
This is Petit FAT File System Module for mbed NXP LPC1768. The ported library from http://elm-chan.org/fsw/ff/00index_p.html made by Mr. ChaN. The connection is same as SDCard library here http://mbed.org/projects/cookbook/wiki/SDCard . If you need change a pin, You can find the definition at libpff/connect.h :)
main.c
00001 /*---------------------------------------------------------------*/ 00002 /* Petit FAT file system module test program R0.02 (C)ChaN, 2009 */ 00003 /*---------------------------------------------------------------*/ 00004 00005 /* 00006 * Ported by Shinichiro Nakamura (CuBeatSystems) 00007 * http://shinta.main.jp/ 00008 * 2010/06/01 00009 */ 00010 00011 #include <string.h> 00012 #include "mbed.h" 00013 #include "libpff/diskio.h" 00014 #include "libpff/pff.h" 00015 00016 Serial pc(USBTX, USBRX); 00017 00018 char Line[128]; /* Console input buffer */ 00019 00020 static 00021 void put_rc (FRESULT rc) { 00022 const char *p; 00023 static const char str[] = 00024 "OK\0" "DISK_ERR\0" "NOT_READY\0" "NO_FILE\0" "NO_PATH\0" 00025 "NOT_OPENED\0" "NOT_ENABLED\0" "NO_FILE_SYSTEM\0"; 00026 uint8_t i; 00027 00028 for (p = str, i = 0; i != (uint8_t)rc && p; i++) { 00029 while (p++); 00030 } 00031 pc.printf("rc=%u FR_%S\n", (WORD)rc, p); 00032 } 00033 00034 00035 00036 static 00037 void put_drc (BYTE res) { 00038 pc.printf("rc=%d\n", res); 00039 } 00040 00041 00042 00043 static 00044 void get_line (char *buff, BYTE len) { 00045 BYTE c, i; 00046 00047 i = 0; 00048 for (;;) { 00049 c = pc.getc(); 00050 if (c == '\r') break; 00051 if ((c == '\b') && i) { 00052 i--; 00053 pc.putc('\b'); 00054 pc.putc(' '); 00055 pc.putc('\b'); 00056 } 00057 if ((c >= ' ') && (i < len - 1)) { 00058 buff[i++] = c; 00059 pc.putc(c); 00060 } 00061 } 00062 buff[i] = 0; 00063 pc.putc('\n'); 00064 } 00065 00066 00067 00068 static 00069 void put_dump (const BYTE *buff, DWORD ofs, int cnt) { 00070 BYTE n; 00071 00072 pc.printf("%08x", ofs); 00073 pc.putc(' '); 00074 for (n = 0; n < cnt; n++) { 00075 pc.putc(' '); 00076 pc.printf("%02x", buff[n]); 00077 } 00078 pc.printf(" "); 00079 for (n = 0; n < cnt; n++) { 00080 pc.putc(((buff[n] < 0x20)||(buff[n] >= 0x7F)) ? '.' : buff[n]); 00081 } 00082 pc.putc('\n'); 00083 } 00084 00085 00086 00087 /*-----------------------------------------------------------------------*/ 00088 /* Main */ 00089 00090 00091 int main (void) { 00092 char *ptr; 00093 long p1, p2; 00094 BYTE res; 00095 WORD s1, s2, s3, ofs, cnt, w; 00096 FATFS fs; /* File system object */ 00097 FATDIR dir; /* Directory object */ 00098 FILINFO fno; /* File information */ 00099 00100 pc.baud(19200); 00101 pc.printf("\nPFF test monitor\n"); 00102 00103 for (;;) { 00104 pc.putc('>'); 00105 get_line(Line, sizeof(Line)); 00106 ptr = Line; 00107 00108 switch (*ptr++) { 00109 case '?' : 00110 pc.printf("di - Initialize physical drive\n"); 00111 pc.printf("dd <sector> <ofs> - Dump partial secrtor 128 bytes\n"); 00112 pc.printf("fi - Mount the volume\n"); 00113 pc.printf("fo <file> - Open a file\n"); 00114 pc.printf("fd - Read the file 128 bytes and dump it\n"); 00115 pc.printf("fw <len> <val> - Write data to the file\n"); 00116 pc.printf("fp - Write console input to the file\n"); 00117 pc.printf("fe <ofs> - Move file pointer of the file\n"); 00118 pc.printf("fl [<path>] - Directory listing\n"); 00119 break; 00120 case 'd' : 00121 switch (*ptr++) { 00122 case 'i' : /* di - Initialize physical drive */ 00123 res = disk_initialize(); 00124 put_drc(res); 00125 break; 00126 case 'd' : /* dd <sector> <ofs> - Dump partial secrtor 128 bytes */ 00127 if (2 != sscanf(ptr, "%x %x", &p1, &p2)) { 00128 break; 00129 } 00130 s2 = p2; 00131 res = disk_readp((BYTE*)Line, p1, s2, 128); 00132 if (res) { 00133 put_drc(res); 00134 break; 00135 } 00136 s3 = s2 + 128; 00137 for (ptr = Line; s2 < s3; s2 += 16, ptr += 16, ofs += 16) { 00138 s1 = (s3 - s2 >= 16) ? 16 : s3 - s2; 00139 put_dump((BYTE*)ptr, s2, s1); 00140 } 00141 break; 00142 } 00143 break; 00144 case 'f' : 00145 switch (*ptr++) { 00146 00147 case 'i' : /* fi - Mount the volume */ 00148 put_rc(pf_mount(&fs)); 00149 break; 00150 00151 case 'o' : /* fo <file> - Open a file */ 00152 while (*ptr == ' ') ptr++; 00153 put_rc(pf_open(ptr)); 00154 break; 00155 #if _USE_READ 00156 case 'd' : /* fd - Read the file 128 bytes and dump it */ 00157 ofs = fs.fptr; 00158 res = pf_read(Line, sizeof(Line), &s1); 00159 if (res != FR_OK) { 00160 put_rc((FRESULT)res); 00161 break; 00162 } 00163 ptr = Line; 00164 while (s1) { 00165 s2 = (s1 >= 16) ? 16 : s1; 00166 s1 -= s2; 00167 put_dump((BYTE*)ptr, ofs, s2); 00168 ptr += 16; 00169 ofs += 16; 00170 } 00171 break; 00172 00173 #endif 00174 #if _USE_WRITE 00175 case 'w' : /* fw <len> <val> - Write data to the file */ 00176 if (2 != sscanf(ptr, "%x %x", &p1, &p2)) { 00177 break; 00178 } 00179 for (s1 = 0; s1 < sizeof(Line); Line[s1++] = (BYTE)p2) ; 00180 p2 = 0; 00181 while (p1) { 00182 if ((UINT)p1 >= sizeof(Line)) { 00183 cnt = sizeof(Line); 00184 p1 -= sizeof(Line); 00185 } else { 00186 cnt = (WORD)p1; 00187 p1 = 0; 00188 } 00189 res = pf_write(Line, cnt, &w); /* Write data to the file */ 00190 p2 += w; 00191 if (res != FR_OK) { 00192 put_rc((FRESULT)res); 00193 break; 00194 } 00195 if (cnt != w) break; 00196 } 00197 res = pf_write(0, 0, &w); /* Finalize the write process */ 00198 put_rc((FRESULT)res); 00199 if (res == FR_OK) 00200 pc.printf("%lu bytes written.\n", p2); 00201 break; 00202 case 'p' : /* fp - Write console input to the file */ 00203 pc.printf("Type any line to write. A blank line finalize the write operation.\n"); 00204 for (;;) { 00205 get_line(Line, sizeof(Line)); 00206 if (!Line[0]) break; 00207 strcat(Line, "\r\n"); 00208 res = pf_write(Line, strlen(Line), &w); /* Write a line to the file */ 00209 if (res) break; 00210 } 00211 res = pf_write(0, 0, &w); /* Finalize the write process */ 00212 put_rc((FRESULT)res); 00213 break; 00214 #endif 00215 #if _USE_LSEEK 00216 case 'e' : /* fe <ofs> - Move file pointer of the file */ 00217 if (1 != sscanf(ptr, "%x", &p1)) { 00218 break; 00219 } 00220 res = pf_lseek(p1); 00221 put_rc((FRESULT)res); 00222 if (res == FR_OK) { 00223 pc.printf("fptr = %lu(0x%lX)\n", fs.fptr, fs.fptr); 00224 } 00225 break; 00226 #endif 00227 #if _USE_DIR 00228 case 'l' : /* fl [<path>] - Directory listing */ 00229 while (*ptr == ' ') ptr++; 00230 res = pf_opendir(&dir, ptr); 00231 if (res) { 00232 put_rc((FRESULT)res); 00233 break; 00234 } 00235 s1 = 0; 00236 for (;;) { 00237 res = pf_readdir(&dir, &fno); 00238 if (res != FR_OK) { 00239 put_rc((FRESULT)res); 00240 break; 00241 } 00242 if (!fno.fname[0]) break; 00243 if (fno.fattrib & AM_DIR) 00244 pc.printf(" <DIR> %s\n", fno.fname); 00245 else 00246 pc.printf("%9lu %s\n", fno.fsize, fno.fname); 00247 s1++; 00248 } 00249 pc.printf("%u item(s)\n", s1); 00250 break; 00251 #endif 00252 } 00253 break; 00254 } 00255 } 00256 00257 } 00258 00259 00260
Generated on Sun Jul 17 2022 23:40:11 by
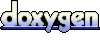