
http://mbed.org/users/shintamainjp/notebook/starboard_expbrd-one_ex1_en/
Dependencies: mbed RemoteIR SuperTweet ConfigFile EthernetNetIf
MyHomeLight.cpp
00001 /** 00002 * ============================================================================= 00003 * My home light controller. 00004 * http://mbed.org/users/shintamainjp/notebook/starboard_expbrd-one_ex1_en/ 00005 * ============================================================================= 00006 * Copyright (c) 2010 Shinichiro Nakamura (CuBeatSystems) 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * ============================================================================= 00026 */ 00027 00028 #include "MyHomeLight.h" 00029 #include "RemoteIR.h" 00030 00031 const MyHomeLight::light_signal_t MyHomeLight::lights[8] = { 00032 {0, "\x2C\x52\x09\x02\x08\x82"}, 00033 {1, "\x2C\x52\x09\x02\x0A\xA2"}, 00034 {2, "\x2C\x52\x09\x02\x0C\xC2"}, 00035 {3, "\x2C\x52\x09\x02\x0E\xE2"}, 00036 {4, "\x2C\x52\x09\x42\x08\xC2"}, 00037 {5, "\x2C\x52\x09\x42\x0A\xE2"}, 00038 {6, "\x2C\x52\x09\x42\x0C\x82"}, 00039 {7, "\x2C\x52\x09\x42\x0E\xA2"}, 00040 }; 00041 00042 /** 00043 * Create. 00044 * 00045 * @param tx_pin Pin of IR transmitter. 00046 */ 00047 MyHomeLight::MyHomeLight(PinName tx_pin) : tx(tx_pin) { 00048 } 00049 00050 /** 00051 * Dispose. 00052 */ 00053 MyHomeLight::~MyHomeLight() { 00054 } 00055 00056 /** 00057 * Toggle state. 00058 * 00059 * @param channel Target channel number. 00060 * @return true if it succeed. 00061 */ 00062 bool MyHomeLight::toggle(const int channel) { 00063 RemoteIR::Format fmt = RemoteIR::AEHA; 00064 uint8_t *sig = getLightSignal(channel); 00065 if (sig != NULL) { 00066 for (int i = 0; i < 2; i++) { 00067 while (tx.getState() != TransmitterIR::Idle) { 00068 wait_us(100); 00069 } 00070 tx.setData(fmt, sig, 48); 00071 wait_ms(120); 00072 } 00073 return true; 00074 } 00075 return false; 00076 } 00077 00078 /** 00079 * Get a signal for a light. 00080 * 00081 * @param channel Channel of a light. 00082 * 00083 * @return A pointer to a signal. 00084 */ 00085 uint8_t *MyHomeLight::getLightSignal(int channel) { 00086 const int n = sizeof(lights) / sizeof(lights[0]); 00087 if ((0 <= channel) && (channel <= n - 1)) { 00088 return (uint8_t *)lights[channel].signal; 00089 } 00090 return NULL; 00091 }
Generated on Fri Jul 15 2022 13:37:25 by
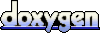