SerialGPS.
Dependents: SerialGPS_TestProgram Nucleo_SerialGPS_to_PC Nucleo_SerialGPS_to_PC SensorInterface ... more
SerialGPS.h
00001 /** 00002 * Serial GPS module interface driver class (Version 0.0.1) 00003 * This interface driver supports NMEA-0183 serial based modules. 00004 * 00005 * Copyright (C) 2010 Shinichiro Nakamura (CuBeatSystems) 00006 * http://shinta.main.jp/ 00007 */ 00008 #include "mbed.h" 00009 #include "SerialBuffered.h" 00010 00011 /** 00012 * Serial GPS module interface driver class (Version 0.0.1) 00013 * This interface driver supports NMEA-0183 serial based modules. 00014 * 00015 * = A list of NMEA-0183 Based GPS modules = 00016 * GT-720F : http://akizukidenshi.com/catalog/g/gM-02711/ 00017 * 00018 * = References = 00019 * NMEA Reference Manual (January 2005) - SiRF Technology, Inc. 00020 */ 00021 class SerialGPS { 00022 public: 00023 00024 /** 00025 * Create. 00026 * 00027 * @param tx A pin of transmit. 00028 * @param rx A pin of receive. 00029 * @param baud Baud rate. (Default = 9600) 00030 */ 00031 SerialGPS(PinName tx, PinName rx, int baud = 9600); 00032 00033 /** 00034 * Destroy. 00035 */ 00036 ~SerialGPS(); 00037 00038 /** 00039 * GGA - Global Positioning System Fixed Data. 00040 * 00041 * $GPGGA,161229.487,3723.2475,N,12158.3416,W,1,07,1.0,9.0,M, , , ,0000*18 00042 */ 00043 typedef struct { 00044 int hour; 00045 int min; 00046 int sec; 00047 double latitude; 00048 char ns; 00049 double longitude; 00050 char ew; 00051 int position_fix; 00052 int satellites_used; 00053 double hdop; 00054 int altitude; 00055 char altitude_unit; 00056 } gps_gga_t; 00057 00058 /** 00059 * GSA hGNSS DOP and Active Satellites. 00060 * 00061 * $GPGSA,A,3,07,02,26,27,09,04,15, , , , , ,1.8,1.0,1.5*33 00062 */ 00063 typedef struct { 00064 char selmode; 00065 int fix; 00066 } gps_gsa_t; 00067 00068 /** 00069 * for RMC: 00070 * Time, date, position, course and speed data. 00071 */ 00072 typedef struct { 00073 int hour; 00074 int min; 00075 int sec; 00076 char status; 00077 double nl; 00078 double el; 00079 } gps_rmc_t; 00080 00081 /** 00082 * for GSV: 00083 * The number of GPS satellites in view satellite ID numbers, 00084 * elevation, azimuth, and SNR values. 00085 */ 00086 typedef struct { 00087 int num; 00088 int elevation; 00089 int azimuth; 00090 int snr; 00091 } gps_gsv_satellite_t; 00092 00093 /** 00094 * for GSV: 00095 * The number of GPS satellites in view satellite ID numbers, 00096 * elevation, azimuth, and SNR values. 00097 */ 00098 typedef struct { 00099 int msgcnt; 00100 int msgnum; 00101 int satcnt; 00102 gps_gsv_satellite_t satellite[4]; 00103 } gps_gsv_t; 00104 00105 /** 00106 * Callback function structure. 00107 */ 00108 typedef struct { 00109 /** 00110 * A callback function for logging data. 00111 */ 00112 void (*cbfunc_log)(char *str); 00113 00114 /** 00115 * A callback function for GGA. 00116 * 00117 * GGA - Global Positioning System Fixed Data. 00118 */ 00119 void (*cbfunc_gga)(gps_gga_t *p); 00120 00121 /** 00122 * A callback function for GLL. 00123 * 00124 * GLL - Geographic Position - Latitude/Longitude. 00125 */ 00126 // TODO 00127 00128 /** 00129 * A callback function for GSA. 00130 * 00131 * GSA - GNSS DOP and Active Satellites. 00132 */ 00133 void (*cbfunc_gsa)(gps_gsa_t *p); 00134 00135 /** 00136 * A callback function for GSV. 00137 * 00138 * GSV - GNSS Satellites in View. 00139 */ 00140 void (*cbfunc_gsv)(gps_gsv_t *p); 00141 00142 /** 00143 * A callback function for MSS. 00144 * 00145 * MSS - MSK Receiver Signal. 00146 */ 00147 // TODO 00148 00149 /** 00150 * A callback function for RMC. 00151 * 00152 * RMC - Recommended Minimum Specific GNSS Data. 00153 */ 00154 void (*cbfunc_rmc)(gps_rmc_t *p); 00155 00156 /** 00157 * A callback function for VTG. 00158 * 00159 * VTG - Course Over Ground and Ground Speed. 00160 */ 00161 // TODO 00162 00163 /** 00164 * A callback function for ZDA. 00165 * 00166 * ZDA - SiRF Timing Message. 00167 */ 00168 // TODO 00169 00170 } gps_callback_t; 00171 00172 /** 00173 * Processing. 00174 */ 00175 bool processing(); 00176 00177 /** 00178 * Attach a callback function. 00179 * 00180 * @param cbfuncs A pointer to a call back function structure. 00181 */ 00182 void attach(gps_callback_t *cbfuncs); 00183 00184 /** 00185 * Detach a callback function. 00186 */ 00187 void detach(void); 00188 00189 private: 00190 SerialBuffered ser; 00191 gps_callback_t *cbfuncs; 00192 static const int PARAM_TXTMAXLEN = 64; 00193 static const int PARAM_ARRAYSIZE = 64; 00194 static bool exists(char c, char *buf) { 00195 const size_t n = strlen(buf); 00196 for (int i = 0; i < n; i++) { 00197 if (c == buf[i]) { 00198 return true; 00199 } 00200 } 00201 return false; 00202 } 00203 static char *parse(char *src, char *des, size_t siz, char *delim); 00204 static int parseAndCallbackGGA(char *src, gps_callback_t *cbfuncs); 00205 static int parseAndCallbackGSA(char *src, gps_callback_t *cbfuncs); 00206 static int parseAndCallbackRMC(char *src, gps_callback_t *cbfuncs); 00207 static int parseAndCallbackGSV(char *src, gps_callback_t *cbfuncs); 00208 static int parseAndCallbackUnknown(char *src, gps_callback_t *cbfuncs); 00209 static uint8_t calcCheckSum(char *buf, size_t siz); 00210 };
Generated on Thu Jul 14 2022 00:05:48 by
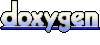