
A demonstration program for R/C servo I/F board. ( http://mbed.org/users/shintamainjp/notebook/starboard-orange_rcservo-ifbrd_en/ )
Dependencies: mbed TextLCD_SR4 NetServicesSource
main.cpp
00001 00002 #include "mbed.h" 00003 #include "EthernetNetIf.h" 00004 #include "HTTPServer.h" 00005 #include "TextLCD_SR4.h" 00006 00007 LocalFileSystem fs_local("local"); 00008 TextLCD_SR4 lcd(p29, p30, p28, p27); 00009 EthernetNetIf eth; 00010 HTTPServer svr; 00011 00012 int main() { 00013 Base::add_rpc_class<AnalogIn>(); 00014 Base::add_rpc_class<AnalogOut>(); 00015 Base::add_rpc_class<DigitalIn>(); 00016 Base::add_rpc_class<DigitalOut>(); 00017 Base::add_rpc_class<DigitalInOut>(); 00018 Base::add_rpc_class<PwmOut>(); 00019 Base::add_rpc_class<Timer>(); 00020 Base::add_rpc_class<BusOut>(); 00021 Base::add_rpc_class<BusIn>(); 00022 Base::add_rpc_class<BusInOut>(); 00023 Base::add_rpc_class<Serial>(); 00024 00025 lcd.cls(); 00026 lcd.printf("Setting up..."); 00027 EthernetErr ethErr = eth.setup(); 00028 if (ethErr) { 00029 lcd.cls(); 00030 lcd.printf("Error (%d)", ethErr); 00031 error("Error %d in setup.\n", ethErr); 00032 return -1; 00033 } else { 00034 IpAddr addr = eth.getIp(); 00035 lcd.cls(); 00036 lcd.printf("Network address"); 00037 lcd.locate(0, 1); 00038 lcd.printf("%3d.%3d.%3d.%3d", addr[0], addr[1], addr[2], addr[3]); 00039 } 00040 00041 FSHandler::mount("/local", "/"); //Mount /local path on web root path 00042 svr.addHandler<FSHandler>("/"); //Default handler 00043 svr.addHandler<RPCHandler>("/rpc"); 00044 svr.bind(80); 00045 00046 while (true) { 00047 Net::poll(); 00048 wait_ms(1); 00049 } 00050 00051 return 0; 00052 }
Generated on Wed Jul 13 2022 21:20:32 by
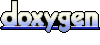