00102 WavFileResultErrorNeedInfoChecked, 00103 WavFileResultErrorNeedDataChecked, 00104 WavFileResultErrorInvalidHandler, 00105 }; 00106 00107 /** 00108 */ 00109 enum WavFileMode { 00110 WavFileModeRead, 00111 WavFileModeWrite, 00112 }; 00113 00114 WAVFILE *wavfile_open(const char *filename, WavFileMode mode, WavFileResult *result); 00115 WavFileResult wavfile_read_info(WAVFILE *p, wavfile_info_t *info); 00116 WavFileResult wavfile_read_data(WAVFILE *p, wavfile_data_t *data); 00117 WavFileResult wavfile_write_info(WAVFILE *p, const wavfile_info_t *info); 00118 WavFileResult wavfile_write_data(WAVFILE *p, const wavfile_data_t *data); 00119 WavFileResult wavfile_close(WAVFILE *p); 00120 void wavfile_result_string(const WavFileResult result, char *buf, size_t siz); 00121 00122 #endif 00123
Generated on Wed Jul 13 2022 05:38:00 by
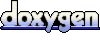