SG12864A
Dependents: SG12864A_TestProgram
SG12864A.h
00001 /** 00002 * SG12864A Graphics LCD module driver class (Version 0.0.1) 00003 * 00004 * Copyright (C) 2010 Shinichiro Nakamura (CuBeatSystems) 00005 * http://shinta.main.jp/ 00006 * 00007 * See also ... 00008 * http://mbed.org/users/shintamainjp/notebook/sg12864asunlike-display-graphics-lcd-driver/ 00009 */ 00010 00011 #ifndef _SG12864A_H_ 00012 #define _SG12864A_H_ 00013 00014 #include "mbed.h" 00015 00016 /** 00017 * SG12864A Graphics LCD module driver class. 00018 */ 00019 class SG12864A { 00020 public: 00021 00022 /** 00023 * Create. 00024 * 00025 * @param di D-/I (H:Instruction, L:Data) 00026 * @param rw R/W- (H:Read, L:Write) 00027 * @param en Enable signal 00028 * @param db0 Data bus line bit-0. 00029 * @param db1 Data bus line bit-1. 00030 * @param db2 Data bus line bit-2. 00031 * @param db3 Data bus line bit-3. 00032 * @param db4 Data bus line bit-4. 00033 * @param db5 Data bus line bit-5. 00034 * @param db6 Data bus line bit-6. 00035 * @param db7 Data bus line bit-7. 00036 * @param cs1 Chip select signal for IC1. 00037 * @param cs2 Chip select signal for IC2. 00038 * @param res Reset signal. 00039 */ 00040 SG12864A( 00041 PinName di, 00042 PinName rw, 00043 PinName en, 00044 PinName db0, 00045 PinName db1, 00046 PinName db2, 00047 PinName db3, 00048 PinName db4, 00049 PinName db5, 00050 PinName db6, 00051 PinName db7, 00052 PinName cs1, 00053 PinName cs2, 00054 PinName res); 00055 /** 00056 * Destroy. 00057 */ 00058 ~SG12864A(); 00059 00060 /** 00061 * Target of a chip. 00062 */ 00063 enum Target { 00064 CS1, 00065 CS2 00066 }; 00067 00068 /* 00069 * ======================================================= 00070 * High Level Interfaces. 00071 * ======================================================= 00072 */ 00073 00074 /** 00075 * Push images from a internal buffer. 00076 */ 00077 void bufferPush(void); 00078 00079 /** 00080 * Pull images to a internal buffer. 00081 */ 00082 void bufferPull(void); 00083 00084 /** 00085 * Clear a internal buffer images. 00086 * 00087 * @param reverse True if images are reversed. 00088 */ 00089 void bufferClear(bool reverse = false); 00090 00091 /** 00092 * Draw a line to a internal buffer. 00093 * 00094 * @param x1 Starting point at x-axis. 00095 * @param y1 Starting point at y-axis. 00096 * @param x2 Ending point at x-axis. 00097 * @param y2 Ending point at y-axis. 00098 * @param reverse True if images are reversed. 00099 */ 00100 void bufferDrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, bool reverse = false); 00101 00102 /** 00103 * Draw a box to a internal buffer. 00104 * 00105 * @param x1 Starting point at x-axis. 00106 * @param y1 Starting point at y-axis. 00107 * @param x2 Ending point at x-axis. 00108 * @param y2 Ending point at y-axis. 00109 * @param reverse True if images are reversed. 00110 */ 00111 void bufferDrawBox(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, bool reverse = false); 00112 00113 /** 00114 * Fill a box to a internal buffer. 00115 * 00116 * @param x1 Starting point at x-axis. 00117 * @param y1 Starting point at y-axis. 00118 * @param x2 Ending point at x-axis. 00119 * @param y2 Ending point at y-axis. 00120 * @param reverse True if images are reversed. 00121 */ 00122 void bufferFillBox(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, bool reverse = false); 00123 00124 /** 00125 * Draw a text string to a internal buffer. 00126 * The font size is 5x7 dots. 00127 * 00128 * @param x Starting point at x-axis. 00129 * @param y Starting point at y-axis. 00130 * @param str Text string. (NULL terminate.) 00131 * @param reverse True if images are reversed. 00132 */ 00133 void bufferDrawString(uint8_t x, uint8_t y, char * str, bool reverse = false); 00134 00135 /** 00136 * Draw a character to a internal buffer. 00137 * The font size is 5x7 dots. 00138 * 00139 * @param x Starting point at x-axis. 00140 * @param y Starting point at y-axis. 00141 * @param c A character. 00142 * @param reverse True if images are reversed. 00143 */ 00144 void bufferDrawChar(uint8_t x, uint8_t y, char c, bool reverse = false); 00145 00146 /** 00147 * Draw a checkbox to a internal buffer. 00148 * 00149 * @param x1 Starting point at x-axis. 00150 * @param y1 Starting point at y-axis. 00151 * @param x2 Ending point at x-axis. 00152 * @param y2 Ending point at y-axis. 00153 * @param state True if state is checked. 00154 * @param reverse True if images are reversed. 00155 */ 00156 void bufferDrawCheckbox(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, bool state, bool reverse = false); 00157 00158 /** 00159 * Draw a progressbar to a internal buffer. 00160 * 00161 * @param x1 Starting point at x-axis. 00162 * @param y1 Starting point at y-axis. 00163 * @param x2 Ending point at x-axis. 00164 * @param y2 Ending point at y-axis. 00165 * @param min Minimum value on a scale. 00166 * @param max Maximum value on a scale. 00167 * @param value Current value on a scale. 00168 * @param reverse True if images are reversed. 00169 */ 00170 void bufferDrawProgressbar(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, int min, int max, int value, bool reverse = false); 00171 00172 /* 00173 * High Level Interfaces. 00174 */ 00175 00176 /** 00177 * Reset module. 00178 */ 00179 void reset(void); 00180 00181 /** 00182 * Clear display. 00183 */ 00184 void clear(void); 00185 00186 /* 00187 * ======================================================= 00188 * Middle Level Interfaces. 00189 * ======================================================= 00190 */ 00191 00192 /** 00193 * Set display ON/OFF. 00194 * 00195 * @param t Target. 00196 * @param on True if the display is ON. 00197 */ 00198 void setDisplayOnOff(Target t, bool on); 00199 00200 /** 00201 * Set display start line. 00202 * 00203 * @param t Target. 00204 * @param displayStartLine Start line number. 00205 */ 00206 void setDisplayStartLine(Target t, uint8_t displayStartLine); 00207 00208 /** 00209 * Set page address. 00210 * 00211 * @param t Target. 00212 * @param addr Address. 00213 */ 00214 void setPageAddress(Target t, uint8_t addr); 00215 00216 /** 00217 * Set column address. 00218 * 00219 * @param t Target. 00220 * @param addr Address. 00221 */ 00222 void setColumnAddress(Target t, uint8_t addr); 00223 00224 /** 00225 * Read status. 00226 * 00227 * @param t Target. 00228 * @param c Status. 00229 */ 00230 void readStatus(Target t, uint8_t *c); 00231 00232 /** 00233 * Write data. 00234 * 00235 * @param t Target. 00236 * @param c Status. 00237 */ 00238 void writeData(Target t, uint8_t c); 00239 00240 /** 00241 * Read data. 00242 * 00243 * @param t Target. 00244 * @param c Status. 00245 */ 00246 void readData(Target t, uint8_t *c); 00247 00248 static const int PIXEL_X = 128; 00249 static const int PIXEL_Y = 64; 00250 static const uint8_t FONT_X = 5; 00251 static const uint8_t FONT_Y = 7; 00252 private: 00253 static const int PAGES = 8; 00254 static const int COLUMNS = 64; 00255 static const uint16_t FONT_MIN_CODE = 0x20; 00256 static const uint16_t FONT_MAX_CODE = 0x7F; 00257 static const uint8_t font5x7_data[]; 00258 uint8_t buffer[PAGES * COLUMNS * 2]; 00259 DigitalOut ioDI; 00260 DigitalOut ioRW; 00261 DigitalOut ioEN; 00262 BusInOut ioDB; 00263 DigitalOut ioCS1; 00264 DigitalOut ioCS2; 00265 DigitalOut ioRES; 00266 00267 /** 00268 * Mode of a write data. 00269 */ 00270 enum Mode { 00271 Data, 00272 Instruction 00273 }; 00274 00275 /* 00276 * ======================================================= 00277 * Low Level Interfaces. 00278 * ======================================================= 00279 */ 00280 00281 /** 00282 * Set I/O direction to read. 00283 */ 00284 void setDirectionToRead(); 00285 00286 /** 00287 * Set I/O direction to write. 00288 */ 00289 void setDirectionToWrite(); 00290 00291 /** 00292 * Write data. 00293 * 00294 * @param t Target. 00295 * @param m Mode. 00296 * @param c Data. 00297 */ 00298 void write(Target t, Mode m, uint8_t c); 00299 00300 /** 00301 * Read data. 00302 * 00303 * @param t Target. 00304 * @param m Mode. 00305 * @param c Data. 00306 */ 00307 void read(Target t, Mode m, uint8_t *c); 00308 00309 /** 00310 * Reset. 00311 * 00312 * @param b True if reset active. 00313 */ 00314 void reset(bool b); 00315 }; 00316 00317 #endif
Generated on Tue Jul 12 2022 11:03:55 by
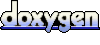